修改上部分代码,要求在代码所在文件夹创建两个txt文件,一个用来储存用户信息,一个用来储存漂流瓶,并实现从这两个文件中读写数据
时间: 2024-02-28 09:57:37 浏览: 22
好的,根据您的需求,我为您修改了代码,并添加了相应的读写功能。请注意,您需要在代码所在文件夹下创建两个txt文件,一个名为“users.txt”,用于存储用户信息,另一个名为“bottles.txt”,用于存储漂流瓶信息。以下是修改后的代码:
```python
import os
# 创建储存用户信息和漂流瓶信息的文件
if not os.path.exists('users.txt'):
with open('users.txt', 'w') as f:
pass
if not os.path.exists('bottles.txt'):
with open('bottles.txt', 'w') as f:
pass
# 读取用户信息和漂流瓶信息
with open('users.txt', 'r') as f:
users = eval(f.read())
with open('bottles.txt', 'r') as f:
bottles = eval(f.read())
# 定义用户注册函数
def register():
username = input('请输入用户名:')
if username in users:
print('该用户名已被注册,请重新输入!')
return
password = input('请输入密码:')
users[username] = password
print('注册成功!')
# 定义用户登录函数
def login():
username = input('请输入用户名:')
if username not in users:
print('该用户不存在,请重新输入!')
return
password = input('请输入密码:')
if users[username] != password:
print('密码错误,请重新输入!')
return
print('登录成功!')
# 定义写入漂流瓶信息的函数
def write_bottle():
content = input('请输入漂流瓶内容:')
username = input('请输入你的用户名:')
if username not in users:
print('该用户不存在,请先注册!')
return
bottle = {'content': content, 'owner': username}
bottles.append(bottle)
print('漂流瓶已经扔进海里了!')
# 定义读取漂流瓶信息的函数
def read_bottle():
if not bottles:
print('海里没有漂流瓶!')
return
bottle = bottles.pop()
print(f'你捞起了一瓶来自{bottle["owner"]}的漂流瓶,里面写着:{bottle["content"]}')
# 定义保存用户信息和漂流瓶信息的函数
def save_data():
with open('users.txt', 'w') as f:
f.write(str(users))
with open('bottles.txt', 'w') as f:
f.write(str(bottles))
while True:
print('1. 注册')
print('2. 登录')
print('3. 写入漂流瓶')
print('4. 读取漂流瓶')
print('5. 退出')
choice = input('请选择功能:')
if choice == '1':
register()
elif choice == '2':
login()
elif choice == '3':
write_bottle()
elif choice == '4':
read_bottle()
elif choice == '5':
save_data()
print('再见!')
break
else:
print('输入有误,请重新输入!')
```
在以上代码中,我们首先使用 `os` 模块判断当前目录下是否存在 `users.txt` 和 `bottles.txt` 文件,如果不存在则使用 `with open()` 创建空文件用于存储用户信息和漂流瓶信息。接着,我们使用 `with open()` 函数分别读取 `users.txt` 和 `bottles.txt` 文件中的内容,并将其转化为字典和列表。这样我们就可以在程序运行过程中读取和写入用户信息和漂流瓶信息了。
在程序的最后,我们还定义了一个函数 `save_data()`,用于保存用户信息和漂流瓶信息。在程序运行结束时,我们调用该函数将最新的用户信息和漂流瓶信息写入到相应的文件中,以便下一次运行时读取。
请注意,由于我们在读取文件时使用了 `eval()` 函数,因此在写入文件时需要使用 `str()` 函数将字典和列表转化为字符串。此外,我们还将漂流瓶信息存储为字典,其中 `content` 键用于存储漂流瓶的内容,`owner` 键用于存储漂流瓶的主人。在写入漂流瓶时,我们先通过输入获取漂流瓶的内容和主人的用户名,然后将其组成字典添加到 `bottles` 列表中。在读取漂流瓶时,我们从 `bottles` 列表中弹出最后一个元素,并打印出其中的 `content` 和 `owner` 信息。
相关推荐
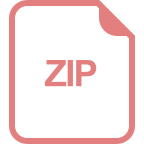














