用java写一个根据目前时间结束时间为下午五点的桌面窗口显示时分秒并于倒计时结束时强制关闭计算机
时间: 2023-05-11 15:07:45 浏览: 146
以下是 Java 代码:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class CountdownTimer extends JFrame implements ActionListener {
private JLabel timeLabel;
private Timer timer;
public CountdownTimer() {
setTitle("倒计时");
setSize(300, 100);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
timeLabel = new JLabel();
timeLabel.setFont(new Font("宋体", Font.BOLD, 30));
timeLabel.setHorizontalAlignment(SwingConstants.CENTER);
add(timeLabel, BorderLayout.CENTER);
timer = new Timer(1000, this);
timer.start();
}
public void actionPerformed(ActionEvent e) {
long currentTime = System.currentTimeMillis();
long endTime = getEndTime(currentTime);
long remainingTime = endTime - currentTime;
if (remainingTime <= 0) {
timeLabel.setText("时间到了!");
timer.stop();
shutdown();
} else {
int hours = (int) (remainingTime / 3600000);
int minutes = (int) ((remainingTime % 3600000) / 60000);
int seconds = (int) ((remainingTime % 60000) / 1000);
timeLabel.setText(String.format("%02d:%02d:%02d", hours, minutes, seconds));
}
}
private long getEndTime(long currentTime) {
Calendar calendar = Calendar.getInstance();
calendar.setTimeInMillis(currentTime);
calendar.set(Calendar.HOUR_OF_DAY, 17);
calendar.set(Calendar.MINUTE, 0);
calendar.set(Calendar.SECOND, 0);
calendar.set(Calendar.MILLISECOND, 0);
if (calendar.getTimeInMillis() <= currentTime) {
calendar.add(Calendar.DAY_OF_MONTH, 1);
}
return calendar.getTimeInMillis();
}
private void shutdown() {
try {
Runtime.getRuntime().exec("shutdown -s -t 0");
} catch (Exception ex) {
ex.printStackTrace();
}
}
public static void main(String[] args) {
new CountdownTimer().setVisible(true);
}
}
```
这个程序会在窗口中显示当前距离下午五点还有多长时间,当时间到达下午五点时,会弹出一个对话框提示用户,并且强制关闭计算机。
阅读全文
相关推荐


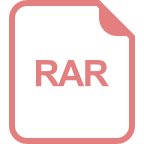










