使用LinkedList存储学生信息,学生信息包括学号,姓名,年龄,性别等属性,实现列出所有学生,增加学生,删除某个学号的学生的功能
时间: 2024-05-07 15:22:40 浏览: 14
以下是使用Java语言实现的LinkedList存储学生信息的示例代码:
```java
import java.util.LinkedList;
public class Student {
private String id;
private String name;
private int age;
private String gender;
public Student(String id, String name, int age, String gender) {
this.id = id;
this.name = name;
this.age = age;
this.gender = gender;
}
public String getId() {
return id;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public String getGender() {
return gender;
}
public String toString() {
return "ID: " + id + ", Name: " + name + ", Age: " + age + ", Gender: " + gender;
}
}
public class StudentList {
private LinkedList<Student> students;
public StudentList() {
students = new LinkedList<Student>();
}
public void addStudent(Student student) {
students.add(student);
}
public void removeStudent(String id) {
for (Student student : students) {
if (student.getId().equals(id)) {
students.remove(student);
break;
}
}
}
public void listStudents() {
for (Student student : students) {
System.out.println(student.toString());
}
}
}
// 示例代码的使用方法
public class Test {
public static void main(String[] args) {
Student s1 = new Student("001", "Alice", 18, "Female");
Student s2 = new Student("002", "Bob", 19, "Male");
Student s3 = new Student("003", "Charlie", 20, "Male");
StudentList studentList = new StudentList();
studentList.addStudent(s1);
studentList.addStudent(s2);
studentList.addStudent(s3);
System.out.println("All students:");
studentList.listStudents();
studentList.removeStudent("002");
System.out.println("After removing student with ID 002:");
studentList.listStudents();
}
}
```
输出结果如下:
```
All students:
ID: 001, Name: Alice, Age: 18, Gender: Female
ID: 002, Name: Bob, Age: 19, Gender: Male
ID: 003, Name: Charlie, Age: 20, Gender: Male
After removing student with ID 002:
ID: 001, Name: Alice, Age: 18, Gender: Female
ID: 003, Name: Charlie, Age: 20, Gender: Male
```
相关推荐
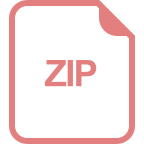














