用html css js写一个超级马里奥游戏
时间: 2023-11-23 15:06:22 浏览: 136
抱歉,用HTML、CSS、JS写一个完整的超级马里奥游戏是非常困难的,需要大量的时间和技术。但是,我们可以使用HTML、CSS、JS来制作一个简单的马里奥游戏的原型。以下是一个简单的示例代码:
HTML:
```
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>超级马里奥游戏</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div id="game-board">
<div id="mario"></div>
<div id="coin"></div>
</div>
<script src="script.js"></script>
</body>
</html>
```
CSS:
```
#game-board {
width: 600px;
height: 400px;
border: 1px solid black;
position: relative;
}
#mario {
width: 50px;
height: 50px;
background-image: url('mario.png');
background-size: cover;
position: absolute;
left: 0;
bottom: 0;
}
#coin {
width: 20px;
height: 20px;
background-image: url('coin.png');
background-size: cover;
position: absolute;
top: 0;
right: 0;
}
```
JS:
```
var mario = document.getElementById('mario');
var coin = document.getElementById('coin');
document.addEventListener('keydown', function(event) {
if (event.code == 'ArrowRight') {
mario.style.left = parseInt(mario.style.left) + 10 + 'px';
} else if (event.code == 'ArrowLeft') {
mario.style.left = parseInt(mario.style.left) - 10 + 'px';
} else if (event.code == 'ArrowUp') {
mario.style.bottom = parseInt(mario.style.bottom) + 50 + 'px';
}
if (isCollided(mario, coin)) {
alert('你获得了金币!');
coin.style.display = 'none';
}
});
function isCollided(element1, element2) {
var rect1 = element1.getBoundingClientRect();
var rect2 = element2.getBoundingClientRect();
return !(
rect1.bottom < rect2.top ||
rect1.top > rect2.bottom ||
rect1.right < rect2.left ||
rect1.left > rect2.right
);
}
```
上述代码中,我们使用HTML来创建游戏场景,使用CSS来设置游戏元素的样式,使用JS来实现角色移动和碰撞检测。当玩家按下方向键时,角色会相应地移动。当角色与金币发生碰撞时,会触发获得金币的事件。
需要注意的是,这只是一个非常简单的马里奥游戏原型,仅供参考。如果你想制作一个更完整、更复杂的游戏,需要更多的技术和时间。
阅读全文
相关推荐

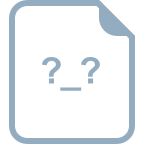
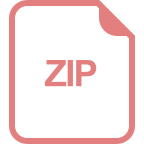

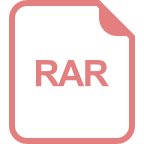
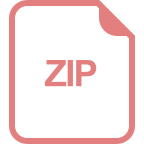
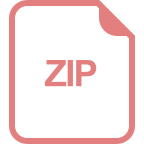
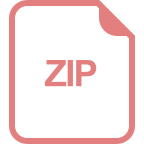
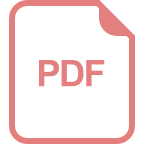
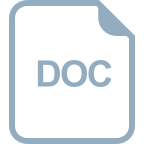
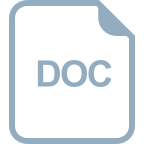
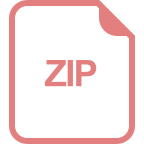
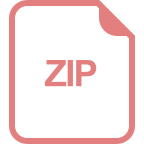
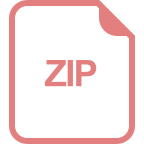
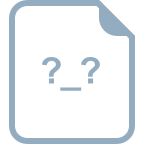