des 加密python实现
时间: 2023-09-01 22:07:14 浏览: 111
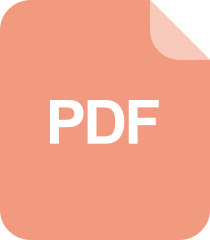
python如何实现DES加密
以下是使用 Python 中的 PyCryptodome 库实现 DES 加密的示例代码:
```python
from Crypto.Cipher import DES
# 设置密钥,必须为8字节
key = b'abcdefgh'
# 创建 DES 对象
des = DES.new(key, DES.MODE_ECB)
# 需要加密的明文
plaintext = b'this is a plaintext'
# 加密明文
ciphertext = des.encrypt(plaintext)
# 输出加密结果
print(ciphertext)
```
需要注意的是,DES 加密算法已经被认为是不安全的,建议使用 AES 等更安全的加密算法。另外,密钥的长度必须为8字节。
阅读全文
相关推荐
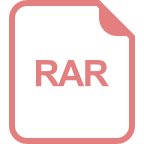
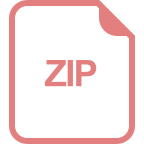




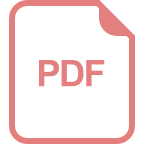
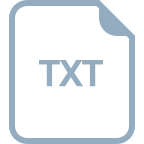
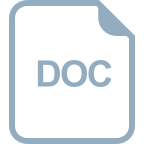





