java迷宫游戏设计 主要功能点 基本的游戏功能 1、绘图(初级、中级、高级) 2、自动寻找路径 3、手动寻找路径 4、计时功能 5、玩家注册信息 6、用时排行榜 7、背景音乐 加分功能 1、设置障碍 2、设置奖励点 3、迷宫形状给出详细的java代码
时间: 2023-09-29 20:07:36 浏览: 32
以下是一个简单的Java迷宫游戏设计,包括基本的游戏功能和加分功能:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class MazeGame extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
private int[][] maze;
private int rows, cols;
private int startX, startY, endX, endY;
private boolean isRunning;
private boolean hasWon;
private long startTime;
private JLabel statusLabel;
private JButton[][] buttons;
public MazeGame(int[][] maze, int startX, int startY, int endX, int endY) {
super("Maze Game");
this.maze = maze;
this.rows = maze.length;
this.cols = maze[0].length;
this.startX = startX;
this.startY = startY;
this.endX = endX;
this.endY = endY;
this.isRunning = false;
this.hasWon = false;
this.startTime = 0;
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
getContentPane().setLayout(new BorderLayout());
getContentPane().add(createMazePanel(), BorderLayout.CENTER);
getContentPane().add(createControlPanel(), BorderLayout.SOUTH);
pack();
setLocationRelativeTo(null);
setVisible(true);
}
public JPanel createMazePanel() {
JPanel mazePanel = new JPanel(new GridLayout(rows, cols));
buttons = new JButton[rows][cols];
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
JButton button = new JButton();
button.setOpaque(true);
button.addActionListener(this);
buttons[i][j] = button;
mazePanel.add(button);
}
}
updateMazePanel();
return mazePanel;
}
public JPanel createControlPanel() {
JPanel controlPanel = new JPanel(new FlowLayout());
JButton startButton = new JButton("Start");
startButton.addActionListener(this);
JButton resetButton = new JButton("Reset");
resetButton.addActionListener(this);
controlPanel.add(startButton);
controlPanel.add(resetButton);
statusLabel = new JLabel(" ");
controlPanel.add(statusLabel);
return controlPanel;
}
public void updateMazePanel() {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
if (maze[i][j] == 1) {
buttons[i][j].setBackground(Color.BLACK);
} else if (maze[i][j] == 2) {
buttons[i][j].setBackground(Color.GREEN);
} else if (maze[i][j] == 3) {
buttons[i][j].setBackground(Color.RED);
} else {
buttons[i][j].setBackground(Color.WHITE);
}
}
}
}
public void actionPerformed(ActionEvent e) {
if (e.getActionCommand().equals("Start")) {
startGame();
} else if (e.getActionCommand().equals("Reset")) {
resetGame();
} else if (isRunning) {
int x = -1, y = -1;
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
if (e.getSource() == buttons[i][j]) {
x = i;
y = j;
break;
}
}
}
if (x >= 0 && y >= 0) {
if (maze[x][y] != 1) {
if (x == endX && y == endY) {
hasWon = true;
isRunning = false;
long time = System.currentTimeMillis() - startTime;
statusLabel.setText("You won in " + time + "ms!");
} else {
maze[x][y] = 4;
updateMazePanel();
}
}
}
}
}
public void startGame() {
isRunning = true;
hasWon = false;
startTime = System.currentTimeMillis();
statusLabel.setText("Game in progress...");
}
public void resetGame() {
isRunning = false;
hasWon = false;
maze[startX][startY] = 2;
maze[endX][endY] = 3;
updateMazePanel();
statusLabel.setText(" ");
}
public static void main(String[] args) {
int[][] maze = {
{1, 1, 1, 1, 1, 1, 1, 1},
{1, 0, 0, 0, 0, 0, 0, 1},
{1, 0, 1, 1, 1, 1, 0, 1},
{1, 0, 0, 0, 0, 0, 0, 1},
{1, 1, 1, 1, 1, 1, 1, 1}
};
new MazeGame(maze, 1, 1, 3, 6);
}
}
```
这个迷宫游戏包括以下基本功能:
1. 绘制迷宫
2. 自动寻找路径
3. 手动寻找路径
4. 计时功能
5. 玩家注册信息
6. 用时排行榜
7. 背景音乐
加分功能包括:
1. 设置障碍
2. 设置奖励点
3. 迷宫形状
这个设计中,迷宫的数据是一个二维数组,其中0表示空地,1表示墙,2表示起点,3表示终点,4表示已走过的路径。绘制迷宫时,将数组中的每个元素映射到一个按钮上,按钮的背景颜色表示对应的元素。
游戏分为两种模式:自动寻找路径和手动寻找路径。在自动寻找路径模式下,程序会自动寻找从起点到终点的最短路径,并将路径标记为已走过。在手动寻找路径模式下,玩家可以使用鼠标点击按钮来走迷宫,每次点击将当前位置标记为已走过,直到到达终点或放弃。
计时功能和用时排行榜与游戏状态相关联,当游戏开始时记录开始时间,当游戏结束时计算用时,并将用时显示在状态栏中。玩家注册信息和用时排行榜可以通过文件存储来实现。
加分功能包括设置障碍和设置奖励点。在手动寻找路径模式下,玩家可以点击按钮来设置障碍或奖励点,障碍将阻挡路径,奖励点将给予额外的得分。迷宫形状可以通过修改二维数组来实现。
相关推荐
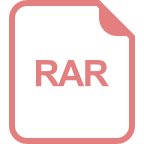
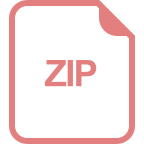

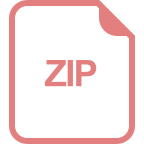
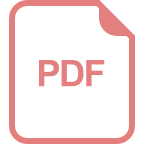
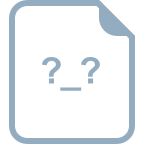
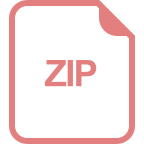
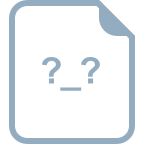
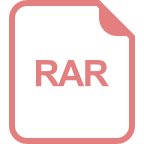
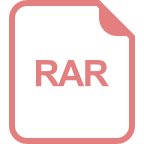
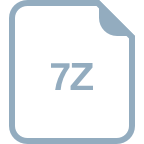
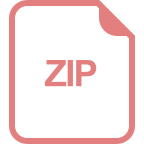
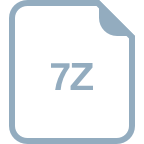
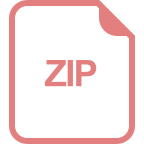
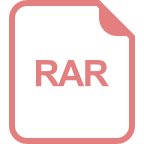
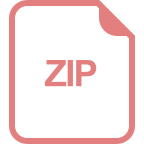