Implement fastest_words, which returns which words each player typed fastest. This function is called once both players have finished typing. It takes in a game. The game argument is a game data abstraction, like the one returned in Problem 9. You can access words in the game with selectors word_at, which takes in a game and the word_index (an integer). You can access the time it took any player to type any word using the time function provided in cats.py. The fastest_words function returns a list of lists of words, one list for each player, and within each list the words they typed the fastest. In the case of a tie, consider the earliest player in the list (the smallest player index) to be the one who typed it the fastest. Be sure to use the accessor functions for the game data abstraction, rather than assuming a particular data format.
时间: 2024-02-25 15:58:53 浏览: 32
Here's a possible implementation of the `fastest_words` function:
```python
from typing import List
from game import Game
from cats import time
def fastest_words(game: Game) -> List[List[str]]:
num_players = len(game.times)
num_words = len(game.words)
fastest = [[] for _ in range(num_players)]
for w in range(num_words):
fastest_time = float('inf')
fastest_players = []
for p in range(num_players):
t = time(game, p, w)
if t < fastest_time:
fastest_time = t
fastest_players = [p]
elif t == fastest_time:
fastest_players.append(p)
for p in fastest_players:
fastest[p].append(game.word_at(w))
return fastest
```
This function takes in a `Game` object as input, and returns a list of lists of words indicating which words each player typed fastest. The `Game` object has two attributes: `words` and `times`. `words` is a list of strings representing the words in the game. `times` is a list of lists representing the time it took each player to type each word.
To compute the fastest words, we iterate over each word in the game. For each word, we determine the fastest time among all players, as well as which players achieved that time. We then append the word to the list of fastest words for each of those players.
Note that in the case of a tie, we consider the earliest player in the list to be the one who typed it the fastest. This is done by always appending the word to the list of the first player in the `fastest_players` list.
Finally, we return the `fastest` list, which contains one list of words for each player indicating which words they typed fastest.
相关推荐
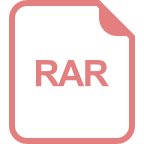
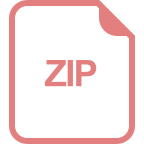












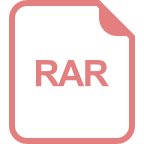