C++ 直线在多边形内部 源代码
时间: 2023-06-26 10:09:04 浏览: 220
以下是一个简单的 C++ 实现,用于检查一条线段是否与多边形相交,以及该线段是否完全位于多边形内部。
```c++
#include <iostream>
#include <vector>
#include <cmath>
using namespace std;
struct Point {
double x, y;
};
struct Line {
Point p1, p2;
};
double cross_product(Point p1, Point p2, Point p3) {
return (p2.x - p1.x) * (p3.y - p1.y) - (p3.x - p1.x) * (p2.y - p1.y);
}
bool is_point_on_line(Point p, Line l) {
double cp1 = cross_product(l.p1, l.p2, p);
double cp2 = cross_product(l.p2, l.p1, p);
return cp1 == 0 && cp2 == 0;
}
bool is_point_in_polygon(Point p, vector<Point> polygon) {
int n = polygon.size();
if (n < 3) return false;
double angle_sum = 0;
for (int i = 0; i < n; i++) {
Point p1 = polygon[i];
Point p2 = polygon[(i + 1) % n];
if (is_point_on_line(p, {p1, p2})) return true;
double angle = atan2(p2.y - p.y, p2.x - p.x) - atan2(p1.y - p.y, p1.x - p.x);
while (angle < 0) angle += 2 * M_PI;
while (angle > 2 * M_PI) angle -= 2 * M_PI;
angle_sum += angle;
}
return fabs(angle_sum - 2 * M_PI) < 1e-9;
}
bool is_line_in_polygon(Line l, vector<Point> polygon) {
return is_point_in_polygon(l.p1, polygon) && is_point_in_polygon(l.p2, polygon);
}
int main() {
// 示例:多边形顶点坐标
vector<Point> polygon = {{0, 0}, {0, 1}, {1, 1}, {1, 0}};
// 示例:测试线段坐标
Line line = {{0.5, 0.5}, {1.5, 1.5}};
if (is_line_in_polygon(line, polygon)) {
cout << "Line is inside polygon." << endl;
} else {
cout << "Line is outside polygon." << endl;
}
return 0;
}
```
该代码使用叉积判断一条线段是否与多边形相交,使用角度和判断一条线段是否完全位于多边形内部。注意,由于浮点数计算精度问题,判断角度和时需要使用 `fabs` 函数进行误差修正。
阅读全文
相关推荐
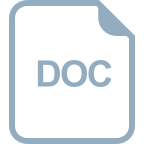
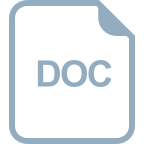
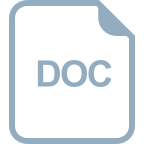
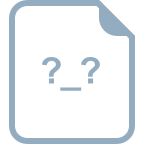
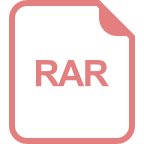
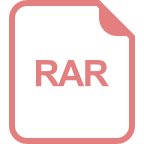
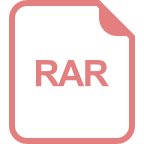
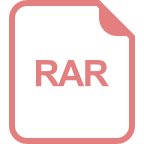
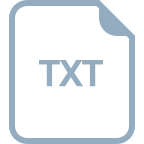
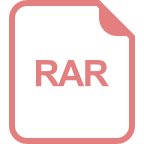
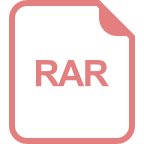
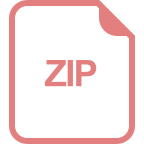
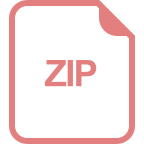
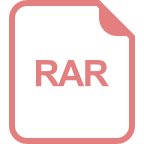
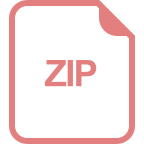
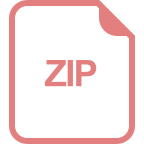
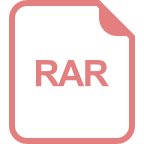
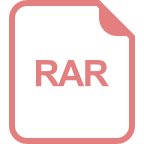
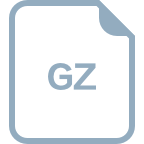