windows C语言使用mqtt推送json字符串
时间: 2023-07-31 08:05:37 浏览: 123
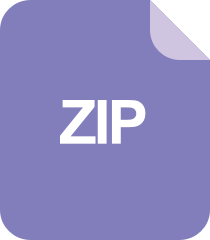
C语言检测字符串是否为json字符串

要在 Windows C 语言中使用 MQTT 推送 JSON 字符串,可以使用开源的 mqtt-c 客户端库。具体步骤如下:
1. 安装 mqtt-c 库
可以在 mqtt-c 的 GitHub 页面下载最新的 release 版本,将其中的 `include` 和 `lib` 文件夹拷贝到你的项目中,并在 Visual Studio 中配置 include 路径和链接库。
2. 连接 MQTT 服务器
使用 `mqtt_client_new` 函数创建 MQTT 客户端,然后使用 `mqtt_connect` 函数连接 MQTT 服务器。例如:
```c
mqtt_client_t* client = mqtt_client_new();
mqtt_connect(client, "localhost", 1883, 60);
```
其中,`localhost` 和 `1883` 分别为 MQTT 服务器的地址和端口号,`60` 表示连接超时时间(单位为秒)。
3. 发布 JSON 字符串
使用 `mqtt_publish` 函数发布 JSON 字符串。例如:
```c
char* json_str = "{\"name\": \"John\", \"age\": 30}";
mqtt_publish(client, "topic", json_str, strlen(json_str), 0, 0);
```
其中,`"topic"` 为要发布到的主题,`json_str` 为 JSON 字符串的指针,`strlen(json_str)` 为字符串长度,`0` 表示 QoS 等级为 0,`0` 表示不保留消息。
4. 断开 MQTT 连接
使用 `mqtt_disconnect` 函数断开 MQTT 连接,然后使用 `mqtt_client_destroy` 函数销毁 MQTT 客户端。例如:
```c
mqtt_disconnect(client);
mqtt_client_destroy(&client);
```
完整的代码如下:
```c
#include <stdio.h>
#include <string.h>
#include "mqtt.h"
int main() {
mqtt_client_t* client = mqtt_client_new();
mqtt_connect(client, "localhost", 1883, 60);
char* json_str = "{\"name\": \"John\", \"age\": 30}";
mqtt_publish(client, "topic", json_str, strlen(json_str), 0, 0);
mqtt_disconnect(client);
mqtt_client_destroy(&client);
return 0;
}
```
注意,在使用 `mqtt_publish` 函数时,需要确保 JSON 字符串的格式正确,否则可能会导致消息无法正常传输。
阅读全文
相关推荐


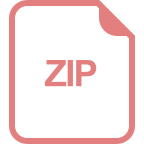
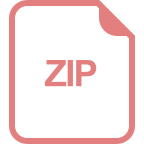
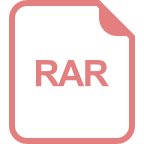
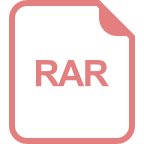
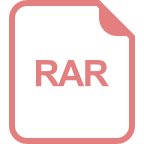
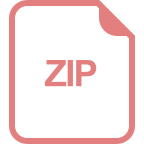
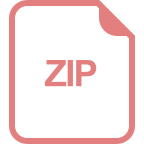
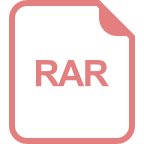
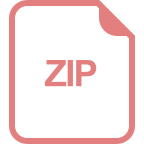
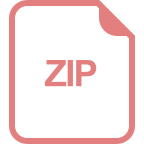
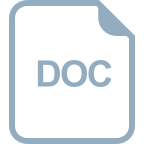
