1、编写一个linux程序,由父进程创建1个子进程,在子进程中显示/home/test.txt文件内容,等待5秒后终止子进程。父进程每秒钟检测子进程是否结束,若子进程未结束打印“child is not finished”,若子进程结束打印“child is finished”后结束
时间: 2024-02-27 19:53:50 浏览: 104
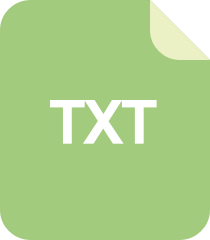
linux 利用进程向文件中写入并输出1~100

好的,这是一个简单的在 Linux 环境下使用 C 语言编写的程序。请确保你已经安装了编译器和相关的开发工具。
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/wait.h>
int main() {
pid_t pid = fork();
if (pid == -1) {
perror("fork failed");
exit(EXIT_FAILURE);
} else if (pid == 0) {
// 子进程
FILE* fp = fopen("/home/test.txt", "r");
if (fp == NULL) {
perror("open file failed");
exit(EXIT_FAILURE);
}
char buffer[1024];
while (fgets(buffer, sizeof(buffer), fp)) {
printf("%s", buffer);
}
fclose(fp);
sleep(5);
exit(EXIT_SUCCESS);
} else {
// 父进程
while (1) {
int status;
int ret = waitpid(pid, &status, WNOHANG);
if (ret == -1) {
perror("waitpid failed");
exit(EXIT_FAILURE);
} else if (ret == 0) {
printf("child is not finished\n");
sleep(1);
} else if (ret == pid) {
printf("child is finished\n");
exit(EXIT_SUCCESS);
}
}
}
}
```
程序中首先使用 `fork()` 创建了一个子进程,并在子进程中打开 `/home/test.txt` 文件,读取其中的内容并输出。然后子进程等待 5 秒后退出。在父进程中,使用 `waitpid()` 检测子进程是否结束。如果子进程还没有结束,父进程会每隔 1 秒钟输出一次提示信息。如果子进程已经结束,父进程则输出相应的提示信息并退出程序。
希望这个程序能够帮助到你!
阅读全文
相关推荐
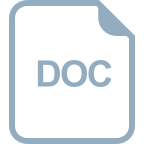
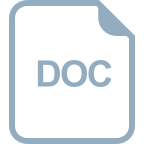
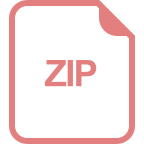
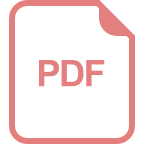
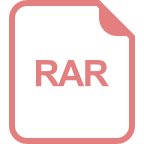
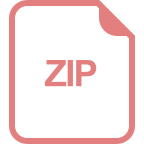
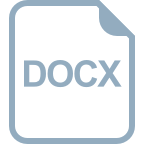
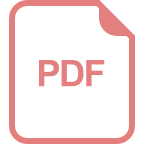
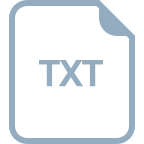
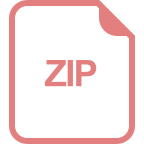
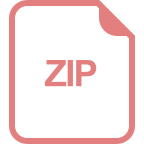
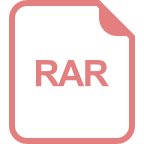
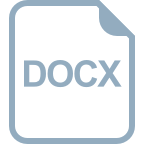
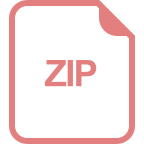
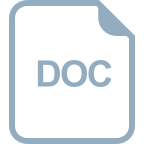
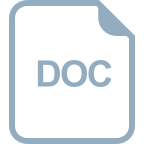
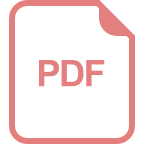
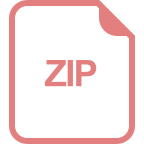