python代码
时间: 2023-07-02 22:24:07 浏览: 114
好的,以下是一个基于Python深度学习框架Keras的植物识别代码示例,供您参考:
```python
# 导入必要的库
import numpy as np
import tensorflow as tf
from keras.preprocessing import image
from keras.models import Sequential
from keras.layers import Conv2D, MaxPooling2D
from keras.layers import Activation, Dropout, Flatten, Dense
from keras import backend as K
# 设置图像大小和通道数
img_width, img_height = 150, 150
if K.image_data_format() == 'channels_first':
input_shape = (3, img_width, img_height)
else:
input_shape = (img_width, img_height, 3)
# 创建模型
model = Sequential()
model.add(Conv2D(32, (3, 3), input_shape=input_shape))
model.add(Activation('relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Conv2D(32, (3, 3)))
model.add(Activation('relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Conv2D(64, (3, 3)))
model.add(Activation('relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Flatten())
model.add(Dense(64))
model.add(Activation('relu'))
model.add(Dropout(0.5))
model.add(Dense(8))
model.add(Activation('softmax'))
# 编译模型
model.compile(loss='categorical_crossentropy',
optimizer='rmsprop',
metrics=['accuracy'])
# 加载训练好的权重
model.load_weights('plant_model.h5')
# 加载图像并进行预测
img_path = 'test_image.jpg'
img = image.load_img(img_path, target_size=(img_width, img_height))
x = image.img_to_array(img)
x = np.expand_dims(x, axis=0)
preds = model.predict_classes(x)
# 打印预测结果
print(preds)
```
这段代码使用了一个基于卷积神经网络的模型,先对图像进行卷积操作和池化操作,然后通过全连接层和softmax函数进行分类,并使用交叉熵作为损失函数进行优化。模型训练完成后,使用load_weights方法加载训练好的权重,并使用img_to_array方法将图像转换为张量进行预测,最后输出预测结果。
阅读全文
相关推荐
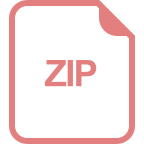
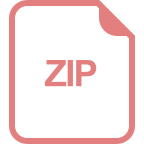
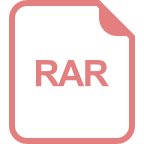
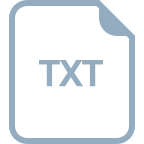