编写一个函数,由实参传来一个字符串,统计此字符串中字母、数字、空格和其他字符的个数,在主函数中输入字符串以及输出上述的结果。C语言
时间: 2023-11-17 14:12:07 浏览: 53
以下是一个简单的 C 语言程序,实现了统计字符串中字母、数字、空格和其他字符的个数的功能:
```c
#include <stdio.h>
#include <ctype.h>
void count_chars(char *str, int *letters, int *digits, int *spaces, int *others)
{
int i;
char ch;
*letters = *digits = *spaces = *others = 0;
for (i = 0; str[i] != '\0'; i++) {
ch = str[i];
if (isalpha(ch)) {
(*letters)++;
} else if (isdigit(ch)) {
(*digits)++;
} else if (isspace(ch)) {
(*spaces)++;
} else {
(*others)++;
}
}
}
int main()
{
char str[100];
int letters, digits, spaces, others;
printf("Enter a string: ");
fgets(str, sizeof(str), stdin);
count_chars(str, &letters, &digits, &spaces, &others);
printf("Number of letters: %d\n", letters);
printf("Number of digits: %d\n", digits);
printf("Number of spaces: %d\n", spaces);
printf("Number of other characters: %d\n", others);
return 0;
}
```
在上面的代码中,`count_chars` 函数接收一个字符串指针和四个整型指针作为参数,分别代表字母、数字、空格和其他字符的计数器。函数内部遍历字符串中的每个字符,使用 `isalpha`、`isdigit` 和 `isspace` 函数判断字符的类型,并根据不同的类型增加相应的计数器。最后,在主函数中调用 `count_chars` 函数,将计数器的值作为参数传递给它,并输出结果。
以上代码是一种简单的实现方式,可以根据实际需求进行修改和优化。
相关推荐
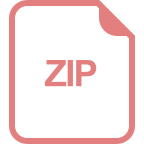
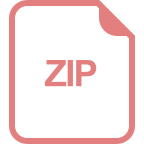















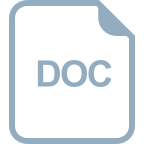