题目内容: 从键盘输入一个字符串,统计字符串中小写字母的个数,要求统计小写字母的个数用自定义函数实现。 完成小写字母字符统计的自定义函数 int fact(char str[])的设计,提交代码时连同主函数一同提交。 主函数如下 #include<stdio.h> main() { int res; char s[50]; gets(s); res=fact(s); printf("%d",res); } 输入格式: 输入一个字符串(长度不超过50个字符) 输出格式: 以整型格式输出结果 输入样例: Hello World! 输出样例: 8
时间: 2024-02-02 10:05:03 浏览: 60
好的,这道题目要求我们统计字符串中小写字母的个数,并且需要自定义一个函数实现。具体思路如下:
1. 首先定义一个计数器 count,用于统计小写字母的个数。
2. 然后循环遍历字符串中的每一个字符,如果该字符是小写字母,则将计数器 count 加 1。
3. 最后返回计数器 count 的值即可。
根据上述思路,我们可以自定义一个函数 int fact(char str[]),用于统计字符串中小写字母的个数。具体实现如下:
```c
int fact(char str[]) {
int count = 0;
for (int i = 0; str[i] != '\0'; i++) {
if (str[i] >= 'a' && str[i] <= 'z') {
count++;
}
}
return count;
}
```
然后在主函数中读入字符串,调用自定义函数计算小写字母的个数,最后输出结果即可。完整代码如下:
```c
#include <stdio.h>
int fact(char str[]) {
int count = 0;
for (int i = 0; str[i] != '\0'; i++) {
if (str[i] >= 'a' && str[i] <= 'z') {
count++;
}
}
return count;
}
int main() {
int res;
char s[50];
gets(s);
res = fact(s);
printf("%d", res);
return 0;
}
```
输入格式:
输入一个字符串(长度不超过 50 个字符)
输出格式:
以整型格式输出结果
输入样例:
Hello World!
输出样例:
8
相关推荐
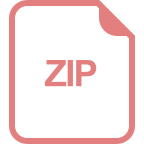














