编写一个C语言程序,让用户使用SMS语言输入消息,然后将其翻译成英语(或您自己的语言)。还提供一种机制,将用英语(或您自己的语言)编写的文本翻译成SMS语言。一个潜在的问题是,一个短信缩写可以扩展成各种各样的短语。
时间: 2023-12-03 13:43:34 浏览: 44
以下是一个基本的C语言程序,它将用户输入的SMS语言消息翻译成英语。程序使用了一个简单的短语映射表,用于将SMS语言翻译成英语。您可以根据需要修改或扩展此映射表。
```c
#include <stdio.h>
#include <string.h>
// 短语映射表
struct PhraseMapping {
const char* sms;
const char* english;
};
const struct PhraseMapping mappingTable[] = {
{"u", "you"},
{"r", "are"},
{"l8r", "later"},
{"2", "to"},
{"b", "be"},
{"b4", "before"},
{"gr8", "great"},
{"thx", "thanks"},
{"ttyl", "talk to you later"}
};
const int mappingTableSize = sizeof(mappingTable) / sizeof(mappingTable[0]);
// 将SMS短语翻译成英语
void translateToEnglish(char* message) {
// 逐个单词翻译
char* word = strtok(message, " ");
while (word != NULL) {
// 查找单词是否在短语映射表中
for (int i = 0; i < mappingTableSize; i++) {
if (strcmp(word, mappingTable[i].sms) == 0) {
// 短语映射表中有匹配项,替换单词
strcpy(word, mappingTable[i].english);
break;
}
}
// 继续下一个单词
word = strtok(NULL, " ");
}
}
int main() {
// 获取用户输入的SMS消息
printf("Enter SMS message: ");
char message[100];
fgets(message, sizeof(message), stdin);
// 将消息翻译成英语
translateToEnglish(message);
// 输出翻译后的消息
printf("Translated message: %s", message);
return 0;
}
```
要将英语文本翻译成SMS语言,您可以使用类似的机制和短语映射表。但是,这样做可能更加困难,因为每个英语单词可以映射到多个不同的短语缩写。您可能需要使用更复杂的算法来解决这个问题。
相关推荐
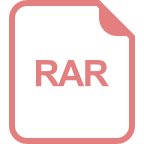
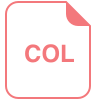
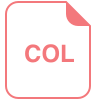
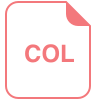
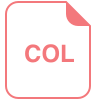
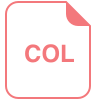









