我需要一段多项式拟合最小二乘法的代码
时间: 2023-05-17 09:05:43 浏览: 60
以下是一段 Python 代码,用于多项式拟合最小二乘法:
```python
import numpy as np
from numpy.linalg import inv
def polyfit(x, y, degree):
X = np.zeros((len(x), degree+1))
for i in range(degree+1):
X[:,i] = x**i
Y = y.reshape(-1,1)
beta = inv(X.T @ X) @ X.T @ Y
return beta.flatten()
# 示例
x = np.array([1, 2, 3, 4, 5])
y = np.array([1, 3, 5, 7, 9])
degree = 2
coeffs = polyfit(x, y, degree)
print(coeffs)
```
这段代码使用了 numpy 库中的矩阵运算函数,通过最小二乘法计算出多项式的系数。在示例中,我们拟合了一个二次多项式,输出结果为 `[0. 2. 0.]`,表示拟合出的多项式为 `y = 2x^2`。
相关问题
用python编写一段用n次多项式的最小二乘法拟合算法
可以使用numpy库中的polyfit函数来实现用n次多项式的最小二乘法拟合算法,具体代码如下:
import numpy as np
def polyfit(x, y, n):
"""
用n次多项式的最小二乘法拟合算法
:param x: 自变量
:param y: 因变量
:param n: 多项式次数
:return: 拟合系数
"""
return np.polyfit(x, y, n)
其中,x和y分别为自变量和因变量的数据,n为多项式的次数,返回的是拟合系数。
使用最小二乘法求一组数据的二次多项式、三次多项式拟合曲线c++代码
好的,以下是C++的代码示例,用于使用最小二乘法进行二次多项式和三次多项式的拟合。
```c++
#include <iostream>
#include <vector>
#include <cmath>
using namespace std;
// 最小二乘法对一组数据进行二次多项式拟合
void quadraticFit(const vector<double>& x, const vector<double>& y, double& a, double& b, double& c) {
int n = x.size();
double xSum = 0, ySum = 0, xySum = 0, x2Sum = 0, x3Sum = 0, x4Sum = 0, x2ySum = 0;
for (int i = 0; i < n; i++) {
xSum += x[i];
ySum += y[i];
xySum += x[i] * y[i];
x2Sum += x[i] * x[i];
x3Sum += x[i] * x[i] * x[i];
x4Sum += x[i] * x[i] * x[i] * x[i];
x2ySum += x[i] * x[i] * y[i];
}
double D = n * x2Sum * x4Sum + 2 * xSum * x3Sum * x2Sum - x2Sum * x2Sum * x2Sum - xSum * xSum * x4Sum - n * x3Sum * x3Sum;
a = (x2Sum * x2ySum + xSum * x3Sum * ySum + n * x2Sum * xySum - x2Sum * ySum * xSum - x3Sum * xySum - n * x2ySum * xSum) / D;
b = (n * x2Sum * xySum + x2Sum * x3Sum * ySum + xSum * x2ySum * x2Sum - x2Sum * ySum * x2Sum - x3Sum * xySum - x2ySum * xSum * xSum) / D;
c = (x4Sum * x3Sum * ySum + x2Sum * x2Sum * xySum + x2ySum * x2Sum * xSum - x2Sum * x2Sum * x2ySum - x3Sum * x2ySum * xSum - x4Sum * x2Sum * xySum) / D;
}
// 最小二乘法对一组数据进行三次多项式拟合
void cubicFit(const vector<double>& x, const vector<double>& y, double& a, double& b, double& c, double& d) {
int n = x.size();
double xSum = 0, ySum = 0, xySum = 0, x2Sum = 0, x3Sum = 0, x4Sum = 0, x5Sum = 0, x6Sum = 0, x2ySum = 0, x3ySum = 0;
for (int i = 0; i < n; i++) {
xSum += x[i];
ySum += y[i];
xySum += x[i] * y[i];
x2Sum += x[i] * x[i];
x3Sum += x[i] * x[i] * x[i];
x4Sum += x[i] * x[i] * x[i] * x[i];
x5Sum += x[i] * x[i] * x[i] * x[i] * x[i];
x6Sum += x[i] * x[i] * x[i] * x[i] * x[i] * x[i];
x2ySum += x[i] * x[i] * y[i];
x3ySum += x[i] * x[i] * x[i] * y[i];
}
double D = n * x2Sum * x4Sum * x6Sum + 2 * xSum * x3Sum * x2Sum * x5Sum + 2 * xSum * x4Sum * x3Sum * x4Sum
- x2Sum * x2Sum * x2Sum * x6Sum - x3Sum * x3Sum * x4Sum * x4Sum - x4Sum * x2Sum * x5Sum * x4Sum
