帮我写一个基于Python的数字识别系统
时间: 2024-04-30 22:19:52 浏览: 109
好的,数字识别系统可以使用机器学习算法来实现。以下是一个基于Python的数字识别系统的简单实现:
1. 数据集准备
首先,我们需要一个数据集来训练我们的模型。MNIST 数据集是一个常用的手写数字数据集,可以在 Python 中使用 TensorFlow 框架来获取和处理。我们可以使用以下代码从 TensorFlow 中加载 MNIST 数据集:
```python
import tensorflow as tf
# Load MNIST dataset
(x_train, y_train), (x_test, y_test) = tf.keras.datasets.mnist.load_data()
# Normalize the images
x_train = x_train / 255.0
x_test = x_test / 255.0
# Reshape the images to 28x28 pixels
x_train = x_train.reshape((-1, 28, 28, 1))
x_test = x_test.reshape((-1, 28, 28, 1))
```
2. 模型构建
接下来,我们可以使用 TensorFlow 构建一个卷积神经网络模型。以下是一个简单的模型:
```python
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Conv2D(64, (3, 3), activation='relu'),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Conv2D(64, (3, 3), activation='relu'),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
```
这个模型包含了 3 层卷积神经网络和 2 层全连接层。
3. 模型训练
现在,我们可以使用训练数据集来训练模型。以下是一个简单的训练代码:
```python
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
model.fit(x_train, y_train, epochs=5, validation_data=(x_test, y_test))
```
在这个代码中,我们使用 Adam 优化器和交叉熵损失函数来训练模型。
4. 模型评估
最后,我们可以使用测试数据集来评估模型的性能。以下是一个简单的评估代码:
```python
test_loss, test_acc = model.evaluate(x_test, y_test)
print('Test accuracy:', test_acc)
```
这个代码将输出测试数据集上的模型准确率。
这就是一个基于 Python 的数字识别系统的简单实现。当然,你可以对模型进行更多的调整和优化来提高识别准确率。
阅读全文
相关推荐
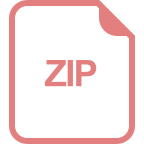
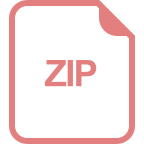
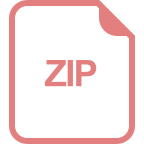















