帮我写一个复杂的html网页音乐播放器代码
时间: 2023-08-28 22:17:23 浏览: 83
以下是一个简单的HTML音乐播放器代码示例:
```html
<!DOCTYPE html>
<html>
<head>
<title>音乐播放器</title>
<style>
/* 播放器样式 */
#player {
display: flex;
flex-direction: column;
align-items: center;
background-color: #f2f2f2;
padding: 20px;
border-radius: 10px;
box-shadow: 0px 0px 10px rgba(0, 0, 0, 0.3);
width: 400px;
}
#controls {
display: flex;
align-items: center;
margin-top: 20px;
}
#controls button {
background-color: #4CAF50;
border: none;
color: white;
padding: 10px;
text-align: center;
text-decoration: none;
display: inline-block;
font-size: 16px;
margin: 4px 2px;
cursor: pointer;
border-radius: 5px;
}
#controls button:hover {
background-color: #3e8e41;
}
#song-list {
margin-top: 20px;
display: flex;
flex-direction: column;
align-items: center;
}
#song-list li {
list-style-type: none;
margin: 10px;
cursor: pointer;
}
#song-list li:hover {
color: #4CAF50;
}
</style>
</head>
<body>
<div id="player">
<h1>音乐播放器</h1>
<audio id="audio-player" src=""></audio>
<div id="controls">
<button id="play-button">播放</button>
<button id="pause-button">暂停</button>
<button id="stop-button">停止</button>
<button id="prev-button">上一首</button>
<button id="next-button">下一首</button>
</div>
<ul id="song-list">
<li data-src="path/to/song1.mp3">歌曲1</li>
<li data-src="path/to/song2.mp3">歌曲2</li>
<li data-src="path/to/song3.mp3">歌曲3</li>
<li data-src="path/to/song4.mp3">歌曲4</li>
</ul>
</div>
<script>
// 获取播放器和歌曲列表
const audioPlayer = document.querySelector('#audio-player');
const songList = document.querySelectorAll('#song-list li');
let currentSongIndex = 0;
// 初始化播放器
function initPlayer() {
audioPlayer.addEventListener('ended', playNextSong);
document.querySelector('#play-button').addEventListener('click', playSong);
document.querySelector('#pause-button').addEventListener('click', pauseSong);
document.querySelector('#stop-button').addEventListener('click', stopSong);
document.querySelector('#prev-button').addEventListener('click', playPrevSong);
document.querySelector('#next-button').addEventListener('click', playNextSong);
// 初始化第一首歌曲
updateSong(currentSongIndex);
}
// 播放歌曲
function playSong() {
audioPlayer.play();
}
// 暂停歌曲
function pauseSong() {
audioPlayer.pause();
}
// 停止歌曲
function stopSong() {
audioPlayer.pause();
audioPlayer.currentTime = 0;
}
// 播放下一首歌曲
function playNextSong() {
if (currentSongIndex < songList.length - 1) {
currentSongIndex++;
} else {
currentSongIndex = 0;
}
updateSong(currentSongIndex);
}
// 播放上一首歌曲
function playPrevSong() {
if (currentSongIndex > 0) {
currentSongIndex--;
} else {
currentSongIndex = songList.length - 1;
}
updateSong(currentSongIndex);
}
// 更新歌曲
function updateSong(index) {
audioPlayer.src = songList[index].getAttribute('data-src');
audioPlayer.load();
audioPlayer.play();
}
// 初始化播放器
initPlayer();
</script>
</body>
</html>
```
这个代码使用了HTML的基本元素和CSS样式,包括标题(`<title>`),播放器(`<div>`),音频播放器(`<audio>`),控制按钮(`<button>`),歌曲列表(`<ul>`)和歌曲列表项(`<li>`)等。你可以根据自己的需求修改播放器的样式和歌曲列表内容。
阅读全文
相关推荐
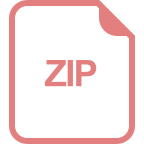
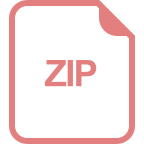
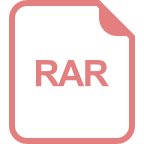















