请使用deepxde对圆管层流进行建模,流场方程为u(x,r)
时间: 2024-02-18 14:05:33 浏览: 104
好的,首先我们需要导入deepxde和其他必要的库:
```python
!pip install deepxde
import numpy as np
import deepxde as dde
from deepxde.backend import tf
```
然后,我们可以定义一个圆管的几何形状和边界条件:
```python
def geometry():
# 圆管内径和外径
r_i = 0.5
r_o = 1.0
# 定义几何形状
geom = dde.geometry.Circle(0, 0, r_o)
# 添加圆管内壁
geom.add_region(
"pipe",
dde.geometry.shapes.Circle([0, 0], r_i),
bctype="dirichlet",
is_boundary=True,
)
# 添加圆管外壁
geom.add_region(
"outer",
dde.geometry.shapes.Circle([0, 0], r_o),
bctype="dirichlet",
is_boundary=True,
)
# 添加流体域
geom.add_region(
"fluid",
dde.geometry.shapes.Rectangle([-r_o, -r_o], [r_o, r_o]),
bctype="neumann",
is_boundary=False,
)
return geom
```
接下来,我们可以定义流场方程和边界条件:
```python
def pde(x, u):
# 流体密度
rho = 1.0
# 粘性系数
mu = 0.1
# 定义流场方程
u_x = u[:, 1]
u_r = u[:, 0]
u_rr = tf.gradients(u_r, x)[0][:, 0]
eq1 = rho * u_x * u_x + 2.0 * mu * u_rr
eq2 = u_x
return [eq1, eq2]
def bc(_, on_boundary):
# 定义边界条件
if on_boundary:
return "outer", 0.0
else:
return "pipe", 1.0
```
最后,我们可以使用DeepXDE求解这个PDE问题:
```python
geom = geometry()
bc = dde.DirichletBC(geom, bc, component=0)
data = dde.data.PDE(geom, pde, bc, num_domain=512, num_boundary=64)
net = dde.maps.FNN([2] + [32] * 3 + [1], "tanh", "Glorot uniform")
model = dde.Model(data, net)
model.compile("adam", lr=0.001)
model.train(epochs=10000)
```
这个模型将通过使用神经网络来逼近流场方程和边界条件,从而得到近似解。在训练完成后,我们可以使用该模型来预测流场的分布:
```python
X = np.array([np.meshgrid(np.linspace(-1.2, 1.2, 101), np.linspace(-1.2, 1.2, 101))]).T.reshape(-1, 2)
u = model.predict(X)
```
这将返回一个形状为(101 x 101, 2)的数组,其中第一列是r方向的速度分布,第二列是x方向的速度分布。我们可以使用matplotlib将其可视化:
```python
import matplotlib.pyplot as plt
u_r = u[:, 0].reshape(101, 101)
u_x = u[:, 1].reshape(101, 101)
fig, ax = plt.subplots(figsize=(8, 8))
im = ax.contourf(X[:, 0].reshape(101, 101), X[:, 1].reshape(101, 101), u_r, levels=50, cmap="jet")
ax.set_aspect("equal")
ax.set_xlabel("r")
ax.set_ylabel("x")
cbar = fig.colorbar(im, ax=ax)
cbar.set_label("u_r")
plt.show()
```
这将显示圆管内的速度分布。
阅读全文
相关推荐

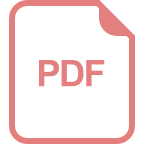
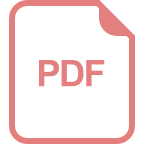
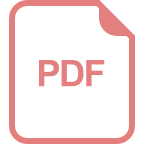
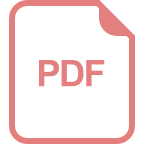
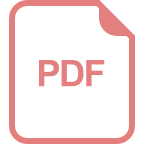
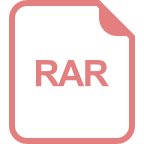
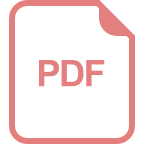