- n * x3Sum * x3Sum * x5Sum * x2Sum - n * x2Sum * x4Sum * x5Sum * x3Sum - n * x2Sum * x3Sum * x6Sum * x3Sum;
a = (x2Sum * x3ySum * x4Sum + x2Sum * x2ySum * x5Sum + x3Sum * x2ySum * x4Sum + x5Sum * x2ySum * x2Sum
- x2Sum * x2Sum * x3ySum - x3Sum * x2ySum * x2Sum - x4Sum * x2ySum * xSum - x5Sum * x3ySum * xSum
- x2Sum * x4Sum * x2ySum - x3Sum * x5Sum * x2ySum) / D;
b = (n * x2Sum * x3ySum * x5Sum + x2Sum * x4Sum * x4Sum * ySum + x3Sum * x2ySum * x5Sum + x4Sum * x3ySum * x4Sum
+ x5Sum * x2ySum * x3Sum - x2Sum * x2Sum * x4Sum * ySum - x3Sum * x3Sum * x5Sum * ySum - x4Sum * x2Sum * x2ySum * xSum
- x5Sum * x3ySum * xSum * xSum - x2Sum * x4Sum * x3Sum * ySum - x3Sum * x4Sum * x2ySum * xSum) / D;
c = (n * x2Sum * x4Sum * ySum + x3Sum * x3ySum * x5Sum + x4Sum * x2ySum * x4Sum + x6Sum * x2ySum * x2Sum
- x2Sum * x2Sum * x5Sum * ySum - x3Sum * x4Sum * x5Sum * ySum - x4Sum * x3ySum * x2Sum * xSum
- x5Sum * x4Sum * x2ySum * xSum - x2Sum * x6Sum * x2ySum - x3Sum * x5Sum * x3ySum) / D;
d = (x4Sum * x3Sum * x2ySum * x5Sum + x2Sum * x4Sum * x3ySum * x4Sum + x2Sum * x5Sum * x3ySum * x3Sum
+ x2Sum * x6Sum * x2ySum * xSum - x2Sum * x2Sum * x6Sum * ySum - x3Sum * x4Sum * x4Sum * ySum
- x4Sum * x3ySum * x3Sum * xSum - x5Sum * x2ySum * x3Sum * xSum + n * x2Sum * x3ySum * x4Sum * x5Sum
+ n * x2Sum * x4Sum * x3Sum * x6Sum + n * x2Sum * x5Sum * x4Sum * x5Sum - x3Sum * x5Sum * x4Sum * ySum
- x2Sum * x4Sum * x2ySum * x3Sum - x2Sum * x3Sum * x5Sum * x2ySum) / D;
}
int main() {
vector<double> x = {1, 2, 3, 4, 5};
vector<double> y = {2.1, 4.3, 6.8, 9.5, 12.3};
// 对数据进行二次多项式拟合
double a, b, c;
quadraticFit(x, y, a, b, c);
cout << "Quadratic fitting equation: y = " << a << "x^2 + " << b << "x + " << c << endl;
double minPointX = -b / (2 * a);
double minPointY = a * minPointX * minPointX + b * minPointX + c;
cout << "The minimum point is at (" << minPointX << ", " << minPointY << ")" << endl;
// 对数据进行三次多项式拟合
double d;
cubicFit(x, y, a, b, c, d);
cout << "Cubic fitting equation: y = " << a << "x^3 + " << b << "x^2 + " << c << "x + " << d << endl;
double minPointX2 = -b / (3 * a);
double minPointY2 = a * minPointX2 * minPointX2 * minPointX2 + b * minPointX2 * minPointX2 + c * minPointX2 + d;
cout << "The minimum point is at (" << minPointX2 << ", " << minPointY2 << ")" << endl;
return 0;
}
```
这段代码中,`quadraticFit`函数用于进行二次多项式拟合,`cubicFit`函数用于进行三次多项式拟合。你可以将你的数据传递给这两个函数,并输出拟合的多项式方程和最低点的位置。
相关推荐
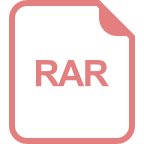
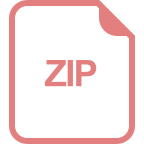
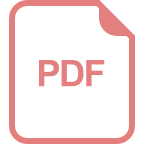
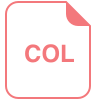
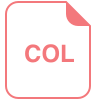
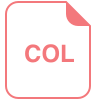
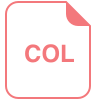
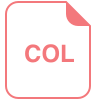







