c++语言中编写,读取几个字符串,以空格区分,存入字符串数组,遇到换行符停止
时间: 2024-05-11 22:15:06 浏览: 135
可以使用 scanf 函数读取并分隔字符串,存入字符串数组中。具体代码如下:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[100];
char strs[10][100];
int count = 0;
printf("请输入字符串(以换行符结束):\n");
while (fgets(str, sizeof(str), stdin)) {
str[strcspn(str, "\n")] = '\0';
// 如果输入的是空行,停止输入
if (strlen(str) == 0) {
break;
}
// 将读取的字符串存入字符串数组中
sscanf(str, "%s", strs[count++]);
}
// 输出存储的字符串
printf("输入的字符串为:\n");
for (int i = 0; i < count; i++) {
printf("%d: %s\n", i + 1, strs[i]);
}
return 0;
}
```
当然,也可以使用 getchar 函数逐个字符读取并分隔字符串,存入字符串数组中。具体代码如下:
```c
#include <stdio.h>
#include <string.h>
int main() {
char ch;
char str[100];
char strs[10][100];
int count = 0;
printf("请输入字符串(以换行符结束):\n");
while ((ch = getchar()) != EOF) {
if (ch == '\n') {
// 如果输入的是空行,停止输入
if (strlen(str) == 0) {
break;
}
// 将读取的字符串存入字符串数组中
sscanf(str, "%s", strs[count++]);
memset(str, 0, sizeof(str)); // 清空字符串缓冲区
} else if (ch == ' ') {
// 将读取的字符串存入字符串数组中
sscanf(str, "%s", strs[count++]);
memset(str, 0, sizeof(str)); // 清空字符串缓冲区
} else {
str[strlen(str)] = ch;
}
}
// 输出存储的字符串
printf("输入的字符串为:\n");
for (int i = 0; i < count; i++) {
printf("%d: %s\n", i + 1, strs[i]);
}
return 0;
}
```
阅读全文
相关推荐




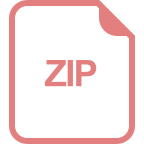













