编写程序实现将一个文件内容追加到另一个文件内容后,如将D盘file文件夹下的f1.txt追加到E盘根目录下的f2.txt中。(必须异常处理)
时间: 2024-03-13 08:43:01 浏览: 52
好的,以下是Python代码实现:
```python
import os
source_file_path = "D:/file/f1.txt"
target_file_path = "E:/f2.txt"
try:
with open(source_file_path, 'r') as source_file:
source_content = source_file.read()
with open(target_file_path, 'a') as target_file:
target_file.write(source_content)
print("文件内容追加成功!")
except Exception as e:
print("文件操作出现异常:", e)
```
解释一下代码的主要逻辑:
1. 定义源文件路径和目标文件路径。
2. 使用 `with open()` 打开源文件和目标文件,分别保存为 `source_file` 和 `target_file`。
3. 读取源文件内容为 `source_content`。
4. 将 `source_content` 写入目标文件。
5. 如果没有发生异常,则输出“文件内容追加成功!”。
需要注意的是,代码中使用了异常处理语句 `try...except`,能够在操作文件时捕获可能出现的异常,并输出异常信息。这样能够避免程序因为文件操作错误而崩溃。
相关问题
. 编写程序实现将一个文件内容追加到另一个文件内容后,如将D盘file文件夹下的f1.txt追加到E盘根目录下的f2.txt中。(必须异常处理)java
好的,我可以为你提供以下Java代码实现:
```java
import java.io.*;
public class AppendFile {
public static void main(String[] args) {
String sourceFilePath = "D:\\file\\f1.txt";
String targetFilePath = "E:\\f2.txt";
try {
File sourceFile = new File(sourceFilePath);
File targetFile = new File(targetFilePath);
if (!sourceFile.exists()) {
System.out.println("Source file does not exist!");
return;
}
if (!targetFile.exists()) {
targetFile.createNewFile();
}
FileWriter fw = new FileWriter(targetFile, true);
BufferedReader br = new BufferedReader(new FileReader(sourceFile));
String line = null;
while ((line = br.readLine()) != null) {
fw.write(line + "\n");
}
br.close();
fw.close();
System.out.println("File appended successfully!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
上述代码中,我们首先定义了源文件路径和目标文件路径,然后创建了源文件和目标文件的 File 对象。接下来,我们检查源文件是否存在,如果不存在则输出提示信息并退出程序;检查目标文件是否存在,如果不存在则创建该文件。
然后,我们使用 FileWriter 类和 BufferedReader 类分别打开目标文件和源文件,并将源文件内容逐行读取,并通过 FileWriter 将其写入目标文件中。
最后,我们关闭 BufferedReader 和 FileWriter,输出操作成功的提示信息。
在上述代码中,我们使用了 try-catch 块来处理可能发生的 IOException 异常。如果在文件操作中发生异常,程序将打印异常信息并结束运行。
Java编写程序实现将一个文件内容追加到另一个文件内容后,如将D盘file文件夹下的f1.txt追加到E盘根目录下的f2.txt中。(必须异常处理)
好的,以下是Java的示例代码:
```java
import java.io.*;
public class FileAppendDemo {
public static void main(String[] args) {
String sourceFilePath = "D:/file/f1.txt";
String targetFilePath = "E:/f2.txt";
try (BufferedReader reader = new BufferedReader(new FileReader(sourceFilePath));
BufferedWriter writer = new BufferedWriter(new FileWriter(targetFilePath, true))) {
String line;
while ((line = reader.readLine()) != null) {
writer.write(line);
writer.newLine();
}
System.out.println("文件追加成功!");
} catch (FileNotFoundException e) {
System.out.println("源文件不存在:" + e.getMessage());
} catch (IOException e) {
System.out.println("文件追加失败:" + e.getMessage());
}
}
}
```
这段代码使用了Java的文件操作功能,其中:
- `sourceFilePath` 是要追加的文件路径;
- `targetFilePath` 是目标文件路径,如果该文件不存在则会自动创建;
- `try (BufferedReader ...)` 是Java 7引入的Try-with-Resources语法,用于自动关闭资源;
- `BufferedReader` 和 `BufferedWriter` 是Java的缓冲读写类,可以提高文件读写效率;
- `while ((line = reader.readLine()) != null)` 是循环读取源文件的每一行内容,并将其写入目标文件中;
- 异常处理部分使用了Java的多个异常类型捕获,并将异常信息打印出来。
注意,使用此代码前请确保源文件和目标文件存在并且有相应的读写权限。
阅读全文
相关推荐
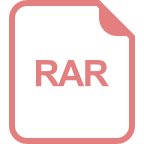
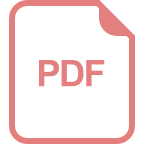



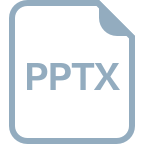
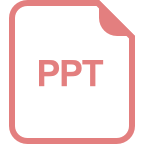
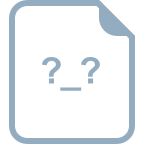
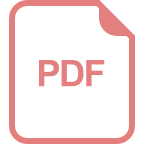
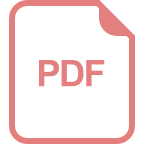
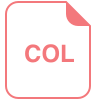
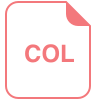
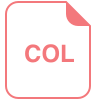
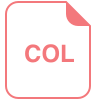