python 遍历出 指定标题的 句柄 和其所有 子句柄和孙句柄 并提取出来
时间: 2024-03-14 21:47:18 浏览: 28
可以使用 Python 的 `pyautogui` 库和 `win32gui` 库来实现遍历窗口句柄和提取子句柄和孙句柄。
以下是一个示例代码,它可以遍历 Windows 窗口,找到指定标题的句柄,并提取出它的所有子句柄和孙句柄:
```python
import pyautogui
import win32gui
def get_hwnds_for_pid (pid):
def callback (hwnd, hwnds):
if win32gui.IsWindowVisible (hwnd) and win32gui.IsWindowEnabled (hwnd):
_, found_pid = win32gui.GetWindowThreadProcessId (hwnd)
if found_pid == pid:
hwnds.append (hwnd)
return True
hwnds = []
win32gui.EnumWindows (callback, hwnds)
return hwnds
def get_child_hwnds (hwnd):
child_hwnds = []
win32gui.EnumChildWindows (hwnd, lambda hwnd, param: param.append(hwnd), child_hwnds)
return child_hwnds
def get_descendant_hwnds (hwnd):
descendant_hwnds = []
def enum(hwnd, param):
param.append(hwnd)
win32gui.EnumChildWindows(hwnd, enum, param)
enum(hwnd, descendant_hwnds)
return descendant_hwnds
def find_window(title):
hwnds = get_hwnds_for_pid (win32gui.GetWindowThreadProcessId (win32gui.GetForegroundWindow ()))
for hwnd in hwnds:
if win32gui.GetWindowText(hwnd) == title:
return hwnd
return None
def extract_text(hwnd):
text = win32gui.GetWindowText(hwnd)
if text:
return text.strip()
else:
return ''
def extract_text_from_hwnds(hwnds):
texts = []
for hwnd in hwnds:
text = extract_text(hwnd)
if text:
texts.append(text)
return texts
# 示例使用方法
window_title = "指定标题"
window_hwnd = find_window(window_title)
if window_hwnd:
child_hwnds = get_child_hwnds(window_hwnd)
descendant_hwnds = get_descendant_hwnds(window_hwnd)
child_texts = extract_text_from_hwnds(child_hwnds)
descendant_texts = extract_text_from_hwnds(descendant_hwnds)
print("子句柄文本:", child_texts)
print("孙句柄文本:", descendant_texts)
else:
print("未找到窗口:", window_title)
```
你可以将代码中的 `window_title` 替换为你要查找的窗口标题,然后运行代码即可。
相关推荐
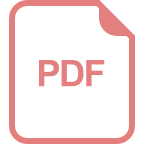
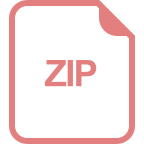
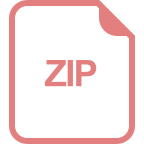














