java计算结果用百分数表示
时间: 2024-02-24 17:56:58 浏览: 43
要将Java计算结果用百分数表示,可以使用NumberFormat类的getPercentInstance方法。例如,假设计算结果为0.75,使用以下代码将其用百分数表示:
```
double result = 0.75;
NumberFormat percentFormat = NumberFormat.getPercentInstance();
String resultAsPercent = percentFormat.format(result);
System.out.println(resultAsPercent); // 输出:75%
```
这样就可以将计算结果以百分数的形式输出。注意,NumberFormat类的getPercentInstance方法返回的是一个NumberFormat对象,需要将其格式化为字符串后才能输出。
相关问题
sql计算结果换成百分数
要将SQL查询结果转换为百分比,可以使用SQL中的数值函数和格式化函数来实现。以下是一个示例:
```
SELECT column1, column2,
(COUNT(*) * 100.0 / SUM(COUNT(*)) OVER ()) AS percentage
FROM your_table
GROUP BY column1, column2;
```
在这个示例中,假设你已经按照column1和column2进行了分组聚合,并使用COUNT(*)计算了每个组的行数。现在,你想将这个计数转换为百分比。
使用SUM(COUNT(*)) OVER ()可以计算出总行数,然后通过将COUNT(*)乘以100.0并除以总行数来得到百分比。最后,将这个计算结果作为"percentage"列返回。
需要注意的是,根据你的数据库,可能需要使用不同的格式化函数来将百分比显示为带有百分号的字符串。例如,在MySQL中,可以使用CONCAT和FORMAT函数来实现:
```
SELECT column1, column2,
CONCAT(FORMAT((COUNT(*) * 100.0 / SUM(COUNT(*)) OVER ()), 2), '%') AS percentage
FROM your_table
GROUP BY column1, column2;
```
上述示例中的FORMAT函数将百分比计算结果保留两位小数,并使用CONCAT函数将结果与百分号连接起来。
请根据你所使用的数据库和具体需求进行调整。
plt.plot 纵坐标用百分数表示
在使用plt.plot绘制图形时,如果想要纵坐标以百分数的形式表示,可以使用matplotlib.ticker模块中的FormatStrFormatter或FuncFormatter函数来实现。引用[1]中的示例代码展示了使用FormatStrFormatter函数的方法,可以通过设置axes.yaxis.set_major_formatter(FormatStrFormatter('%.2f%%'))来将纵坐标格式化为百分数,并指定小数位数。引用[2]中的示例代码展示了使用FuncFormatter函数的方法,可以通过设置axes.yaxis.set_major_formatter(FuncFormatter(lambda x,_:'{}%'.format(x*100)))来将纵坐标格式化为百分数。这两种方法都可以实现纵坐标以百分数的形式显示。
相关推荐
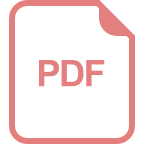












