c# 基于二维数组读取16位tiff格式的灰度图像的像素值
时间: 2023-06-18 17:06:30 浏览: 162
可以使用System.Drawing命名空间中的Bitmap类来读取16位Tiff格式的灰度图像像素值。下面是一个示例代码:
```csharp
using System.Drawing;
using System.Drawing.Imaging;
// ...
// 读取16位Tiff格式的灰度图像
Bitmap bitmap = new Bitmap("image.tif");
// 获取图像的宽度和高度
int width = bitmap.Width;
int height = bitmap.Height;
// 获取图像的像素格式
PixelFormat pixelFormat = bitmap.PixelFormat;
// 判断像素格式是否为16位灰度图像
if (pixelFormat != PixelFormat.Format16bppGrayScale)
{
throw new Exception("不是16位灰度图像");
}
// 锁定位图数据,获取像素值
BitmapData bitmapData = bitmap.LockBits(new Rectangle(0, 0, width, height),
ImageLockMode.ReadOnly, PixelFormat.Format16bppGrayScale);
// 获取像素值的字节数
int stride = bitmapData.Stride;
int bytesPerPixel = stride / width;
// 定义一个二维数组存储像素值
ushort[,] pixels = new ushort[height, width];
// 逐行读取像素值
unsafe
{
byte* p = (byte*)bitmapData.Scan0;
for (int y = 0; y < height; y++)
{
for (int x = 0; x < width; x++)
{
// 获取当前像素的指针
ushort* pixelPtr = (ushort*)(p + y * stride + x * bytesPerPixel);
// 获取像素值
ushort pixelValue = *pixelPtr;
// 存储像素值
pixels[y, x] = pixelValue;
}
}
}
// 解锁位图数据
bitmap.UnlockBits(bitmapData);
```
上述代码使用LockBits方法锁定位图数据,获取像素值之后再使用UnlockBits方法解锁位图数据。在获取像素值时,使用了指针操作来提高效率。注意,由于C#中的多维数组是按行存储的,因此在存储像素值时,需要按照[y, x]的顺序存储。
相关推荐
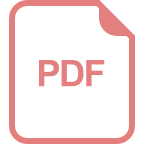
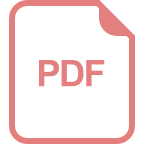














