$("#openBtn").click(function(){ $.ajax({ url:"ntp1.php", type:"GET", success:function(response){ var fc = response; $("#fileContent").val(fc); } }); }); $("#saveBtn").click(function(){ var fileContent = $("#fileContent").val(); $.ajax({ url:"ntp1.php", type:"POST", data:{content:fileContent}, success:function(response){ alert("File saved successfully!"); } }); }); $file_path = "/etc/ntp.conf"; $file_content = file_get_contents($file_path); echo $file_content; $file_content1 = $_POST['content']; file_put_contents($file_path,$file_content1); 运行这段代码时 php获取的文件内容 直接输出在网页上 没有输出在文本框中 而且点击打开文件的按钮时 文件内容才输出在文本框中 但是原路经的文件的内容变为空了
时间: 2024-02-15 07:29:09 浏览: 66
这段代码中存在一些问题。可能是由于 PHP 文件的路径不正确或者文件权限的问题导致的。你需要检查一下 `$file_path` 是否正确,同时也需要检查一下文件的权限是否允许读写。
如果路径和权限都没有问题,那么你可以尝试在 PHP 文件中增加一些调试信息,检查一下 `$file_content1` 中是否包含了正确的内容。如果有问题,可能是由于 JavaScript 代码中传递的数据不正确导致的。
另外,在 JavaScript 代码中,你需要检查一下 `#fileContent` 这个文本框是否存在,以及是否绑定了正确的事件。你可以在浏览器的控制台中打印一些调试信息,来帮助你找到问题所在。
相关问题
$("#openBtn").click(function(){ $.ajax({ url:"ntp1.php", type:"GET", success:function(response){ var fc = response; $("#fileContent").val(fc); } }); }); $("#saveBtn").click(function(){ var fileContent = $("#fileContent").val(); $.ajax({ url:"ntp1.php", type:"POST", data:{content:fileContent}, success:function(response){ alert("File saved successfully!"); } }); }); 优化代码
可以优化的地方如下:
1. 将重复的代码提取出来,避免重复书写。
2. 对于 Ajax 请求的 url 和 type 参数可以提取出来,方便维护。
3. 对于 Ajax 请求的 success 回调函数中的重复代码,也可以提取出来。
优化后的代码如下:
```
var url = "ntp1.php";
var type = "GET";
function getFileContent() {
$.ajax({
url: url,
type: type,
success: function(response) {
$("#fileContent").val(response);
}
});
}
function saveFile() {
var fileContent = $("#fileContent").val();
$.ajax({
url: url,
type: "POST",
data: { content: fileContent },
success: function(response) {
alert("File saved successfully!");
}
});
}
$("#openBtn").click(function() {
getFileContent();
});
$("#saveBtn").click(function() {
saveFile();
});
```
$(document).ready(function(){ $("#openBtn").click(function(){ $.ajax({ url:"ntp1.php", type:"GET", success:function(response){ $("#fileContent").val(response); } }); }); 不使用response 获取php $file_path = "/etc/ntp.conf"; $file_content = file_get_contents($file_path); $file_content的内容
如果您想将 `$file_content` 的内容返回给 AJAX 请求,您可以使用 `echo` 将其作为 HTTP 响应的一部分输出,然后在 AJAX 的 `success` 回调函数中获取它。例如,您可以使用以下 PHP 代码:
```
$file_path = "/etc/ntp.conf";
$file_content = file_get_contents($file_path);
echo $file_content;
```
这将读取 `$file_path` 文件的内容并将其输出到 HTTP 响应中。然后,您可以在 AJAX 的 `success` 回调函数中获取它,如下所示:
```
$(document).ready(function(){
$("#openBtn").click(function(){
$.ajax({
url:"ntp1.php",
type:"GET",
success:function(response){
$("#fileContent").val(response);
}
});
});
});
```
当用户单击 `openBtn` 按钮时,将会发送一个 AJAX 请求到 `ntp1.php` 文件。如果请求成功,`success` 回调函数将会被触发,并且 `response` 参数将会包含 PHP 输出的内容。在这个例子中,`response` 将会包含 `$file_content` 的内容,它会设置到 `fileContent` 元素的值中。
阅读全文
相关推荐
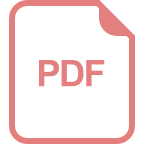
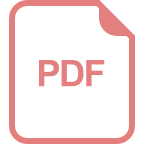
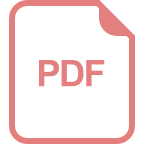







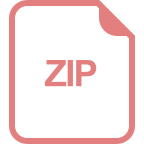
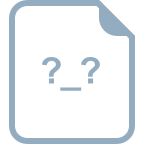
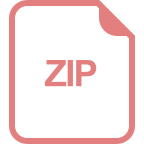
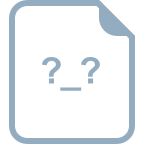