import pandas as pd import tkinter as tk from tkinter import filedialog from sklearn.preprocessing import StandardScaler # 定义全局变量 file_path = "" def import_csv_data(): global file_path file_path = filedialog.askopenfilename() # 读取CSV文件并显示在Text控件上 data = pd.read_csv(file_path) # 获取前5行数据 top_5 = data.head() # 将前5行数据插入到Text控件 txt_data.insert(tk.END, top_5) # 处理缺失值 def handle_missing_values(): global file_path # 修改2:使用全局变量 # 读取CSV文件 data = pd.read_csv(file_path) # 处理缺失值 data.fillna(0, inplace=True) # 显示前10行数据 text_output.insert(tk.END, "处理缺失值成功,前10行数据如下:\n") text_output.insert(tk.END, str(data.head(10))) # 标准化数值型数据 def normalize_numeric_data(): global file_path # 读取CSV文件 data = pd.read_csv(file_path) # 提取数值型数据 numeric_data = data.select_dtypes(include=['float64', 'int64']) # 标准化数据 scaler = StandardScaler() normalized_data = scaler.fit_transform(numeric_data) # 将处理后的数据写回原数据框 data.loc[:, numeric_data.columns] = normalized_data # 显示前10行数据 text_output.insert(tk.END, "标准化数值型数据成功,前10行数据如下:\n") text_output.insert(tk.END, str(data.head(10))) 这段代码后的def encode_categorical_data(): # 读取CSV文件 data = pd.read_csv("file.csv") # 提取类别型数据 categorical_data = data.select_dtypes(include=['object']) # 编码数据 encoder = LabelEncoder() encoded_data = categorical_data.apply(encoder.fit_transform) # 将处理后的数据写回原数据框 data.loc[:, categorical_data.columns] = encoded_data # 显示前10行数据 text_output.insert(tk.END, "编码类别型数据成功,前10行数据如下:\n") text_output.insert(tk.END, str(data.head(10)))这段代码怎么改能跑通
时间: 2024-01-21 22:04:39 浏览: 33
你需要将该函数中读取的文件路径改为全局变量file_path所指向的文件路径,即将"data = pd.read_csv("file.csv")"改为"data = pd.read_csv(file_path)",这样就可以根据用户选择的文件进行处理了。同时,在函数开头加上"global file_path",以便函数可以访问全局变量file_path。修改后的代码如下:
```
def encode_categorical_data():
global file_path
# 读取CSV文件
data = pd.read_csv(file_path)
# 提取类别型数据
categorical_data = data.select_dtypes(include=['object'])
# 编码数据
encoder = LabelEncoder()
encoded_data = categorical_data.apply(encoder.fit_transform)
# 将处理后的数据写回原数据框
data.loc[:, categorical_data.columns] = encoded_data
# 显示前10行数据
text_output.insert(tk.END, "编码类别型数据成功,前10行数据如下:\n")
text_output.insert(tk.END, str(data.head(10)))
```
相关问题
import pandas as pd import csv import matplotlib.pyplot as plt import tkinter as tk from PIL import Image, ImageTk from tkinter import filedialog from matplotlib.backends.backend_tkagg import FigureCanvasTkAgg from tkinter import Tk, Button, Text, filedialog, messagebox from sklearn.preprocessing import StandardScaler,LabelEncoder from sklearn.model_selection import train_test_split from sklearn.linear_model import LinearRegression from sklearn.tree import DecisionTreeRegressor from sklearn.ensemble import RandomForestRegressor解释每一句代码的含义
这段代码是导入Python中的一些常用数据处理和机器学习库,并且实现了一些具体的功能。
- `import pandas as pd`: 导入pandas库并使用pd作为别名,pandas是一个数据处理库,可以对表格数据进行读取、清洗、转换等操作。
- `import csv`: 导入csv库,csv是一种常用的表格数据格式,可以用于读取和写入csv文件。
- `import matplotlib.pyplot as plt`: 导入matplotlib库,并使用plt作为别名,matplotlib是一个绘图库,可以用于绘制各种图形、图表等。
- `import tkinter as tk`: 导入tkinter库,并使用tk作为别名,tkinter是Python的标准GUI库,可以用于创建各种用户界面。
- `from PIL import Image, ImageTk`: 导入PIL库中的Image和ImageTk模块,PIL是一个图像处理库,可以用于读取、处理和保存各种图像格式。
- `from tkinter import filedialog`: 从tkinter库中导入filedialog模块,filedialog是一个用于打开和保存文件对话框的模块。
- `from matplotlib.backends.backend_tkagg import FigureCanvasTkAgg`: 从matplotlib库中导入FigureCanvasTkAgg模块,可以用于在Tkinter界面中绘制matplotlib图形。
- `from tkinter import Tk, Button, Text, filedialog, messagebox`: 从tkinter库中导入Tk、Button、Text、filedialog和messagebox等模块,用于创建各种界面控件和显示消息框。
- `from sklearn.preprocessing import StandardScaler,LabelEncoder`: 从sklearn库中导入StandardScaler和LabelEncoder模块,用于数据预处理,如特征缩放和标签编码等。
- `from sklearn.model_selection import train_test_split`: 从sklearn库中导入train_test_split模块,用于将数据集划分为训练集和测试集。
- `from sklearn.linear_model import LinearRegression`: 从sklearn库中导入LinearRegression模块,用于线性回归分析。
- `from sklearn.tree import DecisionTreeRegressor`: 从sklearn库中导入DecisionTreeRegressor模块,用于决策树回归分析。
