调用数据库用户openid定时给用户发送订阅通知用php这么表示
时间: 2023-02-07 21:57:20 浏览: 118
下面是用 PHP 调用数据库并定时发送订阅通知给用户的基本方法:
1. 首先,连接到数据库,并选择要使用的数据库。
```
$dbc = mysqli_connect('host', 'username', 'password', 'database_name')
or die('Error connecting to MySQL server.');
```
2. 然后,使用 SQL 语句查询数据库中所有需要发送通知的用户的 openid。
```
$query = "SELECT openid FROM users WHERE subscription='1'";
$result = mysqli_query($dbc, $query)
or die('Error querying database.');
```
3. 循环遍历结果集,并使用每个 openid 发送通知。
```
while ($row = mysqli_fetch_array($result)) {
send_notification($row['openid']);
}
```
4. 最后,关闭数据库连接。
```
mysqli_close($dbc);
```
其中,send_notification 函数用于发送通知。这可能需要使用第三方 API 或其他工具。
相关问题
调用数据库用户openid定时给用户发送订阅模板通知用php怎么表示
你可以使用 PHP 的 cron 或者其他定时任务工具来实现定时发送订阅模板通知。
具体来说,你可以使用 PHP 的数据库扩展(例如 PDO 或 MySQLi)来连接到数据库,然后执行 SQL 查询来获取需要发送订阅模板通知的用户的 openid。
然后,你可以使用微信公众平台提供的接口来发送订阅模板消息。你需要在微信公众平台中配置好订阅模板,然后使用相应的 API 调用来向用户发送订阅模板通知。
总的来说,你需要使用 PHP 来完成以下几个步骤:
1. 连接到数据库并执行 SQL 查询来获取需要发送订阅模板通知的用户的 openid。
2. 调用微信公众平台的接口来发送订阅模板通知。
具体的代码实现细节可能会有所不同,但这是大致的流程。
调用数据库用户openid定时给用户发送订阅模板通知的php代码是什么
### 回答1:
首先,您需要在 PHP 中连接到数据库。这通常需要使用 MySQLi 或 PDO 扩展。例如,使用 MySQLi 扩展连接到数据库的代码如下:
```
$servername = "localhost";
$username = "username";
$password = "password";
$dbname = "myDB";
// 创建连接
$conn = mysqli_connect($servername, $username, $password, $dbname);
// 检测连接
if (!$conn) {
die("Connection failed: " . mysqli_connect_error());
}
```
然后,您可以使用 PHP 执行 SQL 查询,从数据库中选择所有需要发送订阅模板消息的用户的 openid。例如:
```
$sql = "SELECT openid FROM users WHERE send_subscription_notification = 1";
$result = mysqli_query($conn, $sql);
if (mysqli_num_rows($result) > 0) {
// 循环遍历每个用户
while($row = mysqli_fetch_assoc($result)) {
$openid = $row['openid'];
// 在这里调用微信 API 发送订阅模板消息
}
}
```
最后,您可以使用 PHP 的 cron 扩展或其他方法来实现定时任务。例如,每小时执行一次上述代码的 crontab 设置如下:
```
0 * * * * /usr/bin/php /path/to/send_subscription_notification.php
```
希望这能帮到您!
### 回答2:
实现定时给用户发送订阅模板通知的PHP代码需要以下步骤:
1. 首先,导入数据库连接的配置文件,并建立与数据库的连接。例如,使用mysqli连接MySQL数据库:
```php
<?php
$servername = "localhost";
$username = "your_username";
$password = "your_password";
$dbname = "your_database";
$conn = new mysqli($servername, $username, $password, $dbname);
if ($conn->connect_error) {
die("连接失败: " . $conn->connect_error);
}
?>
```
2. 查询要发送订阅模板通知的用户的openid。假设用户表为`users`,字段包括`openid`和其他相关信息,可以使用如下代码进行查询:
```php
<?php
$sql = "SELECT openid FROM users";
$result = $conn->query($sql);
if ($result->num_rows > 0) {
while ($row = $result->fetch_assoc()) {
$openid = $row['openid'];
// 在此处调用向用户发送订阅模板通知的函数
}
} else {
echo "没有查询到用户数据";
}
?>
```
3. 在查询结果循环中,可以调用相应的发送订阅模板通知的函数,可以使用微信提供的模板消息API来实现。例如,使用微信公众号的接口`subscribeMessage.send`来发送订阅模板消息:
```php
<?php
function sendSubscriptionMessage($openid, $content) {
$access_token = "your_access_token";
$template_id = "your_template_id";
$url = "https://api.weixin.qq.com/cgi-bin/message/subscribe/send?access_token=" . $access_token;
$data = array(
'touser' => $openid,
'template_id' => $template_id,
'data' => $content
);
$options = array(
'http' => array(
'method' => 'POST',
'content' => json_encode($data),
