python五子棋功能
时间: 2023-10-08 11:07:59 浏览: 49
五子棋是一种非常有趣的棋类游戏,以下是一个简单的 Python 五子棋实现:
1. 导入必要的模块:
```python
import numpy as np
import pygame
```
2. 定义五子棋游戏类:
```python
class Gomoku:
def __init__(self, ROW, COL, LENGTH, WIDTH, BLACK, WHITE, WINNING_LENGTH):
self.ROW = ROW
self.COL = COL
self.LENGTH = LENGTH
self.WIDTH = WIDTH
self.BLACK = BLACK
self.WHITE = WHITE
self.WINNING_LENGTH = WINNING_LENGTH
self.board = np.zeros((ROW, COL))
self.player = 1
self.game_over = False
```
3. 定义绘制棋盘和棋子的方法:
```python
def draw_board(self, screen):
for r in range(self.ROW):
for c in range(self.COL):
pygame.draw.rect(screen, (0, 0, 0), (c * self.LENGTH, r * self.WIDTH, self.LENGTH, self.WIDTH), 2)
if self.board[r][c] == 1:
pygame.draw.circle(screen, self.BLACK, (int(c * self.LENGTH + self.LENGTH / 2), int(r * self.WIDTH + self.WIDTH / 2)), int(self.LENGTH / 2 - 5))
elif self.board[r][c] == 2:
pygame.draw.circle(screen, self.WHITE, (int(c * self.LENGTH + self.LENGTH / 2), int(r * self.WIDTH + self.WIDTH / 2)), int(self.LENGTH / 2 - 5))
def draw_piece(self, screen, x, y):
if self.player == 1:
pygame.draw.circle(screen, self.BLACK, (x, y), int(self.LENGTH / 2 - 5))
elif self.player == 2:
pygame.draw.circle(screen, self.WHITE, (x, y), int(self.LENGTH / 2 - 5))
```
4. 定义下棋和判断胜负的方法:
```python
def drop_piece(self, row, col):
if self.board[row][col] == 0:
self.board[row][col] = self.player
self.player = 3 - self.player
def is_winning_move(self, row, col):
# 横向判断
count = 0
for i in range(max(0, col - self.WINNING_LENGTH + 1), min(self.COL, col + self.WINNING_LENGTH)):
if self.board[row][i] == self.board[row][col]:
count += 1
else:
count = 0
if count == self.WINNING_LENGTH:
return True
# 纵向判断
count = 0
for i in range(max(0, row - self.WINNING_LENGTH + 1), min(self.ROW, row + self.WINNING_LENGTH)):
if self.board[i][col] == self.board[row][col]:
count += 1
else:
count = 0
if count == self.WINNING_LENGTH:
return True
# 斜向判断
count = 0
for i in range(max(0, row - self.WINNING_LENGTH + 1), min(self.ROW, row + self.WINNING_LENGTH)):
for j in range(max(0, col - self.WINNING_LENGTH + 1), min(self.COL, col + self.WINNING_LENGTH)):
if i - row == j - col:
if self.board[i][j] == self.board[row][col]:
count += 1
else:
count = 0
if count == self.WINNING_LENGTH:
return True
# 反斜向判断
count = 0
for i in range(max(0, row - self.WINNING_LENGTH + 1), min(self.ROW, row + self.WINNING_LENGTH)):
for j in range(max(0, col - self.WINNING_LENGTH + 1), min(self.COL, col + self.WINNING_LENGTH)):
if row - i == j - col:
if self.board[i][j] == self.board[row][col]:
count += 1
else:
count = 0
if count == self.WINNING_LENGTH:
return True
return False
```
5. 定义游戏主循环:
```python
def main():
ROW = 15
COL = 15
LENGTH = 40
WIDTH = 40
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
WINNING_LENGTH = 5
SCREEN_WIDTH = COL * LENGTH
SCREEN_HEIGHT = ROW * WIDTH
pygame.init()
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption("Five in a Row")
game = Gomoku(ROW, COL, LENGTH, WIDTH, BLACK, WHITE, WINNING_LENGTH)
while not game.game_over:
for event in pygame.event.get():
if event.type == pygame.QUIT:
game.game_over = True
if event.type == pygame.MOUSEBUTTONDOWN:
x, y = event.pos
col = x // LENGTH
row = y // WIDTH
game.drop_piece(row, col)
if game.is_winning_move(row, col):
game.game_over = True
game.draw_board(screen)
pygame.display.update()
pygame.quit()
if __name__ == "__main__":
main()
```
这样,你就可以在 Pygame 中玩五子棋游戏了!
相关推荐
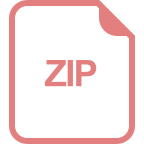
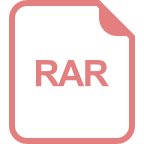
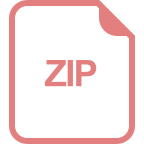












