用C语言编程将一个英文小写字母转换成大写字母输出。
时间: 2023-05-18 16:05:50 浏览: 70
可以使用C语言中的toupper()函数将小写字母转换成大写字母,示例代码如下:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char c = 'a';
char upper_c = toupper(c);
printf("%c\n", upper_c);
return 0;
}
```
输出结果为:
```
A
```
相关问题
c语言编程 编写一个函数,将大写英文字母转换为小写字母、小写字母转换为大写字母。
以下是一个将大写字母转换为小写字母、小写字母转换为大写字母的函数示例:
```c
#include <stdio.h>
#include <ctype.h>
void convert(char *str) {
while (*str != '\0') {
if (isupper(*str)) { // 判断是否为大写字母
*str = tolower(*str); // 转换为小写字母
} else if (islower(*str)) { // 判断是否为小写字母
*str = toupper(*str); // 转换为大写字母
}
str++; // 移动指针
}
}
int main() {
char str[100];
printf("请输入字符串:");
scanf("%s", str);
convert(str);
printf("转换后的字符串为:%s\n", str);
return 0;
}
```
在该示例中,我们使用了 `<ctype.h>` 库中的 `isupper`、`islower`、`tolower` 和 `toupper` 函数。其中,`isupper(c)` 用于判断字符 `c` 是否为大写字母,返回非零值表示是,否则返回零;`islower(c)` 用于判断字符 `c` 是否为小写字母,返回非零值表示是,否则返回零;`tolower(c)` 和 `toupper(c)` 分别用于将字符 `c` 转换为小写和大写字母,返回转换后的字符。
c语言编程:编写一个函数,将大写英文字母转换为小写字母、小写字母转换为大写字母。
可以使用C语言中的字符处理函数`isupper()`和`islower()`来判断字符是否为大写或小写字母,然后使用字符处理函数`toupper()`和`tolower()`进行大小写转换。下面是一个示例函数的实现:
```c
#include <ctype.h>
void convertCase(char *str) {
while (*str != '\0') {
if (isupper(*str)) {
*str = tolower(*str);
} else if (islower(*str)) {
*str = toupper(*str);
}
str++;
}
}
```
这个函数接受一个指向字符串的指针,并将其中的所有大写字母转换为小写字母,所有小写字母转换为大写字母。可以在主函数中调用该函数来进行测试:
```c
#include <stdio.h>
int main() {
char str[] = "Hello, World!";
printf("Original string: %s\n", str);
convertCase(str);
printf("After case conversion: %s\n", str);
return 0;
}
```
输出结果为:
```
Original string: Hello, World!
After case conversion: hELLO, wORLD!
```
相关推荐
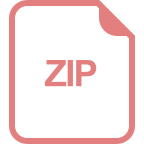












