struct team b = {"kevin",25,99.9}; struct team* d = &b; int i;怎样在后面加一个for循环使得改变里面的name并输出
时间: 2024-03-20 22:45:24 浏览: 40
你可以使用一个 for 循环来遍历一个字符数组,然后将其赋值给结构体中的 name 字段。代码可以这样写:
```
#include <stdio.h>
#include <string.h>
struct team {
char name[20];
int age;
float score;
};
int main() {
struct team b = {"kevin", 25, 99.9};
struct team* d = &b;
int i;
for (i = 0; i < strlen("new name"); i++) {
d->name[i] = "new name"[i];
}
printf("Name: %s, Age: %d, Score: %f\n", d->name, d->age, d->score);
return 0;
}
```
这段代码将 "new name" 赋值给了结构体中的 name 字段,并输出了结果。注意,我们使用了 strlen 函数来获取 "new name" 的长度,以便遍历字符数组。
相关问题
查找错误并举出、修改#define _CRT_SECURE_NO_WARNINGS 1 #include<stdio.h> typedef struct num { float a; //系数 int b; //指数 struct num *next; }*num; struct LinkList // 链表类型 { num head;// 分别指向线性链表中的头结点和最后一个结点 感觉不需要tail int len; // 指示线性链表中数据元素的个数 }; struct LinkList *init(struct LinkList *list)//创建空链表 { list = (struct LinkList *)malloc(sizeof(struct LinkList)); list->len = 0; list->head = (struct num*)malloc(sizeof(struct num));//list->tail = list->head->next = NULL;//list->tail->next = return list; }; void compare(struct LinkList *list, float a, int b)//比较指数 { int i = 0; struct num*p = list->head; for (i; i <= list->len; i++) { if (b > p->b) p = p->next; else if (b = p->b){ p->b += b; break; } else{ add(list, i, a, b);//插入 break; } } if (i>list->len) add(list, i, a, b);//添加到最后一个 }; void add(struct LinkList *list, int index, float a,int b)//添加新的指数项 { struct num*p = list->head, *s; int i; for (i = -1; i<index - 1; i++) { p = p->next; } s = (struct num *)malloc(sizeof(struct num)); s->a = a; s->b = b; s->next = p->next; p->next = s; list->len++; //if (index == list->len) 感觉不需要尾结点 // list->tail = s; }; int main(){ //指数升序查找 struct LinkList *lista, *listb ; lista = init(lista); listb = init(listb); int n,b; float a; scanf("%d", &n); for (int i = 0; i < n; i++)//lista { scanf("%f%d", &a,&b); compare(lista, a, b); } scanf("%d", &n); for (int i = 0; i < n; i++)//listb { scanf("%f%d", &a, &b); compare(listb, a, b); } return 0; }
在这段代码中,有两个问题:
1. 在定义结构体 `num` 时,没有为 `next` 成员指定类型,应该将 `*num` 改为 `struct num *`。
2. 在函数 `init` 中,没有为头结点的 `next` 成员赋值,应该为其赋值为 `NULL`。
下面是修改后的代码:
```c
#define _CRT_SECURE_NO_WARNINGS 1
#include <stdio.h>
#include <stdlib.h>
typedef struct num {
float a; //系数
int b; //指数
struct num* next;
} *num;
struct LinkList // 链表类型
{
num head; // 分别指向线性链表中的头结点和最后一个结点
int len; // 指示线性链表中数据元素的个数
};
struct LinkList* init(struct LinkList* list) //创建空链表
{
list = (struct LinkList*)malloc(sizeof(struct LinkList));
list->len = 0;
list->head = (num)malloc(sizeof(struct num));
list->head->next = NULL;
return list;
};
void compare(struct LinkList* list, float a, int b) //比较指数
{
int i = 0;
num p = list->head;
for (i; i <= list->len; i++)
{
if (b > p->b)
p = p->next;
else if (b == p->b) {
p->a += a;
break;
}
else {
add(list, i, a, b); //插入
break;
}
}
if (i > list->len)
add(list, i, a, b); //添加到最后一个
};
void add(struct LinkList* list, int index, float a, int b) //添加新的指数项
{
num p = list->head, *s;
int i;
for (i = -1; i < index - 1; i++)
{
p = p->next;
}
s = (num)malloc(sizeof(struct num));
s->a = a;
s->b = b;
s->next = p->next;
p->next = s;
list->len++;
};
int main()
{
//指数升序查找
struct LinkList* lista, * listb;
lista = init(lista);
listb = init(listb);
int n, b;
float a;
scanf("%d", &n);
for (int i = 0; i < n; i++) //lista
{
scanf("%f%d", &a, &b);
compare(lista, a, b);
}
scanf("%d", &n);
for (int i = 0; i < n; i++) //listb
{
scanf("%f%d", &a, &b);
compare(listb, a, b);
}
return 0;
}
```
