用Keras写一个non local block,用def方法写,不要class
时间: 2023-08-31 15:23:03 浏览: 149
### 回答1:
非局部块(Non-local Block)是一种用于计算机视觉中的注意力机制。在Keras中,可以使用Lambda层来实现非局部块。以下是一个使用Lambda层实现非局部块的示例代码:
```python
from keras.layers import *
def non_local_block(input_tensor, compression=2, mode='embedded'):
# 获取输入张量的通道数
channel = int(input_tensor.shape[-1])
if mode == 'gaussian':
# 计算高斯核矩阵
theta = Conv2D(channel // compression, 1)(input_tensor)
phi = Conv2D(channel // compression, 1)(input_tensor)
g = Conv2D(channel // compression, 1)(input_tensor)
theta = Reshape((-1, channel // compression))(theta)
phi = Reshape((-1, channel // compression))(phi)
g = Reshape((-1, channel // compression))(g)
theta_phi = Dot(axes=2)([theta, phi])
theta_phi = Activation('softmax')(theta_phi)
y = Dot(axes=2)([theta_phi, g])
y = Reshape((input_tensor.shape[1], input_tensor.shape[2], -1))(y)
y = Conv2D(channel, 1)(y)
else:
# 计算 embedded Gaussian 版本的高斯核矩阵
theta = Conv2D(channel // compression, 1)(input_tensor)
phi = Conv2D(channel // compression, 1)(input_tensor)
g = Conv2D(channel // compression, 1)(input_tensor)
theta_phi = Dot(axes=2)([theta, phi])
theta_phi = Activation('softmax')(theta_phi)
y = Dot(axes=2)([theta_phi, g])
y = Conv2D(channel, 1)(y)
# 添加残差连接
output_tensor = Add()([input_tensor, y])
return output_tensor
```
上述代码中,`non_local_block`函数接受一个输入张量`input_tensor`,以及两个可选参数`compression`和`mode`。`compression`参数用于控制非局部块中每个子通道的压缩比例,`mode`参数用于选择非局部块的实现方式,可以是`'embedded'`或`'gaussian'`。
函数首先获取输入张量的通道数,并根据所选的实现方式计算高斯核矩阵。然后,使用这个高斯核矩阵来计算非局部块的输出张量。最后,通过添加残差连接将输出张量与输入张量相加,得到最终的输出。
使用示例:
```python
from keras.models import Model
from keras.layers import Input
input_tensor = Input(shape=(224, 224, 64))
output_tensor = non_local_block(input_tensor)
model = Model(inputs=input_tensor, outputs=output_tensor)
model.summary()
```
### 回答2:
在Keras中编写一个non-local block可以通过def方法实现,而不使用class方法。non-local block是一种神经网络模块,用于捕捉远距离的依赖关系,用于图像或视频处理任务。
使用Keras的def方法来编写non-local block,需要创建一个包含所有在该block中使用的层的函数。
以下是一个用300字中文回答的non-local block的Keras def方法实现的示例:
```python
import tensorflow as tf
from tensorflow import keras
from tensorflow.keras import layers
def non_local_block(input_tensor):
# 获取input_tensor的尺寸
batch_size, height, width, channels = input_tensor.shape
# 首先,计算查询(query)、键(key)和值(value)
query = layers.Conv2D(channels // 2, kernel_size=1)(input_tensor)
key = layers.Conv2D(channels // 2, kernel_size=1)(input_tensor)
value = layers.Conv2D(channels, kernel_size=1)(input_tensor)
# 计算非局部块的内积,将查询(query)乘以键(key)的转置,然后进行softmax归一化
query_key = tf.matmul(query, tf.transpose(key, [0, 3, 1, 2]))
query_key = keras.activations.softmax(query_key, axis=-1)
# 将softmax归一化的结果乘以值(value)以获得最终的non-local特征表示
output = tf.matmul(query_key, value)
# 对结果进行调整以适应输入的尺寸,然后添加一个残差连接
output = layers.Reshape((height, width, channels))(output)
output = layers.Conv2D(channels, kernel_size=1)(output)
output += input_tensor
return output
```
在此代码中,我们首先使用卷积层计算出查询(query)、键(key)和值(value),然后计算非局部块的内积。通过将查询乘以键的转置和进行softmax归一化,我们可以获得非局部块的特征表示。最后,我们将结果调整为输入的尺寸,并添加一个残差连接。
通过调用non_local_block函数,并将输入张量传递给它,我们就可以在我们的模型中使用non-local block了。例如,我们可以像这样使用non-local block:
```python
input_tensor = layers.Input(shape=(height, width, channels))
output = non_local_block(input_tensor)
```
这是一个简单的例子,说明了如何使用Keras的def方法编写一个non-local block,以捕捉远距离的依赖关系。你可以根据需要对代码进行调整和改进。
阅读全文
相关推荐


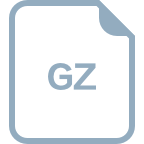




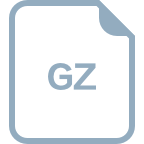

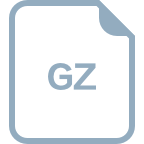

