c++判断三个string大小
时间: 2023-07-21 19:11:11 浏览: 44
可以使用 `>`、`<`、`==` 运算符对 `string` 类型进行比较,比较的结果是布尔类型的值,可以用 `if` 语句进行判断。具体实现代码如下:
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
string str1 = "hello";
string str2 = "world";
string str3 = "cpp";
if (str1 > str2 && str1 > str3) {
cout << "str1 is the largest" << endl;
} else if (str2 > str1 && str2 > str3) {
cout << "str2 is the largest" << endl;
} else if (str3 > str1 && str3 > str2) {
cout << "str3 is the largest" << endl;
} else {
cout << "The strings are equal" << endl;
}
return 0;
}
```
输出结果为:
```
str3 is the largest
```
相关问题
c++判断三个string大小并按从小到大顺序输出
可以使用 `std::sort` 函数进行排序,如下所示:
```c++
#include <iostream>
#include <algorithm>
#include <string>
int main() {
std::string s1 = "hello";
std::string s2 = "world";
std::string s3 = "c++";
std::string arr[] = {s1, s2, s3};
std::sort(arr, arr + 3);
for (int i = 0; i < 3; i++) {
std::cout << arr[i] << " ";
}
std::cout << std::endl;
return 0;
}
```
输出结果为:`c++ hello world`。
c++ string
引用:在C++中,可以使用string类来表示字符串。可以通过索引或at()函数来访问string字符串的元素。例如,字符串str中的第三个字符可以使用str或str.at(2)来获取。
引用:如果需要将数值转换为字符串,在C++中可以使用stringstream类来实现。首先创建一个stringstream对象,然后使用<<操作符将数值插入对象中。最后可以使用>>操作符或str()函数将stringstream对象转换为字符串。下面是一个示例代码:
```c++
#include <string>
#include <sstream>
#include <iostream>
using namespace std;
int main() {
double a = 123.32;
string res;
stringstream ss;
ss << a;
ss >> res; // 或者 res = ss.str();
cout << res;
return 0;
}
```
这段代码将数值a转换为字符串,并将其输出。
对于string类型的字符串,可以使用比较操作符来比较字符串的大小。与C语言中的字符串比较函数不同,C++中的string类可以直接使用>、<、==、>=等数学符号进行比较。例如,可以使用==操作符来判断两个字符串是否相等,或者使用>操作符来比较两个字符串的大小。下面是一个示例代码:
```c++
#include <string>
#include <iostream>
using namespace std;
int main() {
string a;
a = "hello";
if (a == "world")
cout << "yes" << endl;
else if (a > "world") {
cout << "You win." << endl;
} else
cout << "You failed." << endl;
a = " world";
cout << a << endl;
}
```
这段代码首先给字符串a赋值为"hello",然后使用==操作符判断a是否等于"world",如果不等于则继续使用>操作符判断a是否大于"world",如果大于则输出"You win.",否则输出"You failed."。最后将字符串a赋值为" world"并输出。
相关推荐
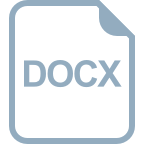
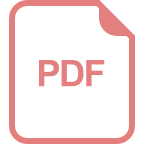
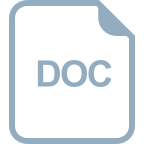