#include <stdio.h>#include <stdlib.h>struct ENode { int adjVex; int w; struct ENode* nextArc;};struct ENode** a; //邻接表int* cost; //cost[i]表示i节点到汇点的最短路径长度int n; //总节点数int m; //总边数int i; //循环变量void CreatGraph(); //构建邻接表void FMultiGraph(); //多段图的向前递推算法int main() { scanf("%d%d", &n, &m); a = (struct ENode**) malloc(n * sizeof(struct ENode*)); CreatGraph(); FMultiGraph(); printf("%d\n", cost[0]); free(cost); for (i = 0; i < n; i++) free(a[i]); free(a); return 0;}//构建邻接表void CreatGraph() { int u, v; int w; struct ENode* t; //临时变量 for (i = 0; i < n; i++) a[i] = NULL; for (i = 0; i < m; i++) { scanf("%d%d%d", &u, &v, &w); t = (struct ENode*) malloc(sizeof(struct ENode)); t->adjVex = v; t->nextArc = a[u]; t->w = w; a[u] = t; }}//多段图的向前递推算法void FMultiGraph() { cost = (int*) malloc(n * sizeof(int)); cost[n - 1] = 0; //汇点到汇点的最短路径长度为0 struct ENode* r; //临时变量 int min; for (i = n - 2; i >= 0; i--) { min = 0x7fffffff; for (r = a[i]; r; r = r->nextArc) { if (r->w + cost[r->adjVex] < min) min = r->w + cost[r->adjVex]; } cost[i] = min; }}加一串记录路径,可以在构建邻接表时再添加一个数组用于记录前驱节点,然后在向前递推算法中回溯路径输出路径的代码
可以在节点的结构体中添加一个前驱节点的指针,然后在构建邻接表时初始化该指针,记录每个节点的前驱节点。在向前递推算法中,除了记录最短路径长度之外,还需要记录每个节点的前驱节点,最后回溯路径输出即可。
修改后的代码如下:
#include <stdio.h>
#include <stdlib.h>
struct ENode {
int adjVex;
int w;
struct ENode* nextArc;
};
struct Node {
struct ENode* firstArc;
struct Node* preNode; //前驱节点指针
};
struct ENode** a; //邻接表
struct Node* nodes; //节点数组
int* cost; //cost[i]表示i节点到汇点的最短路径长度
int n; //总节点数
int m; //总边数
int i; //循环变量
void CreatGraph(); //构建邻接表
void FMultiGraph(); //多段图的向前递推算法
void PrintPath(); //输出路径
int main() {
scanf("%d%d", &n, &m);
a = (struct ENode**) malloc(n * sizeof(struct ENode*));
nodes = (struct Node*) malloc(n * sizeof(struct Node)); //节点数组初始化
CreatGraph();
FMultiGraph();
printf("%d\n", cost[0]);
PrintPath(); //输出路径
free(cost);
for (i = 0; i < n; i++) {
struct ENode* p = a[i];
while (p) {
struct ENode* q = p->nextArc;
free(p);
p = q;
}
}
free(a);
free(nodes); //释放节点数组
return 0;
}
//构建邻接表
void CreatGraph() {
int u, v;
int w;
struct ENode* t; //临时变量
for (i = 0; i < n; i++) {
a[i] = NULL;
nodes[i].preNode = NULL; //前驱节点指针初始化
}
for (i = 0; i < m; i++) {
scanf("%d%d%d", &u, &v, &w);
t = (struct ENode*) malloc(sizeof(struct ENode));
t->adjVex = v;
t->nextArc = a[u];
t->w = w;
a[u] = t;
//记录前驱节点
if (nodes[v].preNode == NULL || nodes[v].preNode > &nodes[u]) {
nodes[v].preNode = &nodes[u];
}
}
}
//多段图的向前递推算法
void FMultiGraph() {
cost = (int*) malloc(n * sizeof(int));
cost[n - 1] = 0; //汇点到汇点的最短路径长度为0
struct ENode* r; //临时变量
int min;
for (i = n - 2; i >= 0; i--) {
min = 0x7fffffff;
for (r = a[i]; r; r = r->nextArc) {
if (r->w + cost[r->adjVex] < min) {
min = r->w + cost[r->adjVex];
//更新前驱节点指针
nodes[i].preNode = &nodes[r->adjVex];
}
}
cost[i] = min;
}
}
//输出路径
void PrintPath() {
struct Node* p = &nodes[0];
printf("0");
while (p->preNode != NULL) {
printf("->%d", (int)(p - nodes));
p = p->preNode;
}
printf("\n");
}
阅读全文
相关推荐
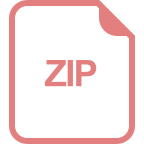
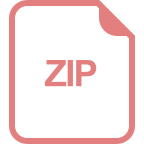
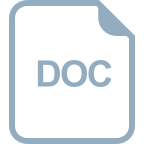
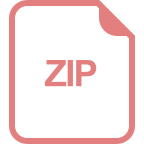
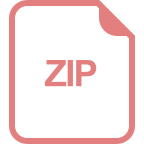
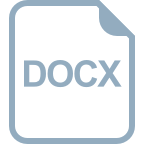
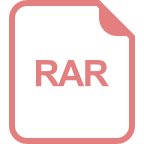
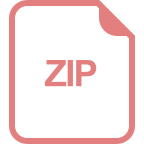
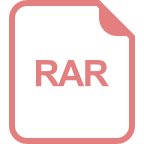
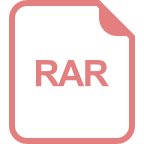
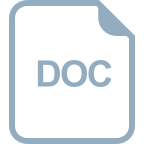
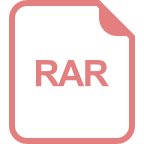
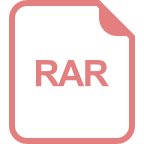
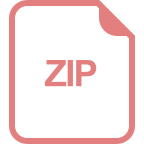