vue2+vite父组件怎么调用子组件中的uni-popup
时间: 2023-09-11 14:05:37 浏览: 123
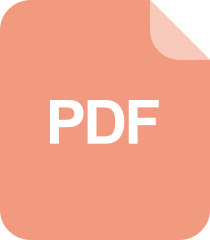
Vue父组件调用子组件事件方法
### 回答1:
如果你的子组件中的 `uni-popup` 是通过 `ref` 来获取的,那么你可以在父组件中通过 `$refs` 来访问该子组件的 `uni-popup`。具体的做法如下:
1. 在子组件中给 `uni-popup` 添加 `ref` 属性:
```html
<template>
<div>
<uni-popup ref="popup"></uni-popup>
</div>
</template>
```
2. 在父组件中通过 `$refs` 访问 `uni-popup`:
```html
<template>
<div>
<my-child ref="child"></my-child>
<button @click="showPopup">显示弹窗</button>
</div>
</template>
<script>
import MyChild from './MyChild.vue'
export default {
components: {
MyChild
},
methods: {
showPopup() {
// 调用子组件中的 uni-popup 的 show 方法
this.$refs.child.$refs.popup.show()
}
}
}
</script>
```
在上面的代码中,我们在父组件中通过 `ref` 给子组件添加了 `ref` 属性,然后通过 `$refs` 访问子组件,并访问子组件中的 `uni-popup`。最后调用 `uni-popup` 的 `show` 方法显示弹窗。需要注意的是,在访问子组件中的 `uni-popup` 时,需要使用 `$refs` 来访问。
### 回答2:
在Vue 2中,可以使用ref来获取子组件的引用,并通过该引用来调用子组件中的方法或属性。但在Vue 2中,由于Vite的单文件组件(SFC)的更新机制,我们不能直接使用传统的ref方法来获取子组件的引用。
在使用Vite中,我们可以通过使用特殊的导出方式来传递子组件的引用给父组件,并在父组件中使用该引用来调用子组件中的方法或属性。首先,在子组件的模板中,我们可以使用特殊的导出方式将子组件的引用导出给父组件。
```vue
<template>
<div>
<uni-popup ref="popupRef"></uni-popup>
</div>
</template>
<script>
export default {
name: 'ChildComponent',
mounted() {
this.$emit('popupRef', this.$refs.popupRef);
}
}
</script>
```
然后,在父组件中,我们可以使用v-on指令来接收子组件传递过来的引用,并将其保存在父组件的data中。
```vue
<template>
<div>
<child-component @popupRef="setPopupRef"></child-component>
<button @click="callPopupMethod">调用子组件的方法</button>
</div>
</template>
<script>
export default {
name: 'ParentComponent',
data() {
return {
popupRef: null
};
},
methods: {
setPopupRef(ref) {
this.popupRef = ref;
},
callPopupMethod() {
if (this.popupRef) {
this.popupRef.openPopup();
}
}
}
}
</script>
```
在上述代码中,父组件`ParentComponent`将通过`@popupRef`事件接收到子组件`ChildComponent`传递过来的引用,并将其保存在`popupRef`变量中。当点击按钮时,父组件调用`callPopupMethod`方法,该方法会判断`popupRef`是否存在,如果存在,则调用子组件的`openPopup`方法。
通过以上方法,我们可以在Vue 2的Vite项目中,实现父组件调用子组件中的`uni-popup`组件的功能。注意在Vite项目中,需要确保已经正确安装了uni-popup组件,并已经在父组件中正确引入和注册相关组件。
### 回答3:
在Vue2中,父组件调用子组件中的`uni-popup`可以通过`ref`引用子组件,并通过`$refs`来访问子组件的方法。具体步骤如下:
1. 在父组件模板中,使用`ref`指令给子组件添加一个引用名称,例如`<child-component ref="child1"></child-component>`。
2. 在父组件的方法中,通过`$refs`来获取子组件的引用,并调用子组件中的方法。例如,在点击事件中调用子组件的`uni-popup`方法,可以使用`this.$refs.child1.$refs.popup.open()`。
完整代码示例:
```html
<template>
<div>
<!-- 父组件模板 -->
<child-component ref="child1"></child-component>
<button @click="openPopup">打开弹窗</button>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent
},
methods: {
openPopup() {
this.$refs.child1.$refs.popup.open(); // 调用子组件中的uni-popup方法
}
}
}
</script>
```
在这个例子中,父组件通过`this.$refs.child1`访问到了子组件的引用,然后再通过`.$refs.popup`访问子组件中名为`popup`的引用,最后再调用`open()`方法来打开弹窗。
需要注意的是,以上操作需要在子组件的生命周期钩子函数中使用`$nextTick`来确保DOM已渲染完毕,例如:
```html
<template>
<div>
<uni-popup ref="popup">
<!-- 弹窗内容 -->
</uni-popup>
</div>
</template>
<script>
export default {
mounted() {
this.$nextTick(() => {
// 初始化uni-popup组件
});
},
methods: {
open() {
// 打开弹窗的方法
}
}
}
</script>
```
通过以上步骤,就可以在父组件中调用子组件中的`uni-popup`组件了。
阅读全文
相关推荐
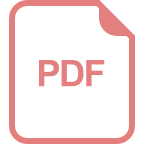
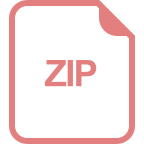
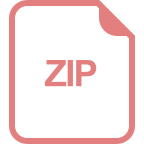
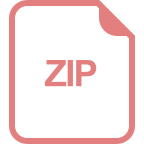
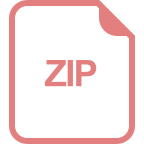
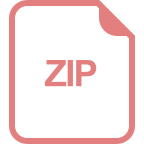
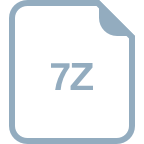
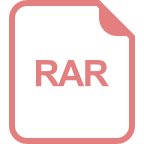
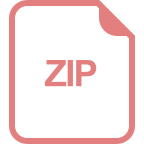
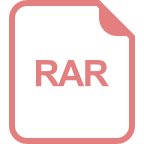
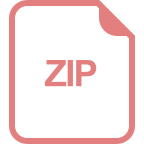
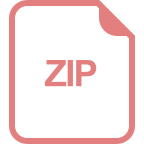
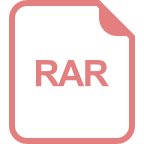
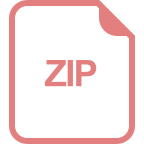
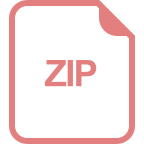
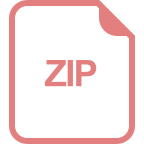
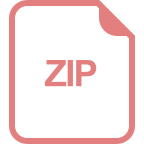
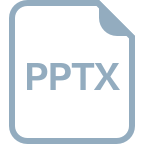