- `from sklearn.ensemble import RandomForestRegressor`: 从sklearn库中导入RandomForestRegressor模块,用于随机森林回归分析。
如何在import pandas as pd import tkinter as tk from tkinter import filedialog from sklearn.preprocessing import StandardScaler,LabelEncoder # 定义全局变量 file_path = "" # 导入数据集 def import_csv_data(): global file_path file_path = filedialog.askopenfilename() # 读取CSV文件并显示在Text控件上 data = pd.read_csv(file_path) # 获取前5行数据 top_5 = data.head() # 将前5行数据插入到Text控件 txt_data.insert(tk.END, top_5) # 处理缺失值 def handle_missing_values(): global file_path # 读取CSV文件 data = pd.read_csv(file_path) # 处理缺失值 data.fillna(0, inplace=True) # 显示前10行数据 text_output.insert(tk.END, "处理缺失值成功,前10行数据如下:\n") text_output.insert(tk.END, str(data.head(10))) # 标准化数值型数据 def normalize_numeric_data(): global file_path # 读取CSV文件 data = pd.read_csv(file_path) # 提取数值型数据 numeric_data = data.select_dtypes(include=['float64', 'int64']) # 标准化数据 scaler = StandardScaler() normalized_data = scaler.fit_transform(numeric_data) # 将处理后的数据写回原数据框 data.loc[:, numeric_data.columns] = normalized_data # 显示前10行数据 text_output.insert(tk.END, "标准化数值型数据成功,前10行数据如下:\n") text_output.insert(tk.END, str(data.head(10)))这段代码后学些一段代码实现设置填空按钮,可设置训练集测试集比例,按后完成指定划分的功能
下面是一个示例代码,实现了设置填空按钮,可设置训练集测试集比例,按后完成指定划分的功能:
```
import pandas as pd
import tkinter as tk
from tkinter import filedialog
from sklearn.preprocessing import StandardScaler, LabelEncoder
from sklearn.model_selection import train_test_split
# 定义全局变量
file_path = ""
train_ratio = 0.8
# 导入数据集
def import_csv_data():
global file_path
file_path = filedialog.askopenfilename()
# 读取CSV文件并显示在Text控件上
data = pd.read_csv(file_path)
# 获取前5行数据
top_5 = data.head()
# 将前5行数据插入到Text控件
txt_data.insert(tk.END, top_5)
# 处理缺失值
def handle_missing_values():
global file_path
# 读取CSV文件
data = pd.read_csv(file_path)
# 处理缺失值
data.fillna(0, inplace=True)
# 显示前10行数据
text_output.insert(tk.END, "处理缺失值成功,前10行数据如下:\n")
text_output.insert(tk.END, str(data.head(10)))
# 标准化数值型数据
def normalize_numeric_data():
global file_path
# 读取CSV文件
data = pd.read_csv(file_path)
# 提取数值型数据
numeric_data = data.select_dtypes(include=['float64', 'int64'])
# 标准化数据
scaler = StandardScaler()
normalized_data = scaler.fit_transform(numeric_data)
# 将处理后的数据写回原数据框
data.loc[:, numeric_data.columns] = normalized_data
# 显示前10行数据
text_output.insert(tk.END, "标准化数值型数据成功,前10行数据如下:\n")
text_output.insert(tk.END, str(data.head(10)))
# 划分训练集和测试集
def split_train_test():
global file_path, train_ratio
# 读取CSV文件
data = pd.read_csv(file_path)
# 划分数据集
train, test = train_test_split(data, train_size=train_ratio)
# 显示训练集和测试集大小
text_output.insert(tk.END, "训练集大小:{}\n".format(len(train)))
text_output.insert(tk.END, "测试集大小:{}\n".format(len(test)))
# 创建主窗口
root = tk.Tk()
root.title("数据处理工具")
root.geometry("800x600")
# 创建菜单栏
menubar = tk.Menu(root)
filemenu = tk.Menu(menubar, tearoff=0)
filemenu.add_command(label="导入数据集", command=import_csv_data)
filemenu.add_command(label="处理缺失值", command=handle_missing_values)
filemenu.add_command(label="标准化数值型数据", command=normalize_numeric_data)
filemenu.add_command(label="划分训练集和测试集", command=split_train_test)
menubar.add_cascade(label="文件", menu=filemenu)
root.config(menu=menubar)
# 创建控件
txt_data = tk.Text(root, height=10, width=100)
txt_data.pack()
text_output = tk.Text(root, height=10, width=100)
text_output.pack()
train_ratio_label = tk.Label(root, text="训练集比例:")
train_ratio_label.pack()
train_ratio_entry = tk.Entry(root)
train_ratio_entry.pack()
split_button = tk.Button(root, text="划分训练集和测试集", command=split_train_test)
split_button.pack()
# 运行主循环
root.mainloop()
```
在这个示例代码中,我们添加了一个文本框和一个按钮来设置训练集测试集比例,当用户点击“划分训练集和测试集”按钮时,程序会调用`split_train_test()`函数来进行数据划分,并在文本框中显示训练集和测试集的大小。注意,在这个示例中,我们使用了`train_test_split`函数来划分数据集。该函数可以随机划分数据集,并且可以指定训练集和测试集的比例。在这个示例中,我们使用了一个全局变量`train_ratio`来保存训练集比例,默认为0.8。
相关推荐
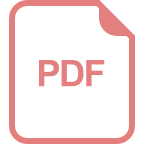
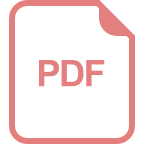








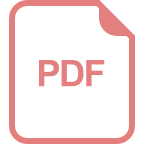
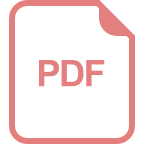
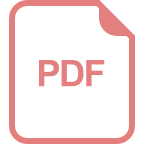