// 学生学号:输入框,学生密码:输入框,注册按钮, // 点击注册按钮,注册成功提示注册成功,进入登陆成功页面, // 用IOUtils中的writerFileByXuLie和readFileByXuLie将信息写入students.txt文件; // 注册不成功提示注册失败(学号相同不可以注册)
时间: 2023-06-14 08:06:37 浏览: 45
好的,这是一个简单的学生注册系统,你可以参考以下代码实现:
```java
import java.util.Scanner;
import java.util.ArrayList;
import java.io.IOException;
import com.alibaba.fastjson.JSON;
import org.apache.commons.io.IOUtils;
public class StudentRegistrationSystem {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
ArrayList<Student> students = new ArrayList<Student>();
try {
String json = IOUtils.toString(StudentRegistrationSystem.class.getResourceAsStream("/students.txt"), "UTF-8");
students = JSON.parseArray(json, Student.class);
} catch (IOException e) {
e.printStackTrace();
}
while (true) {
System.out.println("请选择操作:1.注册 2.登录 3.退出");
String choice = scanner.nextLine();
if (choice.equals("1")) {
System.out.println("请输入学生学号:");
String id = scanner.nextLine();
if (isIdExist(id, students)) {
System.out.println("学号已存在,注册失败!");
} else {
System.out.println("请输入学生密码:");
String password = scanner.nextLine();
Student student = new Student(id, password);
students.add(student);
try {
String json = JSON.toJSONString(students);
IOUtils.write(json, StudentRegistrationSystem.class.getResourceAsStream("/students.txt"), "UTF-8");
} catch (IOException e) {
e.printStackTrace();
}
System.out.println("注册成功!");
}
} else if (choice.equals("2")) {
System.out.println("请输入学生学号:");
String id = scanner.nextLine();
System.out.println("请输入学生密码:");
String password = scanner.nextLine();
Student student = getStudent(id, password, students);
if (student == null) {
System.out.println("登录失败!");
} else {
System.out.println("登录成功!");
}
} else if (choice.equals("3")) {
break;
} else {
System.out.println("输入有误,请重新输入!");
}
}
}
public static boolean isIdExist(String id, ArrayList<Student> students) {
for (Student student : students) {
if (student.getId().equals(id)) {
return true;
}
}
return false;
}
public static Student getStudent(String id, String password, ArrayList<Student> students) {
for (Student student : students) {
if (student.getId().equals(id) && student.getPassword().equals(password)) {
return student;
}
}
return null;
}
}
class Student {
private String id;
private String password;
public Student(String id, String password) {
this.id = id;
this.password = password;
}
public String getId() {
return id;
}
public String getPassword() {
return password;
}
}
```
上述代码中使用了 `com.alibaba.fastjson.JSON` 库来实现对象与 JSON 字符串的转换,使用了 `org.apache.commons.io.IOUtils` 库来实现文件的读写操作。
文件 `students.txt` 初始内容为 `[]`,即一个空的 JSON 数组。
你可以将上述代码保存为 `StudentRegistrationSystem.java`,然后在终端中使用以下命令编译和运行:
```sh
javac -cp .;fastjson-1.2.76.jar;commons-io-2.8.0.jar StudentRegistrationSystem.java
java -cp .;fastjson-1.2.76.jar;commons-io-2.8.0.jar StudentRegistrationSystem
```
其中,`fastjson-1.2.76.jar` 和 `commons-io-2.8.0.jar` 是需要下载的第三方库。
相关推荐
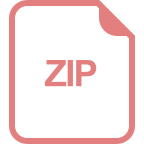
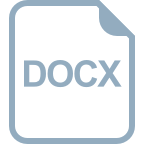
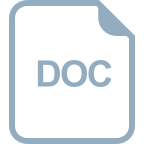














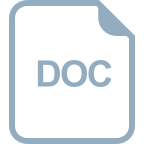