使用 Python 3 和 tkinter 写一个excle表处理程序,GUI界面为:3个文本框3个按钮,按钮名称分别叫导入表格、绩效总表、处理表格。 表格处理过程为:点击导入表格导入多个需要处理的表格文件显示在第一个文本框中。点击绩效总表按钮导入表格文件显示到第二个文本框中,点击处理表格按钮,将每一个导入的表格的第一给工作表的名称改为表格文件名,然后复制导入表格中每一个表格的第一个工作表(包括格式)粘贴到绩效总表中。
时间: 2024-02-12 21:06:07 浏览: 78
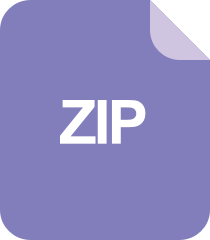
Python实现处理excel表格,主要以多表汇总为主.zip
好的,可以给你一个基本的代码框架,你可以根据需要进行修改和完善:
```
import tkinter as tk
from tkinter import filedialog
import os
import openpyxl
class ExcelProcessor:
def __init__(self):
self.root = tk.Tk()
self.root.title("Excel表处理程序")
self.root.geometry("500x200")
self.import_files = []
self.output_file = ""
self.label1 = tk.Label(self.root, text="导入表格:")
self.label1.grid(row=0, column=0)
self.entry1 = tk.Entry(self.root, width=30)
self.entry1.grid(row=0, column=1)
self.button1 = tk.Button(self.root, text="导入表格", command=self.import_excel)
self.button1.grid(row=0, column=2)
self.label2 = tk.Label(self.root, text="绩效总表:")
self.label2.grid(row=1, column=0)
self.entry2 = tk.Entry(self.root, width=30)
self.entry2.grid(row=1, column=1)
self.button2 = tk.Button(self.root, text="导入绩效总表", command=self.import_output_file)
self.button2.grid(row=1, column=2)
self.button3 = tk.Button(self.root, text="处理表格", command=self.process_excel)
self.button3.grid(row=2, column=1)
self.root.mainloop()
def import_excel(self):
filetypes = (("Excel files", "*.xlsx"), ("All files", "*.*"))
files = filedialog.askopenfilenames(filetypes=filetypes)
self.import_files = list(files)
self.entry1.delete(0, tk.END)
self.entry1.insert(0, "\n".join(self.import_files))
def import_output_file(self):
filetypes = (("Excel files", "*.xlsx"), ("All files", "*.*"))
file_path = filedialog.askopenfilename(filetypes=filetypes)
self.output_file = file_path
self.entry2.delete(0, tk.END)
self.entry2.insert(0, self.output_file)
def process_excel(self):
if not self.import_files:
tk.messagebox.showerror("错误", "请导入需要处理的表格文件!")
return
if not self.output_file:
tk.messagebox.showerror("错误", "请导入绩效总表文件!")
return
output_wb = openpyxl.load_workbook(self.output_file)
for file_path in self.import_files:
file_name = os.path.basename(file_path)
wb = openpyxl.load_workbook(file_path)
sheets = wb.sheetnames
sheet1 = wb[sheets[0]]
sheet1.title = file_name
output_sheet = output_wb.create_sheet(title=file_name)
for row in sheet1.rows:
for cell in row:
output_sheet[cell.coordinate].value = cell.value
output_sheet[cell.coordinate].font = cell.font
output_sheet[cell.coordinate].fill = cell.fill
output_sheet[cell.coordinate].border = cell.border
output_sheet[cell.coordinate].alignment = cell.alignment
output_wb.save(self.output_file)
tk.messagebox.showinfo("提示", "表格处理完成!")
if __name__ == "__main__":
ExcelProcessor()
```
这个代码使用了tkinter库实现了一个简单的GUI界面,包括三个文本框和三个按钮。导入表格按钮和导入绩效总表按钮分别调用了文件对话框来选择需要导入的表格文件和绩效总表文件。处理表格按钮则调用了处理函数来进行表格处理操作。
在处理函数中,我们首先检查导入的表格文件和绩效总表文件是否为空,如果为空,则弹出错误提示框。否则,我们依次遍历导入的表格文件,将每一个表格的第一个工作表的名称改为文件名,并将其复制到绩效总表中。我们使用openpyxl库来读取和写入Excel表格,其中load_workbook函数用于读取Excel表格,create_sheet函数用于创建新的工作表,sheetnames属性用于获取所有工作表的名称,title属性用于获取或设置工作表的名称,rows属性用于获取所有行,coordinate属性用于获取单元格的坐标,value、font、fill、border、alignment属性用于获取或设置单元格的值、字体、填充、边框和对齐方式。
最后,我们使用messagebox库来弹出提示框,告知用户表格处理完成。
阅读全文
相关推荐





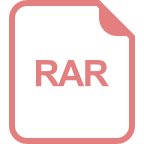
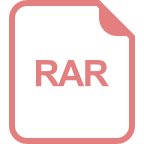
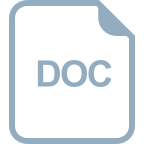
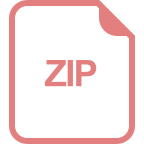
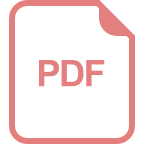
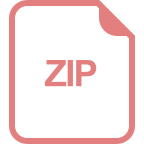
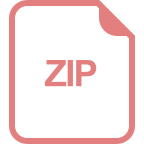
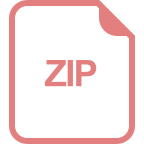
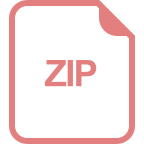
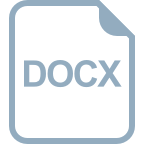