Java实现图书管理系统mysql
时间: 2023-06-29 16:06:13 浏览: 48
实现一个图书管理系统需要以下几个步骤:
1. 设计数据库:使用MySQL数据库,设计图书信息表(book)、读者信息表(reader)、借阅记录表(borrow)等表格,并且建立相应的关系。
2. Java连接数据库:使用JDBC技术,连接MySQL数据库,实现对数据库的增删改查操作。
3. 实现图书管理系统功能:根据需求,实现图书借阅、归还、查询、添加、修改和删除等功能。
以下是一个简单的Java实现图书管理系统的示例代码:
```java
import java.sql.*;
import java.util.Scanner;
public class LibrarySystem {
static final String JDBC_DRIVER = "com.mysql.jdbc.Driver";
static final String DB_URL = "jdbc:mysql://localhost:3306/library";
static final String USER = "root";
static final String PASS = "password";
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
try {
Class.forName(JDBC_DRIVER);
conn = DriverManager.getConnection(DB_URL, USER, PASS);
stmt = conn.createStatement();
Scanner input = new Scanner(System.in);
while (true) {
System.out.println("1.添加图书");
System.out.println("2.删除图书");
System.out.println("3.修改图书");
System.out.println("4.查询图书");
System.out.println("5.借阅图书");
System.out.println("6.归还图书");
System.out.println("0.退出系统");
System.out.print("请输入菜单编号:");
int choice = input.nextInt();
switch (choice) {
case 1:
addBook(stmt, input);
break;
case 2:
deleteBook(stmt, input);
break;
case 3:
updateBook(stmt, input);
break;
case 4:
queryBook(stmt, input);
break;
case 5:
borrowBook(stmt, input);
break;
case 6:
returnBook(stmt, input);
break;
case 0:
System.out.println("退出系统!");
System.exit(0);
break;
default:
System.out.println("输入有误,请重新输入!");
break;
}
}
} catch (SQLException se) {
se.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (stmt != null) {
stmt.close();
}
} catch (SQLException se2) {
}
try {
if (conn != null) {
conn.close();
}
} catch (SQLException se) {
se.printStackTrace();
}
}
}
// 添加图书
public static void addBook(Statement stmt, Scanner input) throws Exception {
System.out.print("请输入图书编号:");
int id = input.nextInt();
System.out.print("请输入图书名称:");
String name = input.next();
System.out.print("请输入图书作者:");
String author = input.next();
System.out.print("请输入图书价格:");
double price = input.nextDouble();
String sql = "INSERT INTO book VALUES (" + id + ", '" + name + "', '" + author + "', " + price + ")";
int count = stmt.executeUpdate(sql);
if (count > 0) {
System.out.println("添加成功!");
} else {
System.out.println("添加失败!");
}
}
// 删除图书
public static void deleteBook(Statement stmt, Scanner input) throws Exception {
System.out.print("请输入要删除的图书编号:");
int id = input.nextInt();
String sql = "DELETE FROM book WHERE id=" + id;
int count = stmt.executeUpdate(sql);
if (count > 0) {
System.out.println("删除成功!");
} else {
System.out.println("删除失败!");
}
}
// 修改图书
public static void updateBook(Statement stmt, Scanner input) throws Exception {
System.out.print("请输入要修改的图书编号:");
int id = input.nextInt();
System.out.print("请输入图书名称:");
String name = input.next();
System.out.print("请输入图书作者:");
String author = input.next();
System.out.print("请输入图书价格:");
double price = input.nextDouble();
String sql = "UPDATE book SET name='" + name + "', author='" + author + "', price=" + price + " WHERE id=" + id;
int count = stmt.executeUpdate(sql);
if (count > 0) {
System.out.println("修改成功!");
} else {
System.out.println("修改失败!");
}
}
// 查询图书
public static void queryBook(Statement stmt, Scanner input) throws Exception {
System.out.print("请输入要查询的图书名称:");
String name = input.next();
String sql = "SELECT * FROM book WHERE name='" + name + "'";
ResultSet rs = stmt.executeQuery(sql);
while (rs.next()) {
int id = rs.getInt("id");
String bookName = rs.getString("name");
String author = rs.getString("author");
double price = rs.getDouble("price");
System.out.println("编号:" + id + " 书名:" + bookName + " 作者:" + author + " 价格:" + price);
}
rs.close();
}
// 借阅图书
public static void borrowBook(Statement stmt, Scanner input) throws Exception {
System.out.print("请输入借阅人姓名:");
String name = input.next();
System.out.print("请输入要借阅的图书编号:");
int bookId = input.nextInt();
String sql = "INSERT INTO borrow (reader_name, book_id, borrow_time) VALUES ('" + name + "', " + bookId + ", NOW())";
int count = stmt.executeUpdate(sql);
if (count > 0) {
System.out.println("借阅成功!");
} else {
System.out.println("借阅失败!");
}
}
// 归还图书
public static void returnBook(Statement stmt, Scanner input) throws Exception {
System.out.print("请输入归还人姓名:");
String name = input.next();
System.out.print("请输入要归还的图书编号:");
int bookId = input.nextInt();
String sql = "UPDATE borrow SET return_time=NOW() WHERE reader_name='" + name + "' AND book_id=" + bookId;
int count = stmt.executeUpdate(sql);
if (count > 0) {
System.out.println("归还成功!");
} else {
System.out.println("归还失败!");
}
}
}
```
注意:以上示例代码仅供参考,实际开发中需要考虑更多的细节问题。
相关推荐
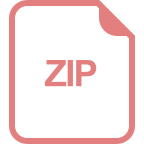
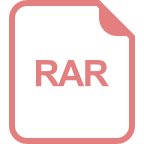
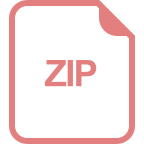










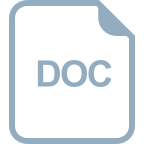
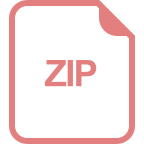