'header' => "Content-Type: application/json\n"
)
);
$context = stream_context_create($options);
$result = file_get_contents($url, false, $context);
// 处理发送结果
if ($result === false) {
// 发送失败的处理逻辑
} else {
// 发送成功的处理逻辑
}
}
?>
```
在调用`sendSubscriptionMessage`函数时,传入用户的openid和消息内容即可实现向用户发送订阅模板通知。
以上就是调用数据库用户openid定时给用户发送订阅模板通知的PHP代码的步骤和示例。请根据具体需求,进行相应的更改和扩展。
### 回答3:
调用数据库用户openid定时给用户发送订阅模板通知的PHP代码可以如下所示:
```php
<?php
// 设置时区为中国标准时间
date_default_timezone_set("Asia/Shanghai");
// 连接数据库
$servername = "localhost";
$username = "your_username";
$password = "your_password";
$dbname = "your_database_name";
$conn = new mysqli($servername, $username, $password, $dbname);
if ($conn->connect_error) {
die("连接数据库失败:" . $conn->connect_error);
}
// 获取当前时间
$current_time = date("Y-m-d H:i:s");
// 设置定时发送的时间(这里以每天早上9点为例)
$send_time = date("Y-m-d") . " 09:00:00";
// 查询当前时间之前的用户openid
$sql = "SELECT openid FROM users WHERE register_time <= '$current_time'";
$result = $conn->query($sql);
if ($result->num_rows > 0) {
while ($row = $result->fetch_assoc()) {
// 发送订阅模板通知
$openid = $row["openid"];
$template_id = "your_template_id";
$url = "your_template_url";
$data = array(
"first" => array("value" => "您好,这是每天给您发送的订阅模板通知", "color" => "#173177"),
"keyword1" => array("value" => "内容1", "color" => "#173177"),
"keyword2" => array("value" => "内容2", "color" => "#173177"),
// 添加更多关键字
"remark" => array("value" => "感谢您的订阅", "color" => "#173177")
);
$data_string = json_encode($data);
$post_data = array(
"touser" => $openid,
"template_id" => $template_id,
"url" => $url,
"data" => $data_string
);
$post_json = json_encode($post_data);
// 发送请求
$access_token = "your_access_token"; // 从微信公众平台获取的访问令牌
$url = "https://api.weixin.qq.com/cgi-bin/message/subscribe/send?access_token=" . $access_token;
$curl = curl_init();
curl_setopt($curl, CURLOPT_URL, $url);
curl_setopt($curl, CURLOPT_POST, 1);
curl_setopt($curl, CURLOPT_POSTFIELDS, $post_json);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($curl, CURLOPT_HEADER, 0);
curl_setopt($curl, CURLOPT_HTTPHEADER, array('Content-Type: application/json'));
$output = curl_exec($curl);
if (curl_errno($curl)) {
echo 'Error: ' . curl_error($curl);
}
curl_close($curl);
// 记录发送日志
$log_message = "发送给用户:" . $openid . ",发送时间:" . $current_time . ",发送结果:" . $output;
file_put_contents("log.txt", $log_message . PHP_EOL, FILE_APPEND);
}
} else {
echo "没有需要发送的用户。";
}
$conn->close();
?>
```
请根据实际情况将代码中的参数(如数据库连接信息、模板ID、访问令牌等)进行替换。代码的基本逻辑是:获取当前时间,连接数据库查询注册时间早于当前时间的用户openid,然后使用CURL发送订阅模板通知给每个用户,并记录发送日志。
阅读全文
相关推荐
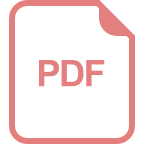
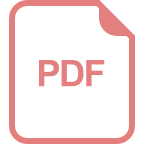
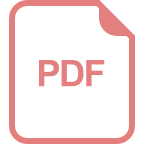







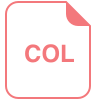
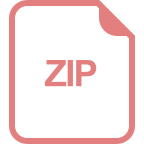
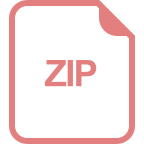
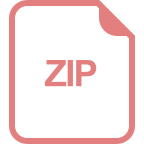
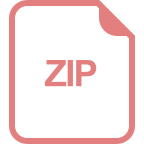
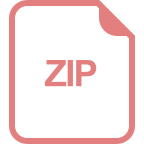
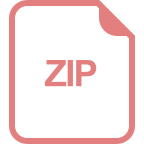
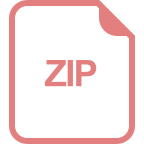