使用c语言写满足下列条件的代码1、某公司的雇员分为以下若干类: 抽象类Employee:这是所有员工总的父类; 数据成员:员工的姓名,员工的生日月份。 成员函效:string getname (),获取员工姓名;float getSalary (int month) 根据参数月份来确定工资,如果该月员工过生日,则公司会额外奖励100元。 SalariedEmployee: Employee 的子类,拿固定工资的员工。 数据成员:月薪 HourlyEmployee: Employee 的子类,按小时拿工资的员工,每月工作超出 160小时的部分按照1.5 倍工资发放。 数据成员:每小时的工资、每月工作的小时数。 Sales Employee: Employee的子类,销售人员,工资由月销售额和提成率決 定。 数据成员:月销售额、提成率。 要求写一个程序,把若干各种类型的员工放在一个 Employee 数组 里,写一个函数,打印出某月每个员工的工资数额。
时间: 2023-06-27 18:01:59 浏览: 234
以下是满足题目要求的C语言代码:
```
#include <stdio.h>
#include <string.h>
// 定义抽象类 Employee
typedef struct Employee {
char name[50]; // 员工姓名
int birth_month; // 员工生日月份
float (*getSalary)(struct Employee *self, int month); // 获取员工工资函数指针
} Employee;
// 定义 SalariedEmployee 类
typedef struct SalariedEmployee {
Employee super; // 继承 Employee 类
float salary; // 月薪
} SalariedEmployee;
// 获取 SalariedEmployee 的工资
float getSalariedEmployeeSalary(SalariedEmployee *self, int month) {
float bonus = 0;
if (month == self->super.birth_month) { // 如果过生日
bonus = 100; // 额外奖励100元
}
return self->salary + bonus;
}
// 定义 HourlyEmployee 类
typedef struct HourlyEmployee {
Employee super; // 继承 Employee 类
float hourly_wage; // 每小时工资
float hours_worked; // 每月工作小时数
} HourlyEmployee;
// 获取 HourlyEmployee 的工资
float getHourlyEmployeeSalary(HourlyEmployee *self, int month) {
float base_salary = self->hourly_wage * self->hours_worked;
float overtime_salary = 0;
if (self->hours_worked > 160) { // 超出160小时部分按1.5倍工资发放
overtime_salary = (self->hours_worked - 160) * self->hourly_wage * 0.5;
}
return base_salary + overtime_salary;
}
// 定义 SalesEmployee 类
typedef struct SalesEmployee {
Employee super; // 继承 Employee 类
float sales; // 月销售额
float commission_rate; // 提成率
} SalesEmployee;
// 获取 SalesEmployee 的工资
float getSalesEmployeeSalary(SalesEmployee *self, int month) {
return self->sales * self->commission_rate;
}
// 打印某月每个员工的工资数额
void printEmployeeSalary(Employee **employees, int num_employees, int month) {
for (int i = 0; i < num_employees; i++) {
Employee *employee = employees[i];
printf("%s's salary for month %d is %.2f\n", employee->name, month, employee->getSalary(employee, month));
}
}
int main() {
// 创建员工数组
Employee *employees[3];
// 创建 SalariedEmployee 对象
SalariedEmployee salaried_employee;
strcpy(salaried_employee.super.name, "John");
salaried_employee.super.birth_month = 3;
salaried_employee.super.getSalary = (float (*)(Employee *, int))getSalariedEmployeeSalary;
salaried_employee.salary = 5000;
employees[0] = (Employee *)&salaried_employee;
// 创建 HourlyEmployee 对象
HourlyEmployee hourly_employee;
strcpy(hourly_employee.super.name, "Mary");
hourly_employee.super.birth_month = 5;
hourly_employee.super.getSalary = (float (*)(Employee *, int))getHourlyEmployeeSalary;
hourly_employee.hourly_wage = 50;
hourly_employee.hours_worked = 180;
employees[1] = (Employee *)&hourly_employee;
// 创建 SalesEmployee 对象
SalesEmployee sales_employee;
strcpy(sales_employee.super.name, "Tom");
sales_employee.super.birth_month = 9;
sales_employee.super.getSalary = (float (*)(Employee *, int))getSalesEmployeeSalary;
sales_employee.sales = 10000;
sales_employee.commission_rate = 0.1;
employees[2] = (Employee *)&sales_employee;
// 打印每个员工的工资
printEmployeeSalary(employees, 3, 4);
return 0;
}
```
阅读全文
相关推荐
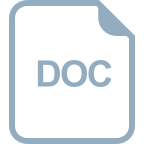
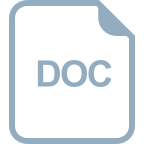
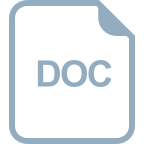
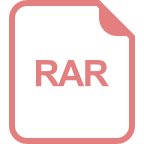
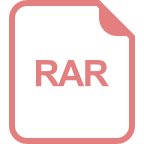
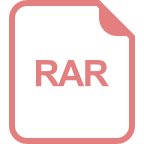
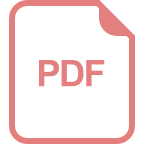
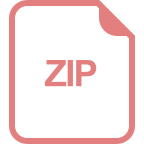
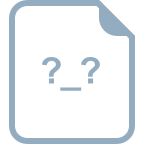
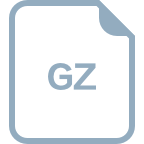
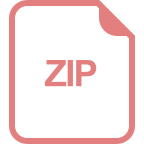
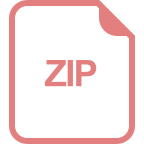
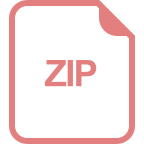
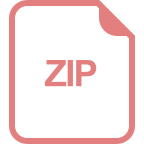
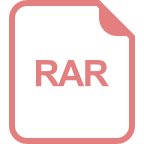
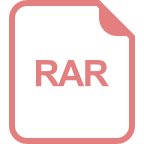
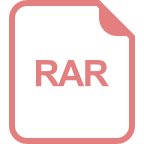
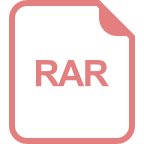