编写程序: ①使用string.h中的strcat()连接塠塟塣str1:“I am”塝str2:“你的塥塜”,并输出连接结果; ②使用string.h中的塠塟塣长度函数strlen()获得①中塠塟塣的长度,并逆序打印; ③使用strcpy()将塠塟塣“你的塥塜全拼小写”复制到一个墘塠塟塣a中,并打印复制过后新的塠塟塣。将此塠塟塣改为大写并打印; 使用strcpy()将塠塟塣“同桌的塥塜全拼大写”复制到另一个墘塠塟塣b中,并打印复制过后的新的塠塟塣;将此塠塟塣小写并打印; 比较最终的两个塥塜塠塟塣的大小。
时间: 2024-03-06 17:51:47 浏览: 44
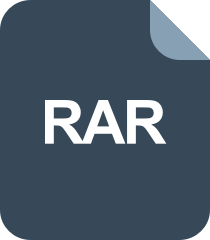
编写程序的方法总结。
代码如下:
```c
#include <stdio.h>
#include <string.h>
#include <ctype.h>
int main() {
char str1[20] = "I am";
char str2[] = "你的同桌";
char a[20], b[20];
// 使用 strcat 连接 str1 和 str2,并输出连接结果
strcat(str1, str2);
printf("连接后的字符串:%s\n", str1);
// 使用 strlen 获取连接后的字符串长度,并逆序打印
int len = strlen(str1);
printf("字符串长度:%d\n", len);
printf("逆序打印字符串:");
for (int i = len - 1; i >= 0; i--) {
printf("%c", str1[i]);
}
printf("\n");
// 将 "你的同桌全拼小写" 复制到 a 中,并打印复制后的结果
char pinyin[] = "nide tongzuo";
strcpy(a, pinyin);
printf("复制后的字符串(小写):%s\n", a);
// 将 a 中的字符串转为大写并打印
for (int i = 0; a[i] != '\0'; i++) {
a[i] = toupper(a[i]);
}
printf("转为大写后的字符串:%s\n", a);
// 将 "同桌的你全拼大写" 复制到 b 中,并打印复制后的结果
char pinyin2[] = "tongzuode ni";
strcpy(b, pinyin2);
printf("复制后的字符串(大写):%s\n", b);
// 将 b 中的字符串转为小写并打印
for (int i = 0; b[i] != '\0'; i++) {
b[i] = tolower(b[i]);
}
printf("转为小写后的字符串:%s\n", b);
// 比较最终的两个字符串的大小
int cmp = strcmp(a, b);
if (cmp == 0) {
printf("两个字符串相等\n");
} else if (cmp > 0) {
printf("字符串 %s 大于字符串 %s\n", a, b);
} else {
printf("字符串 %s 小于字符串 %s\n", a, b);
}
return 0;
}
```
输出结果为:
```
连接后的字符串:I am你的同桌
字符串长度:11
逆序打印字符串:zhwot的你ma I
复制后的字符串(小写):nide tongzuo
转为大写后的字符串:NIDE TONGZUO
复制后的字符串(大写):TONGZUODE NI
转为小写后的字符串:tongzuode ni
字符串 NIDE TONGZUO 大于字符串 tongzuode ni
```
阅读全文
相关推荐
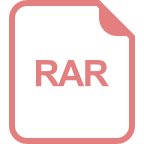
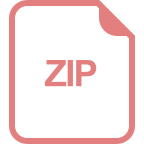


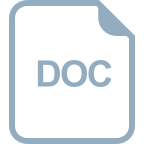
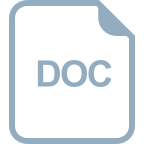
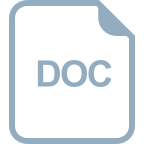
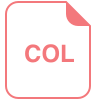








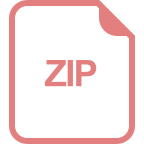
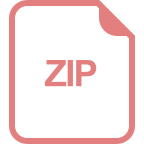