stm32F103C8T6串口3通讯
时间: 2023-09-05 18:06:56 浏览: 96
下面是使用STM32F103C8T6的串口3进行通讯的基本步骤:
1. 配置GPIO引脚
首先,需要配置GPIO引脚作为串口3的TX和RX引脚。在STM32F1系列中,USART3的TX引脚为PB10,RX引脚为PB11。
```c
// Enable GPIOB clock
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
// Configure USART3 Tx (PB10) as alternate function push-pull
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStructure);
// Configure USART3 Rx (PB11) as input floating
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_11;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOB, &GPIO_InitStructure);
```
2. 配置USART
接下来,需要配置USART3的参数,例如波特率、数据位、停止位、校验位等。在这里,我们选择9600波特率,8位数据位,1位停止位,无校验位。
```c
// Enable USART3 clock
RCC_APB1PeriphClockCmd(RCC_APB1Periph_USART3, ENABLE);
// Configure USART3
USART_InitTypeDef USART_InitStructure;
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART3, &USART_InitStructure);
// Enable USART3
USART_Cmd(USART3, ENABLE);
```
3. 发送数据
完成了上述配置后,就可以使用USART3发送数据了。以下是一个简单的示例:
```c
// Send a string via USART3
char* message = "Hello, world!\r\n";
for (int i = 0; i < strlen(message); i++) {
while (USART_GetFlagStatus(USART3, USART_FLAG_TXE) == RESET); // Wait for the USART3 Tx buffer to empty
USART_SendData(USART3, message[i]); // Send the next character
}
```
4. 接收数据
如果要从USART3接收数据,可以使用以下代码:
```c
// Receive a character via USART3
while (USART_GetFlagStatus(USART3, USART_FLAG_RXNE) == RESET); // Wait for the USART3 Rx buffer to fill
char received_char = USART_ReceiveData(USART3); // Read the received character
```
以上就是使用STM32F103C8T6的串口3进行通讯的基本步骤。注意,以上代码仅为示例,具体实现可能需要根据具体需求进行修改。
阅读全文
相关推荐
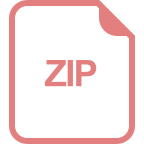
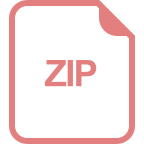
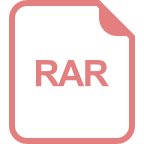
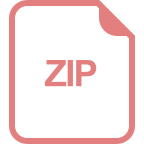
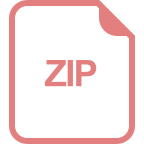
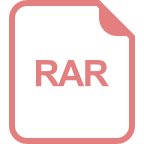
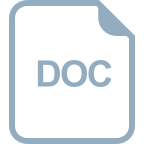







