多线程并发 cdemo
时间: 2023-05-14 14:01:07 浏览: 118
多线程并发是指在一个进程中同时执行多个线程,每个线程的执行流程相互独立但共享该进程的内存空间,从而使得系统可以在同一时间内完成多个任务,提高系统的并发能力和资源利用率。
在 C 语言中,可以使用 pthread 库来创建并管理线程。通过创建多个线程来同时执行多个任务,可以提高程序的效率和响应速度。在并发环境下,可能会涉及到共享数据的问题,需要使用临界区来保证在同一时间内只有一个线程能够访问共享数据,从而避免数据的不一致性和冲突问题。
同时,需要关注线程的安全性和效率。对于数据的读写操作,可以使用原子操作来保证数据的一致性;对于多个线程的同步和协作,可以使用信号量等同步机制。此外,一些优化技巧,如避免过度使用锁等,也可以提高程序的效率。
总之,多线程并发是一种高效的程序设计方法,可以提高系统的响应速度和资源利用率,但同时需要关注线程的安全性和效率,避免产生竞争和死锁等问题。
相关问题
openwrt linux 多线程并发 特定线程挂起和释放 帮忙写一个C demo
OpenWRT Linux 是一个针对嵌入式设备定制的轻量级Linux发行版,它支持多线程并发处理。在 C 语言中,我们可以使用 pthreads 库来进行线程操作,包括创建、管理和同步。下面是一个简单的 C 示例,展示了如何创建两个线程,其中一个线程会被主动挂起并最终释放:
```c
#include <stdio.h>
#include <pthread.h>
// 线程函数
void* thread_function(void* arg) {
printf("Thread %d is running.\n", *(int*)arg);
// 模拟工作循环,这里我们简单地让线程休眠一段时间
sleep(5);
printf("Thread %d finished.\n", *(int*)arg);
return NULL;
}
int main() {
pthread_t thread1, thread2; // 线程ID
int id1 = 1, id2 = 2;
// 创建两个线程
if (pthread_create(&thread1, NULL, thread_function, &id1)) {
perror("Error creating thread1");
exit(EXIT_FAILURE);
}
if (pthread_create(&thread2, NULL, thread_function, &id2)) {
perror("Error creating thread2");
exit(EXIT_FAILURE);
}
// 主线程暂停一段时间
printf("Main thread pausing...\n");
sleep(3); // 挂起主线程
// 撤销挂起线程1
pthread_cancel(thread1);
// 等待线程结束(取消线程会立即返回)
pthread_join(thread1, NULL);
// 继续运行线程2
printf("Resuming normal operation and joining thread2...\n");
pthread_join(thread2, NULL);
printf("Both threads have completed.\n");
return 0;
}
```
在这个示例中,`main` 函数首先创建了两个线程,然后主线程短暂休息。此时,通过 `pthread_cancel` 取消线程1使其挂起,接着主线程继续执行。最后,主线程等待两个线程都完成。
openwrt linux 多线程并发 采用signal的方式 特定线程挂起和释放 帮忙写一个C demo
OpenWRT Linux 是一个针对嵌入式设备的轻量级Linux发行版,它通常用于路由器和物联网设备。在OpenWRT上进行多线程并发操作并利用`signal`处理机制来控制特定线程的挂起和释放,你可以参考下面这个简单的C语言示例:
```c
#include <stdio.h>
#include <pthread.h>
#include <signal.h>
#define NUM_THREADS 5
#define THREAD_NAME_FORMAT "Thread %d"
// Signal handler function to be installed for our custom signal
void sig_handler(int signum) {
if (signum == SIGUSR1) { // Custom signal (e.g., SIGUSR1)
printf("Received signal, pausing thread...\n");
pthread_mutex_lock(&mutex); // Acquire the mutex to synchronize access
paused_threads++; // Increment the paused threads counter
if (paused_threads == NUM_THREADS) {
printf("All threads paused, releasing mutex and exiting...\n");
pthread_cond_signal(&cond); // Notify main thread to continue
}
pthread_mutex_unlock(&mutex);
} else {
printf("Unknown signal received.\n");
}
}
int main() {
pthread_t threads[NUM_THREADS];
int paused_threads = 0;
pthread_mutex_init(&mutex, NULL); // Initialize a mutex
pthread_cond_init(&cond, NULL); // Initialize a condition variable
// Install our custom signal handler
struct sigaction sa;
sa.sa_handler = sig_handler;
sigemptyset(&sa.sa_mask);
sa.sa_flags = 0;
sigaction(SIGUSR1, &sa, NULL);
for (int i = 0; i < NUM_THREADS; ++i) {
const char *thread_name = THREAD_NAME_FORMAT, *name = thread_name + strlen(thread_name) - 2;
pthread_create(&threads[i], NULL, worker_thread, (void*)name);
}
// Main loop: Wait for all threads to finish or a signal is caught
while (!all_threads_done()) {
pthread_cond_wait(&cond, &mutex); // Wait until signaled to continue
printf("Main thread checking status, %d of %d threads are running\n", running_threads, NUM_THREADS);
}
// Clean up resources
for (int i = 0; i < NUM_THREADS; ++i) {
pthread_join(threads[i], NULL);
}
pthread_mutex_destroy(&mutex);
pthread_cond_destroy(&cond);
return 0;
}
// Worker thread function that performs some work and listens for signals
void* worker_thread(void *arg) {
char *thread_name = arg;
printf("Starting %s...\n", thread_name);
while (1) {
// Simulate work
// ... [Your actual work here]
// Check if we should pause
signal(SIGUSR1, SIG_IGN); // Block SIGUSR1 temporarily
if (paused_threads > 0) {
printf("%s is paused.\n", thread_name);
pthread_mutex_lock(&mutex);
--paused_threads;
if (paused_threads == 0) {
printf("%s resumed after other threads.\n", thread_name);
signal(SIGUSR1, sig_handler); // Reactivate signal handling
pthread_mutex_unlock(&mutex);
}
} else {
// Normal work cycle
// ... [Continue your work]
}
}
}
// Helper functions to check thread statuses
bool all_threads_done() {
// Implement logic to check if all threads have finished their tasks
// This could involve checking thread join status or a shared flag
// For simplicity, let's assume it always returns false in this example
return false;
}
int running_threads = 0;
阅读全文
相关推荐
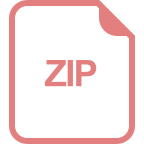

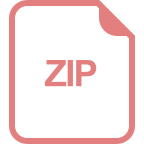
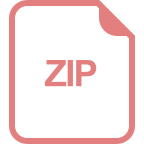
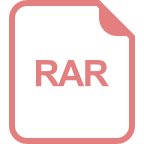
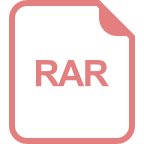
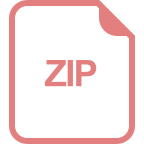
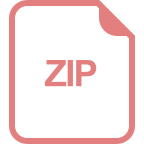
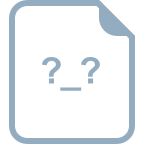
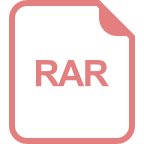
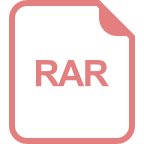
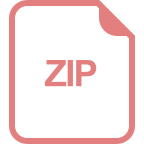
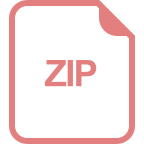