ts declare
时间: 2023-09-12 21:11:08 浏览: 71
在 TypeScript 中,`declare` 关键字用于告诉编译器某个标识符的类型信息,而不需要实际的实现代码。它可以用于声明全局变量、函数、类、接口等。
例如,你可以使用 `declare` 声明一个全局变量:
```typescript
declare const myVar: number;
```
这样就告诉编译器 `myVar` 是一个全局变量,类型为 `number`,但是并没有提供具体的实现。
`declare` 也可以用于声明全局函数:
```typescript
declare function myGlobalFunction(): void;
```
这样就告诉编译器存在一个名为 `myGlobalFunction` 的全局函数,但是同样没有提供具体的实现。
需要注意的是,`declare` 只用于编译时的类型检查,并不会生成真正的 JavaScript 代码。这意味着在使用 `declare` 声明全局变量或函数时,你需要确保它们在运行时的环境中是可用的。
相关问题
ts declare使用
In TypeScript, the `declare` keyword is used to tell the compiler that a variable, function, class, or namespace already exists and is defined elsewhere. This is useful when you want to use a library or framework that was written in JavaScript, but you want to write your code in TypeScript.
Here are a few examples of how to use `declare` in TypeScript:
1. Declare a global variable:
```
declare const myVar: string;
```
This tells TypeScript that `myVar` is a global variable of type `string`, but the variable is not defined in the current file.
2. Declare a function:
```
declare function myFunc(param: string): number;
```
This tells TypeScript that `myFunc` is a function that takes a parameter of type `string` and returns a value of type `number`, but the function is not defined in the current file.
3. Declare a class:
```
declare class MyClass {
constructor(param: string);
myMethod(): void;
}
```
This tells TypeScript that `MyClass` is a class with a constructor that takes a parameter of type `string`, and the class has a method called `myMethod` that returns `void`, but the class is not defined in the current file.
4. Declare a namespace:
```
declare namespace MyNamespace {
const myVar: string;
function myFunc(param: string): number;
class MyClass {
constructor(param: string);
myMethod(): void;
}
}
```
This tells TypeScript that `MyNamespace` is a namespace that contains a variable called `myVar`, a function called `myFunc`, and a class called `MyClass`, but the namespace is not defined in the current file.
Using `declare` in TypeScript is a powerful tool for working with existing JavaScript code and libraries.
ts declare详解
TypeScript 中的 declare 关键字用于声明某些变量、函数或类的类型,但并不实际定义它们的实现。这是因为 TypeScript 可以用来编写 JavaScript,而 JavaScript 中的许多变量、函数或类并没有明确定义它们的类型。因此,使用 declare 关键字可以帮助 TypeScript 理解这些 JavaScript 代码的类型。
declare 关键字的使用有以下几种情况:
1. 声明全局变量或函数
在 TypeScript 中,全局变量或函数应该在任何文件中都可以访问。但如果这些变量或函数没有明确定义类型,则 TypeScript 编译器可能无法正确解析它们的类型。因此,使用 declare 关键字可以告诉编译器这些变量或函数的类型。
例如,以下代码声明了一个全局变量 message,并指定它的类型为字符串:
```
declare var message: string;
```
2. 声明模块
在 TypeScript 中,可以使用 declare 关键字来声明模块的类型。例如,以下代码声明了一个名为 MyModule 的模块,并指定它包含一个名为 myFunction 的函数:
```
declare module MyModule {
function myFunction(): void;
}
```
3. 声明类的类型
在 TypeScript 中,可以使用 declare 关键字来声明一个类的类型,而不实际定义它的实现。这通常用于与第三方库集成时,以便编译器知道这些类的类型。
例如,以下代码声明了一个名为 MyLibrary 的类,并指定它包含一个名为 myFunction 的方法:
```
declare class MyLibrary {
myFunction(): void;
}
```
需要注意的是,使用 declare 关键字并不会实际生成任何代码,它只是告诉编译器这些变量、函数或类的类型。因此,如果使用 declare 关键字声明一个变量、函数或类的类型,就必须确保在代码中正确地实现它们。
相关推荐
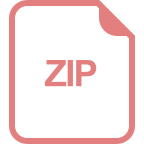
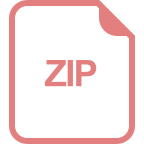
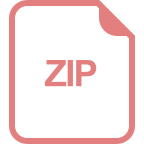












