语音质量评估STOI代码
时间: 2023-10-16 21:17:42 浏览: 254
STOI(Short-Time Objective Intelligibility)是一种用于评估语音质量的客观指标,它可以用来衡量音频的清晰度和可懂性。以下是一份计算STOI值的Python代码示例:
```python
import soundfile as sf
import numpy as np
def stoi(clean_audio, noisy_audio, sample_rate):
"""Calculate Short-Time Objective Intelligibility (STOI)"""
clean, _ = sf.read(clean_audio)
noisy, _ = sf.read(noisy_audio)
assert clean.shape == noisy.shape, "Both audios should have the same shape"
clean = clean / np.max(np.abs(clean))
noisy = noisy / np.max(np.abs(noisy))
frame_size = int(sample_rate * 0.030) # 30ms
hop_size = int(sample_rate * 0.010) # 10ms
n_frames = int((len(clean) - frame_size) / hop_size) + 1
clean_stft = np.abs(np.array([np.fft.fft(clean[i:i+frame_size], n=frame_size) for i in range(0, len(clean)-frame_size+1, hop_size)]))
noisy_stft = np.abs(np.array([np.fft.fft(noisy[i:i+frame_size], n=frame_size) for i in range(0, len(noisy)-frame_size+1, hop_size)]))
clean_stft = clean_stft[:, :int(frame_size/2)]
noisy_stft = noisy_stft[:, :int(frame_size/2)]
eps = np.finfo(float).eps
ratio = np.divide(clean_stft, noisy_stft + eps)
log_ratio = np.log10(ratio)
log_ratio[np.isnan(log_ratio)] = 0
mean_log_ratio = np.mean(log_ratio, axis=1)
modified_mean_log_ratio = np.maximum(np.minimum(mean_log_ratio, 1), -1)
stoi_val = np.mean(np.power(10, -0.1 * (modified_mean_log_ratio - 1)), axis=0)
return stoi_val
```
使用方法如下:
```python
clean_audio = "clean.wav"
noisy_audio = "noisy.wav"
sample_rate = 16000
stoi_val = stoi(clean_audio, noisy_audio, sample_rate)
print("STOI value:", stoi_val)
```
其中,`clean_audio`和`noisy_audio`分别是干净语音和带噪语音的文件路径,`sample_rate`是采样率。计算结果以0到1之间的值表示STOI值,值越高表示语音质量越好。
阅读全文
相关推荐
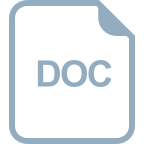
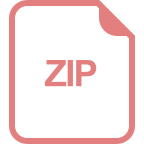
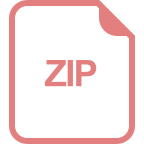
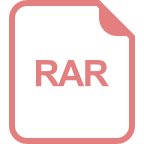
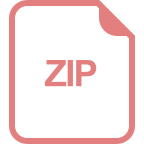
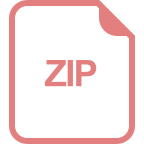
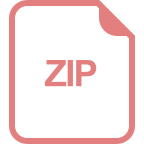
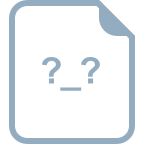
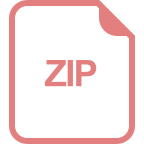
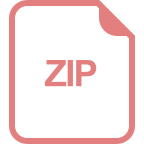
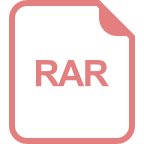
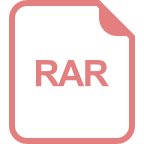
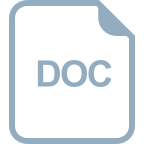
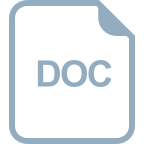
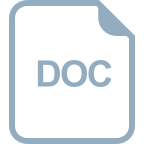
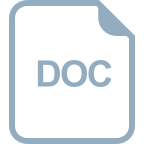