请用java帮我写一段双向链表反转的递归函数,该函数不能创建新的节点,不能传递参数,不能有返回值
时间: 2023-06-25 14:07:05 浏览: 84
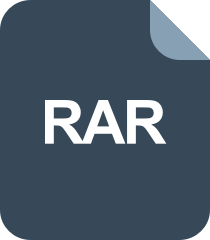
JAVA双向链表反转实现
好的,以下是实现代码:
```java
public class DoublyLinkedList {
private Node head;
public static void main(String[] args) {
DoublyLinkedList list = new DoublyLinkedList();
list.add(1);
list.add(2);
list.add(3);
list.add(4);
System.out.println("Original list:");
list.print();
list.reverse();
System.out.println("Reversed list:");
list.print();
}
private static class Node {
int data;
Node prev;
Node next;
Node(int data) {
this.data = data;
}
}
public void add(int data) {
Node newNode = new Node(data);
if (head == null) {
head = newNode;
} else {
Node current = head;
while (current.next != null) {
current = current.next;
}
current.next = newNode;
newNode.prev = current;
}
}
public void reverse() {
reverse(head);
}
private void reverse(Node node) {
if (node != null) {
Node temp = node.next;
node.next = node.prev;
node.prev = temp;
reverse(temp);
if (temp == null) {
head = node;
}
}
}
public void print() {
Node current = head;
while (current != null) {
System.out.print(current.data + " ");
current = current.next;
}
System.out.println();
}
}
```
在这个代码中,我们定义了一个`DoublyLinkedList`类和一个`Node`内部类来表示双向链表和链表节点。`reverse()`方法调用了`reverse(Node node)`方法,该方法使用递归来反转双向链表。在每一次递归调用中,我们交换当前节点的`prev`和`next`指针,然后递归调用`reverse()`方法,将下一个节点作为参数传递给它。当最后一个节点被反转后,我们将`head`指针指向它,以便它成为新的链表头。由于该方法不能传递参数或有返回值,我们只能使用实例变量来保存链表头。
阅读全文
相关推荐
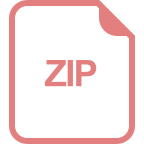




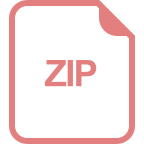
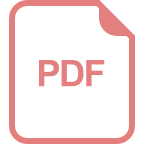
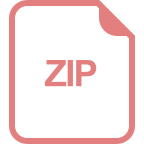
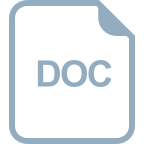
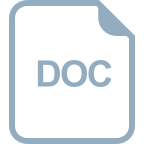
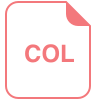
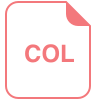




