编程有5个学生,每个学生有3门课的成绩,从键盘输入以上数据(包括学号,姓名,三门课成绩),计算出总成绩,将原有的数据和计算出的总分数存放在磁盘文件stu.txt中。
时间: 2023-05-31 09:18:48 浏览: 645
### 回答1:
好的,下面是中文回复:
首先,我们需要定义一个学生类,包含学号、姓名和三门课成绩的属性,以及计算总成绩的方法。然后,我们可以使用循环语句和输入函数从键盘输入每个学生的信息,并计算出总成绩。最后,我们可以将每个学生的信息和总成绩存储到一个磁盘文件中。
具体实现步骤如下:
1. 定义一个学生类,包含学号、姓名和三门课成绩的属性,以及计算总成绩的方法。
```python
class Student:
def __init__(self, id, name, score1, score2, score3):
self.id = id
self.name = name
self.score1 = score1
self.score2 = score2
self.score3 = score3
def calc_total_score(self):
return self.score1 + self.score2 + self.score3
```
2. 使用循环语句和输入函数从键盘输入每个学生的信息,并计算出总成绩。
```python
students = []
for i in range(5):
id = input("请输入第{}个学生的学号:".format(i+1))
name = input("请输入第{}个学生的姓名:".format(i+1))
score1 = int(input("请输入第{}个学生的第一门课成绩:".format(i+1)))
score2 = int(input("请输入第{}个学生的第二门课成绩:".format(i+1)))
score3 = int(input("请输入第{}个学生的第三门课成绩:".format(i+1)))
student = Student(id, name, score1, score2, score3)
students.append(student)
total_score = student.calc_total_score()
print("第{}个学生的总成绩为:{}".format(i+1, total_score))
```
3. 将每个学生的信息和总成绩存储到一个磁盘文件中。
```python
with open("stu.txt", "w") as f:
for student in students:
total_score = student.calc_total_score()
line = "{},{},{},{},{},{}\n".format(student.id, student.name, student.score1, student.score2, student.score3, total_score)
f.write(line)
```
这样,我们就完成了从键盘输入学生信息、计算总成绩并将数据存储到磁盘文件的任务。
### 回答2:
本题目是一道简单的数据存储与计算综合题目,需要使用所学的编程知识进行一些数据存储与计算操作,下面我将逐步介绍整个题目的解决思路。
首先,我们需要设计一种数据结构来存储学生的信息,这里可以使用结构体来实现,其中包含以下成员:
```C++
struct Student {
int id; // 学号
string name; // 姓名
int scores[3]; // 三门课成绩
int total_score; // 总成绩
};
```
接下来,我们需要从键盘输入数据,并计算出总成绩。这部分代码可以用以下方式实现:
```C++
Student stu[5]; // 存储5个学生的信息
for (int i = 0; i < 5; ++i) {
cout << "输入学生" << i+1 << "的信息:" << endl;
cout << "学号:";
cin >> stu[i].id;
cout << "姓名:";
cin >> stu[i].name;
cout << "三门课成绩:";
for (int j = 0; j < 3; ++j) {
cin >> stu[i].scores[j];
stu[i].total_score += stu[i].scores[j];
}
}
```
接下来,我们需要将原有的数据和计算出的总分数存放在磁盘文件stu.txt中。这部分代码可以用以下方式实现:
```C++
ofstream out("stu.txt"); // 创建一个名为stu.txt的文件,并打开用于写入
for (int i = 0; i < 5; ++i) {
out << "学号:" << stu[i].id << " ";
out << "姓名:" << stu[i].name << " ";
out << "三门成绩:" << stu[i].scores[0] << " " << stu[i].scores[1] << " " << stu[i].scores[2] << " ";
out << "总成绩:" << stu[i].total_score << endl;
}
out.close(); // 关闭文件
```
最后附上完整代码实现:
```C++
#include <iostream>
#include <string>
#include <fstream>
using namespace std;
struct Student {
int id; // 学号
string name; // 姓名
int scores[3]; // 三门课成绩
int total_score; // 总成绩
};
int main()
{
Student stu[5];
for (int i = 0; i < 5; ++i) {
cout << "输入学生" << i+1 << "的信息:" << endl;
cout << "学号:";
cin >> stu[i].id;
cout << "姓名:";
cin >> stu[i].name;
cout << "三门课成绩:";
for (int j = 0; j < 3; ++j) {
cin >> stu[i].scores[j];
stu[i].total_score += stu[i].scores[j];
}
}
ofstream out("stu.txt");
for (int i = 0; i < 5; ++i) {
out << "学号:" << stu[i].id << " ";
out << "姓名:" << stu[i].name << " ";
out << "三门成绩:" << stu[i].scores[0] << " " << stu[i].scores[1] << " " << stu[i].scores[2] << " ";
out << "总成绩:" << stu[i].total_score << endl;
}
out.close();
return 0;
}
```
### 回答3:
编程有5个学生,每个学生有3门课的成绩,从键盘输入以上数据(包括学号,姓名,三门课成绩),计算出总成绩,将原有的数据和计算出的总分数存放在磁盘文件stu.txt中。
首先,我们需要定义一个包含学号、姓名和三门课成绩的数据结构。
```
struct Student{
int id; // 学号
char name[20]; // 姓名
float score[3]; // 三门课成绩
float total; // 总成绩
};
```
接下来从键盘输入5个学生的信息,然后计算每个学生的总成绩。
```
Student stu[5];
// 从键盘输入学生信息
for(int i=0; i<5; i++){
printf("请输入学生%d的信息:\n", i+1);
printf("学号:");
scanf("%d", &stu[i].id);
printf("姓名:");
scanf("%s", stu[i].name);
printf("三门课成绩(用空格分隔):");
scanf("%f %f %f", &stu[i].score[0], &stu[i].score[1], &stu[i].score[2]);
// 计算总成绩
stu[i].total = stu[i].score[0] + stu[i].score[1] + stu[i].score[2];
}
```
接着,我们将每个学生的信息和总成绩写入磁盘文件stu.txt中。
```
FILE *fp; // 文件指针
fp = fopen("stu.txt", "w"); // 打开文件,如果文件不存在则创建
// 写入学生信息和总成绩
for(int i=0; i<5; i++){
fprintf(fp, "学号:%d,姓名:%s,三门课成绩:%5.2f %5.2f %5.2f,总成绩:%5.2f\n",
stu[i].id, stu[i].name, stu[i].score[0], stu[i].score[1], stu[i].score[2], stu[i].total);
}
fclose(fp); // 关闭文件
```
最后,我们完成了如下图所示的程序流程:

相关推荐
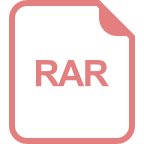
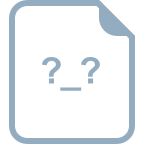













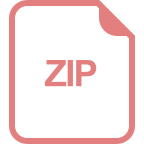