C# opencvsharp点云拼图
时间: 2023-08-28 11:04:48 浏览: 71
要在C#中使用OpenCVSharp进行点云拼图,需要掌握以下步骤:
1. 读取点云文件:使用OpenCVSharp的PCL模块读取点云数据。点云数据可以是PCL格式,也可以是其他格式。
2. 特征点提取:使用OpenCVSharp的SURF或SIFT算法提取点云中的特征点。特征点可以视为点云中的关键点,可以用于匹配和对齐。
3. 特征点匹配:使用OpenCVSharp的FLANN或Brute-Force算法对特征点进行匹配。匹配可以在两个点云之间建立对应关系。
4. 点云对齐:使用OpenCVSharp的ICP算法对点云进行对齐。ICP算法可以通过最小化点云之间的距离平方和来找到最佳的对齐变换。
5. 点云拼接:使用OpenCVSharp的点云拼接算法将对齐后的点云合并成一个大的点云。拼接后的点云可以保存为PCL格式或其他格式。
下面是一个简单的示例代码,演示如何使用OpenCVSharp进行点云拼图:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using OpenCvSharp;
using OpenCvSharp.PCL;
namespace PointCloudRegistration
{
class Program
{
static void Main(string[] args)
{
// 读取点云文件
PclCloud cloud1 = PclCloud.FromFile("cloud1.pcd");
PclCloud cloud2 = PclCloud.FromFile("cloud2.pcd");
// 提取特征点
var detector = new CvSURF(500);
KeyPoint[] keypoints1, keypoints2;
Mat descriptors1, descriptors2;
detector.DetectAndCompute(cloud1, null, out keypoints1, descriptors1);
detector.DetectAndCompute(cloud2, null, out keypoints2, descriptors2);
// 匹配特征点
var matcher = new FlannBasedMatcher();
var matches = matcher.Match(descriptors1, descriptors2);
// 对齐点云
var icp = new IterativeClosestPoint();
icp.SetInputSource(cloud1);
icp.SetInputTarget(cloud2);
icp.SetMaximumIterations(100);
icp.Align(cloud1);
// 拼接点云
var concat = new ConcatenateClouds();
var result = new PclCloud();
concat.Concatenate(cloud1, cloud2, result);
// 保存拼接后的点云
result.ToFile("result.pcd");
}
}
}
```
注意:上述代码仅作为示例,实际使用时需要根据具体的点云数据进行调整和优化。
相关推荐
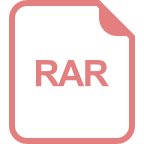
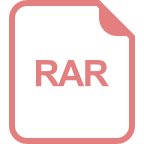














