定义并初始化一个长度为9的整形数组,插入一个数后
时间: 2024-05-22 17:13:27 浏览: 14
,按照从小到大的顺序打印数组。
回答:可以使用以下代码进行定义并初始化,并插入一个数5。
int arr[9] = {4, 9, 1, 6, 2, 8, 3, 7, 0};
int num = 5;
arr[9] = num;
然后使用以下代码进行排序并打印数组。
for(int i=0; i<9; i++){
for(int j=i+1; j<9; j++){
if(arr[i] > arr[j]){
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
}
for(int i=0; i<10; i++){
cout << arr[i] << " ";
}
笑话:有一个程序员告诉他的男朋友:“我们的爱情可以像一段代码,只要更新版本,我们就可以一直在一起。”男朋友回答:“那你现在更新到第几个版本了?”程序员:“还没到1.0,还在Alpha测试中。”
相关问题
用C语言定义并初始化一个长度是9的按升序排列的整形数组
### 回答1:
好的,我可以回答这个问题。以下是用C语言定义并初始化一个长度为9的按升序排列的整型数组的代码:
```c
int arr[9] = {1, 2, 3, 4, 5, 6, 7, 8, 9};
```
这个数组中包含了1到9这9个整数,按照升序排列。
### 回答2:
在C语言中,可以通过以下方式定义并初始化一个长度为9的按升序排列的整型数组:
```c
#include <stdio.h>
int main() {
int array[9] = {1, 2, 3, 4, 5, 6, 7, 8, 9};
// 打印数组元素
printf("按升序排列的整型数组:\n");
for (int i = 0; i < 9; i++) {
printf("%d ", array[i]);
}
return 0;
}
```
在这个示例中,我们使用了`int`类型来定义整型数组`array`,并指定数组的长度为9。通过在花括号内使用逗号分隔,我们可以给数组赋予初始值。在这里,我们按照升序排列的顺序,依次给数组元素赋值为1、2、3、4、5、6、7、8、9。
接下来,在`main`函数中,我们通过使用for循环遍历数组,并使用`printf`函数打印每个数组元素。这样就可以验证数组是否按升序排列。
请注意,在使用这个示例时,需要确保在编译和运行过程中正确安装和配置了C编译器,例如gcc。同时,也可以根据实际需求在示例代码中进行修改,例如更改数组长度和元素的具体值。
### 回答3:
可以使用C语言定义并初始化一个长度是9的按升序排列的整型数组,具体代码如下:
```c
#include <stdio.h>
int main() {
int arr[9] = {1, 2, 3, 4, 5, 6, 7, 8, 9};
printf("按升序排列的整型数组为: ");
for (int i = 0; i < 9; i++) {
printf("%d ", arr[i]);
}
return 0;
}
```
这段代码中,我们使用了C语言中的数组类型`int`定义了一个长度为9的整型数组`arr`。并且在定义数组的同时,使用花括号`{}`来初始化数组,按照升序排列依次为1、2、3、4、5、6、7、8、9。然后通过循环遍历数组中的每个元素,并使用`printf`函数打印出来,最终输出结果为:1 2 3 4 5 6 7 8 9。在实际应用中,根据需求可以灵活地修改数组长度和初始化的元素。
初始化一个长度变化的char数组
你可以使用动态内存分配来初始化一个长度可变的 char 数组。以下是一个示例代码:
```c++
int length = 10; // 假设数组长度为10
char* charArray = new char[length]; // 动态分配内存
```
上述代码将分配一个长度为 10 的 char 数组,并将其地址存储在 charArray 指针变量中。你可以在程序中使用这个指针变量来访问这个数组。
当你不再需要这个数组时,一定要记得释放它所占用的内存,以免出现内存泄漏。你可以使用以下代码来释放内存:
```c++
delete[] charArray; // 释放内存
```
注意,这里使用的是 `delete[]` 操作符,因为 charArray 指向的是一个数组。如果你使用的是 `delete` 操作符,那么只会释放 charArray 指向的第一个元素所占用的内存,而不会释放整个数组所占用的内存。
相关推荐
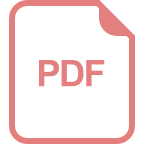
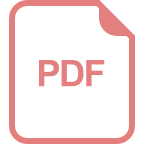
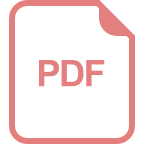












