uniapp小程序高德地图循环渲染店铺坐标
时间: 2023-10-02 21:13:02 浏览: 101
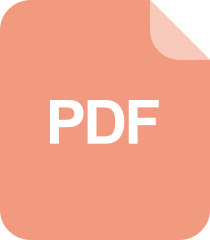
微信小程序 高德地图路线规划实现过程详解
你可以使用高德地图的 JavaScript API,在地图上循环渲染店铺坐标。具体实现步骤如下:
1. 引入高德地图 JavaScript API
```html
<template>
<view>
<map :id="mapId" :longitude="longitude" :latitude="latitude" :scale="scale" :markers="markers"></map>
</view>
</template>
<script>
import { mapState } from 'vuex';
export default {
computed: mapState(['longitude', 'latitude', 'scale', 'markers']),
data() {
return {
mapId: 'myMap'
};
},
onLoad() {
wx.getLocation({
type: 'gcj02',
success: (res) => {
this.$store.commit('setLongitude', res.longitude);
this.$store.commit('setLatitude', res.latitude);
}
});
}
};
</script>
<style>
map {
width: 100%;
height: 100%;
}
</style>
```
2. 在 `onLoad` 生命周期中获取当前位置的经纬度,并使用 Vuex 存储
```js
onLoad() {
wx.getLocation({
type: 'gcj02',
success: (res) => {
this.$store.commit('setLongitude', res.longitude);
this.$store.commit('setLatitude', res.latitude);
}
});
}
```
3. 在 Vuex 中存储当前位置的经纬度、地图缩放级别和需要渲染的店铺坐标
```js
const state = {
longitude: 0, // 当前位置经度
latitude: 0, // 当前位置纬度
scale: 14, // 地图缩放级别
markers: [] // 店铺坐标
};
const mutations = {
setLongitude(state, longitude) {
state.longitude = longitude;
},
setLatitude(state, latitude) {
state.latitude = latitude;
},
setMarkers(state, markers) {
state.markers = markers;
}
};
```
4. 循环获取需要渲染的店铺坐标,并存储到 Vuex 中
```js
wx.request({
url: 'https://your-api.com/get-shops',
success: (res) => {
const shops = res.data;
const markers = [];
for (let i = 0; i < shops.length; i++) {
const shop = shops[i];
markers.push({
id: shop.id,
longitude: shop.longitude,
latitude: shop.latitude,
iconPath: '/static/images/marker.png', // 自定义图标
width: 30,
height: 30,
callout: {
content: shop.name,
color: '#ffffff',
fontSize: 14,
bgColor: '#555555',
padding: 10,
borderRadius: 5,
display: 'ALWAYS'
}
});
}
this.$store.commit('setMarkers', markers);
}
});
```
5. 在模板中使用地图组件,并绑定需要渲染的经纬度和店铺坐标
```html
<template>
<view>
<map :id="mapId" :longitude="longitude" :latitude="latitude" :scale="scale" :markers="markers"></map>
</view>
</template>
<script>
import { mapState } from 'vuex';
export default {
computed: mapState(['longitude', 'latitude', 'scale', 'markers']),
data() {
return {
mapId: 'myMap'
};
},
onLoad() {
wx.getLocation({
type: 'gcj02',
success: (res) => {
this.$store.commit('setLongitude', res.longitude);
this.$store.commit('setLatitude', res.latitude);
}
});
wx.request({
url: 'https://your-api.com/get-shops',
success: (res) => {
const shops = res.data;
const markers = [];
for (let i = 0; i < shops.length; i++) {
const shop = shops[i];
markers.push({
id: shop.id,
longitude: shop.longitude,
latitude: shop.latitude,
iconPath: '/static/images/marker.png',
width: 30,
height: 30,
callout: {
content: shop.name,
color: '#ffffff',
fontSize: 14,
bgColor: '#555555',
padding: 10,
borderRadius: 5,
display: 'ALWAYS'
}
});
}
this.$store.commit('setMarkers', markers);
}
});
}
};
</script>
<style>
map {
width: 100%;
height: 100%;
}
</style>
```
这样,你就可以在地图上循环渲染店铺坐标了。
阅读全文
相关推荐
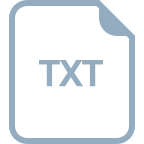
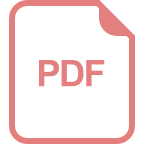


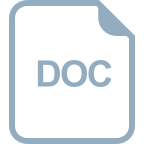












