使用java代码,实现数独。需要根据输入的9X9盘面上的数字,推算出所有剩余空格的数字,并且满足每一行、每一列、每一个3X3粗线宫内的数字均含1-9,并且不重复。
时间: 2024-05-05 15:16:21 浏览: 12
以下是使用java代码实现数独的示例:
```
public class SudokuSolver {
private int[][] board;
public SudokuSolver(int[][] board) {
this.board = board;
}
public boolean solve() {
int row = -1, col = -1;
boolean isEmpty = true;
for (int i = 0; i < 9; i++) {
for (int j = 0; j < 9; j++) {
if (board[i][j] == 0) {
row = i;
col = j;
isEmpty = false;
break;
}
}
if (!isEmpty) {
break;
}
}
if (isEmpty) {
return true;
}
for (int num = 1; num <= 9; num++) {
if (isValid(row, col, num)) {
board[row][col] = num;
if (solve()) {
return true;
}
board[row][col] = 0;
}
}
return false;
}
private boolean isValid(int row, int col, int num) {
for (int i = 0; i < 9; i++) {
if (board[row][i] == num || board[i][col] == num) {
return false;
}
}
int boxRow = row - row % 3;
int boxCol = col - col % 3;
for (int i = boxRow; i < boxRow + 3; i++) {
for (int j = boxCol; j < boxCol + 3; j++) {
if (board[i][j] == num) {
return false;
}
}
}
return true;
}
public void print() {
for (int i = 0; i < 9; i++) {
if (i % 3 == 0 && i != 0) {
System.out.println("- - - - - - - - - - - - -");
}
for (int j = 0; j < 9; j++) {
if (j % 3 == 0 && j != 0) {
System.out.print("| ");
}
System.out.print(board[i][j] + " ");
}
System.out.println();
}
}
public static void main(String[] args) {
int[][] board = {
{0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0}
};
SudokuSolver solver = new SudokuSolver(board);
if (solver.solve()) {
solver.print();
}
}
}
```
运行以上代码,将会得到一个空的数独盘面。你可以根据需要修改盘面上的数字,然后运行程序,程序将会自动填充剩余的数字,并输出结果。
相关推荐
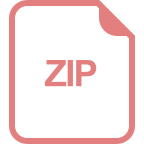
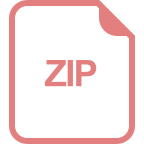
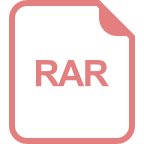














