java操作微信进行信息的发送
时间: 2023-09-18 17:16:03 浏览: 49
在Java中操作微信进行信息的发送可以通过使用微信开放平台的API来实现。以下是一个简单的示例代码:
```java
import okhttp3.*;
import java.io.IOException;
public class WeChatSender {
private static final String API_URL = "https://api.weixin.qq.com/cgi-bin/message/custom/send?access_token=%s";
private OkHttpClient client;
public WeChatSender() {
client = new OkHttpClient();
}
public void sendMessage(String accessToken, String openId, String message) throws IOException {
String url = String.format(API_URL, accessToken);
MediaType mediaType = MediaType.parse("application/json; charset=utf-8");
RequestBody requestBody = RequestBody.create(mediaType, buildJson(openId, message));
Request request = new Request.Builder()
.url(url)
.post(requestBody)
.build();
try (Response response = client.newCall(request).execute()) {
System.out.println(response.body().string());
}
}
private String buildJson(String openId, String message) {
return String.format("{\"touser\":\"%s\",\"msgtype\":\"text\",\"text\":{\"content\":\"%s\"}}", openId, message);
}
public static void main(String[] args) throws IOException {
String accessToken = "YOUR_ACCESS_TOKEN";
String openId = "USER_OPENID";
String message = "Hello, World!";
WeChatSender sender = new WeChatSender();
sender.sendMessage(accessToken, openId, message);
}
}
```
在上面的代码中,你需要替换`YOUR_ACCESS_TOKEN`为你的访问令牌(access token),`USER_OPENID`为用户的openid,然后调用`sendMessage`方法即可发送消息。
请注意,该示例代码仅演示了发送文本消息的基本操作,如果你需要发送其他类型的消息,你需要根据微信开放平台的文档调用相应的API接口进行操作。同时,你还需要在微信开放平台注册并创建一个公众号或小程序,并获取到对应的访问令牌(access token)和用户openid。
相关推荐
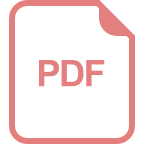














