使用springboot写一个文件上传的代码
时间: 2024-02-13 12:59:52 浏览: 23
好的,以下是使用Spring Boot实现文件上传的示例代码:
1. 首先,创建一个控制器类,如下所示:
```java
@RestController
@RequestMapping("/api")
public class FileUploadController {
@PostMapping("/upload")
public ResponseEntity<String> handleFileUpload(@RequestParam("file") MultipartFile file) {
String message = "";
try {
// 保存文件到本地
Path path = Paths.get("uploads/" + file.getOriginalFilename());
Files.write(path, file.getBytes());
message = "Uploaded the file successfully: " + file.getOriginalFilename();
return ResponseEntity.status(HttpStatus.OK).body(message);
} catch (IOException e) {
message = "Could not upload the file: " + file.getOriginalFilename() + "!";
return ResponseEntity.status(HttpStatus.EXPECTATION_FAILED).body(message);
}
}
}
```
2. 在上面的控制器类中,我们使用了`@RequestParam`注解来接收文件上传请求中的文件。我们将文件保存到本地文件系统中的uploads目录下。
3. 接下来,我们需要配置`multipartResolver`来支持文件上传功能。在`application.properties`文件中添加以下配置:
```
spring.servlet.multipart.enabled=true
spring.servlet.multipart.file-size-threshold=2KB
spring.servlet.multipart.max-file-size=200MB
spring.servlet.multipart.max-request-size=215MB
```
4. 创建一个HTML表单来测试文件上传功能。可以使用以下代码示例:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>File Upload Example</title>
</head>
<body>
<h3>Upload a file:</h3>
<form method="POST" enctype="multipart/form-data" action="/api/upload">
<input type="file" name="file">
<br/><br/>
<input type="submit" value="Upload">
</form>
</body>
</html>
```
5. 启动应用程序并在浏览器中打开`http://localhost:8080`,上传文件并查看控制台输出,以确保文件已成功上传。
以上就是使用Spring Boot实现文件上传的示例代码。希望能对你有所帮助!
相关推荐
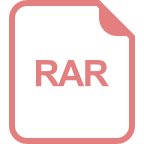
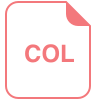
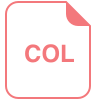
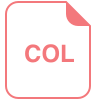
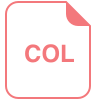
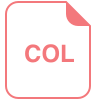









