设置jtextpanel自动换行
时间: 2023-12-03 22:02:34 浏览: 150
好的,我可以帮你回答这个问题。你可以使用JTextArea而不是JTextPanel来实现自动换行,就像这样:
```
JTextArea textArea = new JTextArea();
textArea.setLineWrap(true);
textArea.setWrapStyleWord(true);
```
这样,文本就会在达到文本框的边缘时自动换行。
相关问题
java中,JTextPanel实现打字效果
你可能想问的是如何实现在JTextPane控件中打字效果。可以通过以下步骤来实现:
1. 设置JTextPane的Document类型为StyledDocument,因为StyledDocument支持富文本格式。
StyledDocument doc = new DefaultStyledDocument();
JTextPane textPane = new JTextPane(doc);
2. 创建一个定时器,每隔一定时间向StyledDocument中插入一些文本,从而实现打字效果。
Timer timer = new Timer(delay, new ActionListener() {
int pos = 0;
public void actionPerformed(ActionEvent e) {
try {
doc.insertString(doc.getLength(), text.charAt(pos) + "", null);
pos++;
if (pos >= text.length()) { // 如果文本已经全部加入,停止定时器
((Timer) e.getSource()).stop();
}
} catch (BadLocationException ex) {
ex.printStackTrace();
}
}
});
timer.start();
其中text为要打印的文本,delay为定时器的执行间隔。
注意:使用定时器时,必须在Swing的Event Dispatch Thread线程中执行。可以使用SwingUtilities.invokeLater或者SwingWorker来实现。
java中,JTextPanel实现打字效果,给一个例子
好的,以下是一个简单的 Java 程序,演示如何使用 JTextPane 实现打字效果:
```java
import javax.swing.*;
import javax.swing.text.*;
public class TypingEffectExample {
public static void main(String[] args) throws InterruptedException {
JFrame frame = new JFrame();
JTextPane textPane = new JTextPane();
frame.add(textPane);
frame.setSize(400, 300);
frame.setVisible(true);
StyledDocument doc = textPane.getStyledDocument();
Style style = doc.addStyle("typing", null);
StyleConstants.setForeground(style, textPane.getForeground());
String text = "Hello, world!\nThis is a typing effect example.\n";
for (int i = 0; i < text.length(); i++) {
doc.insertString(doc.getLength(), String.valueOf(text.charAt(i)), style);
TimeUnit.MILLISECONDS.sleep(100); // 暂停 100 毫秒,模拟打字的效果
}
}
}
```
这个程序创建了一个 JFrame 窗口,里面放了一个 JTextPane。程序使用了 JTextPane 的 getStyledDocument 方法获取到一个 StyledDocument 实例,然后使用 addStyle 添加了一个名为 "typing" 的新样式,并设置了它的前景色和其他属性。
接下来,程序使用了一个简单的循环来逐个字符地将文本插入到 JTextPane 中。在每个字符插入后,程序暂停 100 毫秒,模拟打字的效果。注意,插入文本时使用的是之前创建的 "typing" 样式而非默认样式。
希望这个例子能对你有所帮助!
阅读全文
相关推荐
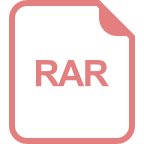
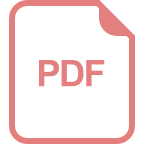
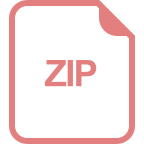
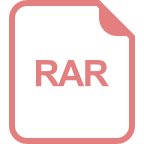
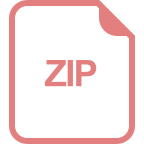
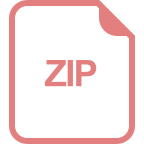
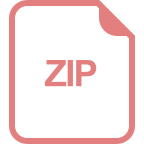
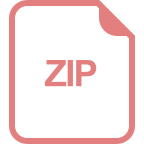
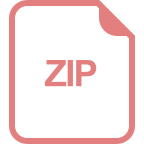
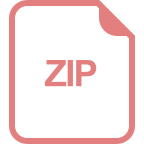
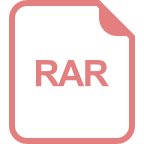
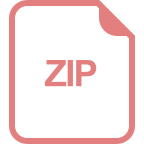